Plug-In API (PlugIn Class)
The following are the definitions and descriptions for the PlugIn class its related functions, properties, and elements.
IMPORTANT: When referencing an Omni Automation plug-in file or bundle, the term “plug-in” is hyphenated. However, when referencing the PlugIn class, no hypens or spaces are used, and the combined words “Plug” and “In” each begin with a capitalized character.
PlugIn Class Functions
find(identifier: String, minimumVersion: Version or null) → (PlugIn or null) • This function is used by scripts to identify a specific installed plug-in.
Find Installed Plug-In
plgn = PlugIn.find("com.omni-automation.og.sort-selected-shapes")
if(!plgn){new Alert("ITEM NOT FOUND", "Plug-In not installed.").show()}
PlugIn Class Properties
all (Array of PlugIn r/o) • This property is used to identify al of the currently installed plug-ins for the host Omni application.
Log Properties of Installed Plug-Ins
PlugIn.all.forEach((plugin, index) => {
if (index != 0){console.log(" ")}
console.log("FILENAME:", plugin.displayName)
console.log("ID:", plugin.identifier)
console.log("VERSION:", plugin.version.versionString)
console.log("AUTHOR:", plugin.author)
console.log("DESC:", plugin.description)
console.log("URL:", plugin.URL.string)
})
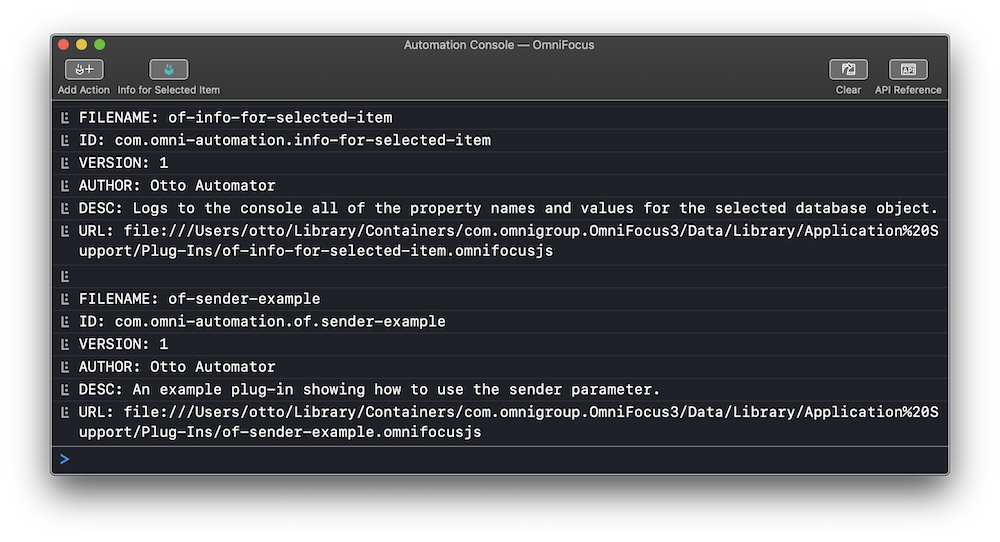
PlugIn Instance Properties
actions (Array of PlugIn.Action r/o) • Returns an array of object references to the actions of the plug-in.
author (String r/o) • Returns the value of the “author” metadata key declared in the plug-in.
description (String r/o) • Returns the value of the “description” metadata key declared in the plug-in.
displayName (String r/o) • Returns the localized, human-readable name for the plug-in. This value is commonly the filename of the plug-in file without the file extension.
handlers (Array of PlugIn.Handler r/o) • Returns an array of object references to the handlers (if any) included in the plug-in.
identifier (String r/o) • The unique identifier of the plug-in.
libraries (Array of PlugIn.Library r/o) • Returns an array of object references to the libraries (if any) included in the plug-in.
version (Version r/o) • Returns a Version instance for the plug-in. TIP: use the versionString property of the Version class to extract the version string for the plug-in.
URL (URL or null r/o) • Returns the original URL from whence this plug-in came, if known. Usually a file URL to a disk item.
PlugIn Instance Functions
A set of functions usually used with bundle plug-ins:
library(identifier: String) → (Object or null) • Looks for a PlugIn.Library in the receiver and returns it if found. Used to locate a specific library.
action(identifier: String) → (PlugIn.Action or null) • Looks for a PlugIn.Action in the receiver and returns it if found. Used to locate a specific action.
handler(identifier: String) → (PlugIn.Handler or null) • Looks for a PlugIn.Handler in the receiver and returns it if found. Used to locate a specific handler.
resourceNamed(name: String) → (URL or null) • Looks for a specific resource in the plug-in Resources folder and returns a URL instance if found.
imageNamed(name: String) → (Image or null) • Looks for a specific image file in the plug-in Resources folder and returns an Image instance if found.
