Library Plug-Ins
Omni Automation Libraries are specialized plug-ins that provide the means for storing, using, and sharing Omni Automation functions. They make automation tasks easier by dramatically shortening scripts, and they enable the ability to share useful routines with other devices.
Constructor
new PlugIn.Library(version: Version) → (PlugIn.Library) • Returns a new Library. Typically used as a file within a Plug-In bundle, or as a solitary-file plug-in.
Instance Properties
name (String r/o) • Returns the name of the PlugIn.Library. The name is usually the file name of the library file (without file extension).
plugIn (PlugIn r/o) • Returns the PlugIn that contains this object.
version (Version r/o) • Returns the Version of this library, as passed to the constructor. Use the versionString property of the Version class to get the version string.
Here is the basic template for a single-file library plug-in:
Basic Single-File Library
/*{
"type": "library",
"targets": ["omnigraffle","omnioutliner","omnigraffle","omniplan"],
"identifier": "com.omni-automation.all.libraryName",
"version": "1.0"
}*/
(() => {
const myLibrary = new PlugIn.Library(new Version("1.0"));
myLibrary.myFirstFunction = function(passedParameter){
// function code
return myFirstFunctionResult
};
myLibrary.mySecondFunction = function(passedParameter){
// function code
return mySecondFunctionResult
};
myLibrary.info = function(){
var libProps = Object.getOwnPropertyNames(myLibrary)
libProps.forEach((propName, index) => {
if (index != 0){console.log(" ")}
console.log("•", propName)
var item = myLibrary[propName]
if (typeof item === "string"){
console.log(item)
} else if (typeof item === "function"){
console.log(item.toString())
} else if (typeof item === "object"){
if(item instanceof Version){
console.log(item.versionString)
} else if(item instanceof PlugIn){
console.log(item.identifier)
}
}
})
};
return myLibrary;
})();
Here’s a script demonstrating how to identify the libraries contained within a plug-in:
Get Names of Libraries within Plug-In
plugin = PlugIn.find("com.omni-automation.all.date-library")
libs = plugin.libraries
//--> [[object PlugIn.Library: Optional("all-date-library") v1]]
libNames = libs.map(lib => lib.name)
console.log(libNames)
//--> ["all-date-library"]
Here’s an example script statements calling functions within a library:
Calling Library Functions
// locate the library’s parent plug-in by identifier
plugin = PlugIn.find("com.YourIdentifier.NameOfPlugIn")
if (!plugin){throw new Error("Library plug-in not installed.")}
// load a library identified by name
firstLibrary = plugin.library("NameOfFirstLibrary")
// load a library identified by name
secondLibrary = plugin.library("NameOfSecondLibrary")
// call a library function by name
callResult = firstLibrary.nameOfLibraryFunction()
// call a library function by name
secondLibrary.nameOfLibraryFunction(callResult)
Date Library
The following example library from the Date class documentation, is a single-file plug-in containing date comparison functions. This Omni Automation library can be called from within scripts or plug-ins for any of the Omni applications. TAP|CLICK the “Download Library” button to download the library plug-in archive.
The library, once installed, can be loaded and called in scripts using the following statements:
Calling the Date Library
plugin = PlugIn.find("com.omni-automation.all.date-library")
dateLibrary = plugin.library("all-date-library")
// dateLibrary.function-to-call()
dateLibrary.info()
The example library includes a function titled info() that returns the names and values of all of the library’s properties and functions. Adding this function to the libraries you create is a good practice, and provides users with a quick way to learn how to use your library.