Here’s an example of importing a plug-in resource to an OmniGraffle canvas:
Importing Resource to Canvas
var plugin = PlugIn.find("com.YourCompany.og.PlugInName")
var imageFileName = "ImageName.png"
var url = plugin.resourceNamed(imageFileName)
url.fetch(data => {
var aRect = new Rect(0, 0, 72, 72)
var cnvs = document.windows[0].selection.canvas
var aGraphic = cnvs.addShape('Rectangle', aRect)
aGraphic.strokeThickness = 0
aGraphic.shadowColor = null
aGraphic.fillColor = null
aGraphic.image = addImage(data)
aGraphic.name = imageFileName
var imageSize = aGraphic.image.originalSize
var imgX = imageSize.width
var imgY = imageSize.height
aGraphic.geometry = new Rect(aGraphic.geometry.x, aGraphic.geometry.y, imgX, imgY)
document.windows[0].selection.view.select([aGraphic])
})
PlugIn.Action Class
“Actions” are the script functions that perform the work of their parent plug-in. Single-file plug-ins contain a single action function, while bundle plug-ins may contain one or more actions.
Constructor
new PlugIn.Action(perform: Function) → (PlugIn.Action) • Returns a new PlugIn.Action. Only used within an action JavaScript file embedded within a bundle plug-in, or as a JavaScript function included in a single-file plug-in.
Instance Properties
description (String r/o) • The provided description for the action.
label (String r/o) • Returns the default label (menu item text) to use for interface controls that invoke the action.
longLabel (String r/o) • Returns the label to use for interface controls that invoke the action, when a long amount of space is available.
mediumLabel (String r/o) • Returns the label to use for interface controls that invoke the action, when a medium amount of space is available.
name (String r/o) • Returns the name of the PlugIn.Action. Usually the file name (without file extension) of the action file or the parent plug-in file for a single-action plug-in.
paletteLabel (String r/o) • Returns the label to use for interface controls that show a prototype of the action control, such as on a macOS toolbar configuration sheet.
perform (Function r/o) • The executing function of the action instance.
plugIn (PlugIn r/o) • Returns the parent PlugIn instance that contains this action.
shortLabel (String r/o) • Returns the label to use for interface controls that invoke the action, when a short amount of space is available.
validate (Function or null) • A function to check whether the action is supported, given the current application state, as determined by the arguments passed (typically including the selection). This optional Function may be configured while the Action is being loaded, but after that the Action will be frozen.
Example
The following plug-in can be installed with all Omni applications, and uses both the PlugIn class properties and the PlugIn.Action properties to display an alert containing the metadata information for the host plug-in:
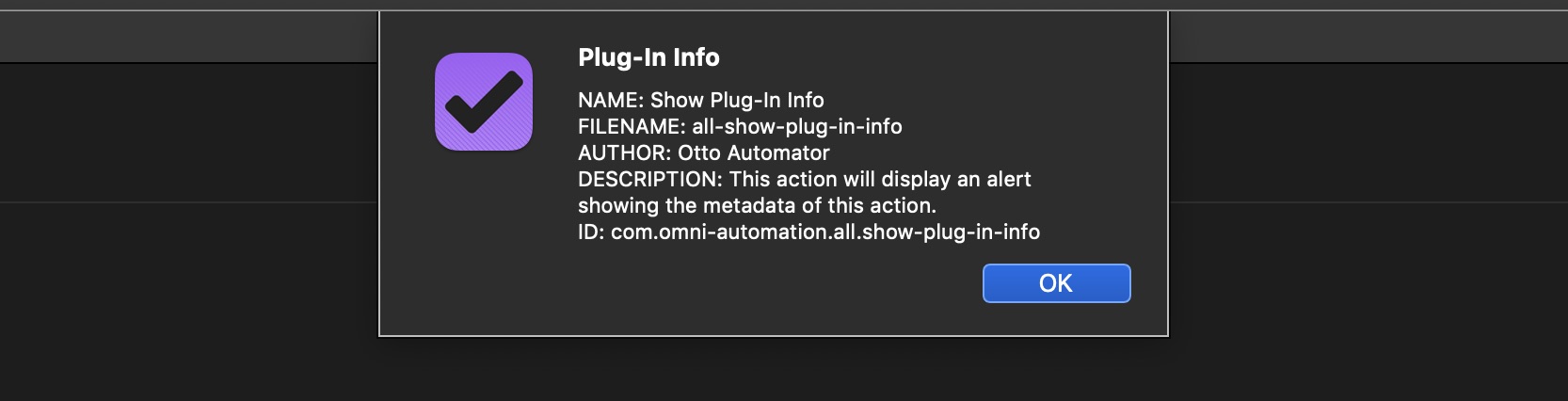
Show Plug-In Info
/*{
"type": "action",
"targets": ["omnifocus",
"omnigraffle", "omniplan", "omnioutliner"], "author": "Otto Automator",
"identifier": "com.omni-automation.all.show-plug-in-info",
"version": "1.1",
"description": "This action will display an alert showing the metadata of this action.",
"label": "Show Plug-In Info",
"shortLabel": "Plug-In Info",
"paletteLabel": "Plug-In Info",
"image": "info.square.fill"
}*/
(() => {
const action = new PlugIn.Action(function(selection, sender){
desc = action.description
auth = action.plugIn.author
filename = action.name
id = action.plugIn.identifier
label = action.label
message = "LABEL: " + label
message += "\n" + "FILENAME: " + filename
message += "\n" + "AUTHOR: " + auth
message += "\n" + "DESCRIPTION: " + desc
message += "\n" + "ID: " + id
new Alert("Plug-In Info", message).show()
});
action.validate = function(selection, sender){
return true
};
return action;
})();
NOTE: See the Single-File Plug-In and Bundle Plug-In sections for more information about plug-ins and actions.
PlugIn.Handler Class
“Handlers” are functions that get triggered automatically when specifically-enabled “events” happen in the host application.
Constructor
new PlugIn.Handler(invoke: Function) → (PlugIn.Handler) • Returns a new PlugIn.Handler. Only used within an handler JavaScript file embedded within a PlugIn.
Instance Properties
invoke (Function r/o) • The Function that will be executed for each handler registered for an event posted by an application object.
name (String r/o) • Returns the name of the PlugIn.Handler.
plugIn (PlugIn r/o) • Returns the PlugIn that contains this object.
willAttach (Function or null) • An optional Function that can be set on PlugIn.Handler as it is being loaded (but not after). This function is passed the application object that post events to trigger the handler. The return value should be a state object that is JSON archivable (or undefined if the handler has no state to maintain across invocations).
willDetach (Function or null) • An optional Function that can be set on PlugIn.Handler as it is being loaded (but not after). Called when a previously attached PlugIn.Handler is being detached from an application object. Any return value or thrown error are ignored.
NOTE: See the Handlers Section for more information.
PlugIn.Library Class
Omni Automation Libraries are specialized plug-ins that provide the means for storing, using, and sharing Omni Automation functions. They make automation tasks easier by dramatically shortening scripts, and they enable the ability to share useful routines with other devices.
Constructor
new PlugIn.Library(version: Version) → (PlugIn.Library) • Returns a new Library. Typically used as a file within a Plug-In bundle, or as a solitary-file plug-in.
Instance Properties
name (String r/o) • Returns the name of the PlugIn.Library. The name is usually the file name of the library file (without file extension).
plugIn (PlugIn r/o) • Returns the PlugIn that contains this object.
version (Version r/o) • Returns the Version of this library, as passed to the constructor. Use the versionString property of the Version class to get the version string.
Here is the basic template for a single-file library plug-in:
Basic Single-File Library
/*{
"type": "library",
"targets": ["omnigraffle","omnioutliner","omnigraffle","omniplan"],
"identifier": "com.omni-automation.all.libraryName",
"version": "1.0"
}*/
(() => {
var myLibrary = new PlugIn.Library(new Version("1.0"));
myLibrary.myFirstFunction = function(passedParameter){
// function code
return myFirstFunctionResult
};
myLibrary.mySecondFunction = function(passedParameter){
// function code
return mySecondFunctionResult
};
return myLibrary;
})();
Here’s example script statements calling functions within a library:
Calling a Library
// locate the library’s parent plug-in by identifier
var plugin = PlugIn.find("com.YourIdentifier.NameOfPlugIn")
if (plugin == null){throw new Error("Library plug-in not installed.")}
// load a library identified by name
var firstLibrary = plugin.library("NameOfFirstLibrary")
// load a library identified by name
var secondLibrary = plugin.library("NameOfSecondLibrary")
// call a library function by name
var callResult = firstLibrary.nameOfLibraryFunction()
// call a library function by name
secondLibrary.nameOfLibraryFunction(callResult)
NOTE: See the Library Section for more information.