Library Info Function
lib.info = function(){
libProps = Object.getOwnPropertyNames(lib)
libProps.forEach((propName, index) => {
if (index != 0){console.log(" ")}
console.log("•", propName)
var item = lib[propName]
if (typeof item === "string"){
console.log(item)
} else if (typeof item === "function"){
console.log(item.toString())
} else if (typeof item === "object"){
if(item instanceof Version){
console.log(item.versionString)
} else if(item instanceof PlugIn){
console.log(item.identifier)
}
}
})
}
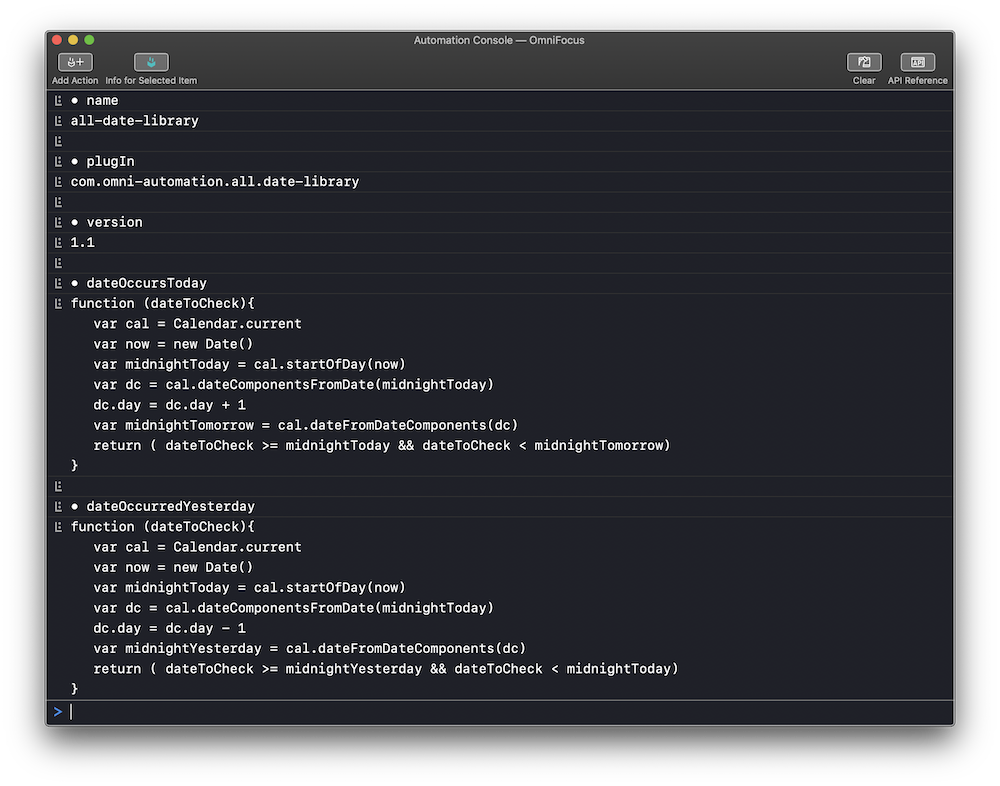
NOTE: In order to have the function code returned correctly when the library info() function is called, align your functions in the library to be flushed to the left side — removing some of preceding tabs:
The Date Comparison Library
/*{
"type": "library",
"targets": ["omnifocus","omnigraffle","omnioutliner","omniplan"],
"identifier": "com.omni-automation.all.date-library",
"author": "Otto Automator",
"version": "1.1",
"description": "A library of date functions."
}*/
(() => {
const lib = new PlugIn.Library(new Version("1.1"));
// returns true if provided date/time occurs today
lib.dateOccursToday = function(dateToCheck){
cal = Calendar.current
now = new Date()
midnightToday = cal.startOfDay(now)
dc = cal.dateComponentsFromDate(midnightToday)
dc.day = dc.day + 1
midnightTomorrow = cal.dateFromDateComponents(dc)
return ( dateToCheck >= midnightToday && dateToCheck < midnightTomorrow)
}
// returns true if provided date/time took place yesterday
lib.dateOccurredYesterday = function(dateToCheck){
cal = Calendar.current
now = new Date()
midnightToday = cal.startOfDay(now)
dc = cal.dateComponentsFromDate(midnightToday)
dc.day = dc.day - 1
midnightYesterday = cal.dateFromDateComponents(dc)
return ( dateToCheck >= midnightYesterday && dateToCheck < midnightToday)
}
// returns true if the provided date/time takes place tomorrow
lib.dateOccurrsTomorrow = function(dateToCheck){
cal = Calendar.current
now = new Date()
midnightToday = cal.startOfDay(now)
dc = cal.dateComponentsFromDate(midnightToday)
dc.day = dc.day + 1
midnightTomorrow = cal.dateFromDateComponents(dc)
dc = cal.dateComponentsFromDate(midnightToday)
dc.day = dc.day + 2
dayAfterTomorrow = cal.dateFromDateComponents(dc)
return (dateToCheck >= midnightTomorrow && dateToCheck < dayAfterTomorrow)
}
// returns true if the provided date/time takes place next week
lib.dateOccursNextWeek = function(dateToCheck){
fmatr = Formatter.Date.withStyle(Formatter.Date.Style.Short)
weekStart = fmatr.dateFromString('next week')
dc = new DateComponents()
dc.day = 7
followingWeek = Calendar.current.dateByAddingDateComponents(weekStart, dc)
return (dateToCheck >= weekStart && dateToCheck < followingWeek)
}
// returns true if the provided date/time takes place this month
lib.dateOccurrsThisMonth = function(dateToCheck){
cal = Calendar.current
currentMonthIndex = cal.dateComponentsFromDate(new Date()).month
targetMonthIndex = cal.dateComponentsFromDate(dateToCheck).month
return (targetMonthIndex === currentMonthIndex)
}
// returns true if the provided date/time takes place next month
lib.dateOccurrsNextMonth = function(dateToCheck){
cal = Calendar.current
dc = cal.dateComponentsFromDate(new Date())
dc.day = 1
dc.month = dc.month + 1
nextMonth = cal.dateFromDateComponents(dc)
nextMonthIndex = cal.dateComponentsFromDate(nextMonth).month
targetMonthIndex = cal.dateComponentsFromDate(dateToCheck).month
return (nextMonthIndex === targetMonthIndex)
}
// returns true if the provided date/time takes place on the provided target date
lib.dateOccursOnTargetDate = function(dateToCheck, targetDate){
cal = Calendar.current
targetDateStart = cal.startOfDay(targetDate)
dc = cal.dateComponentsFromDate(targetDateStart)
dc.day = dc.day + 1
dayAfterTargetDate = cal.dateFromDateComponents(dc)
return ( dateToCheck >= targetDateStart && dateToCheck < dayAfterTargetDate)
}
lib.info = function(){
libProps = Object.getOwnPropertyNames(lib)
libProps.forEach((propName, index) => {
if (index != 0){console.log(" ")}
console.log("•", propName)
var item = lib[propName]
if (typeof item === "string"){
console.log(item)
} else if (typeof item === "function"){
console.log(item.toString())
} else if (typeof item === "object"){
if(item instanceof Version){
console.log(item.versionString)
} else if(item instanceof PlugIn){
console.log(item.identifier)
}
}
})
}
return lib;
})();
Calling the Library
Here’s an example script calling the single-file library from another script or plug-in:
Call Date Comparison Library
plugin = PlugIn.find("com.omni-automation.all.date-library")
dateLibrary = plugin.library("all-date-library")
date = new Date(new Date().setHours(25, 15, 0))
if (dateLibrary.dateOccurrsTomorrow(date)){console.log("Tomorrow!")}
If the library is part of a plug-in bundle, it can be called by simply appending the library file name (no file extension) to the “this” keyword. Here’s the code for a example bundle plug-in action that calls a library in the bundle:
Calling a Bundle Plug-In Library
(() => {
const action = new PlugIn.Action(function(selection, sender){
this.library("all-date-library").info()
})
action.validate = function(selection, sender){
return true
};
return action;
})();
Omni Automation “Lorem Ipsum” Library
Here’s a very useful library for generating text placeholder content: the Omni Automation Lorem Ipsum Library
According to Wikipedia, Lorem ipsum (/ˌlɔː.rəm ˈɪp.səm/ LOR-əm IP-səm) is a dummy or placeholder text commonly used in graphic design, publishing, and web development. Its purpose is to permit a page layout to be designed, independently of the copy that will subsequently populate it, or to demonstrate various fonts of a typeface without meaningful text that could be distracting.
You may find this library useful for creating demonstration text content for Omni applications, such as generating outlines in OmniOutliner, or generating projects in OmniFocus.
List Library Functions
plugin = PlugIn.find("com.omni-automation.all.lorem-ipsum")
loremIpsumLib = plugin.library("all-lorem-ipsum")
loremIpsumLib.listFunctions()
Lorem Ipsum Library functions:
- getRandomInt(min, max)
- randomWord()
- randomWords(numberOfWords, shouldCapitalize)
- randomString(numberOfWords)
- randomSentence()
- randomSentenceWithWordRange(lowValue, highValue)
- randomParagraph()
- randomParagraphWithSentenceRange(lowValue, highValue)
- randomParagraphWithSentenceRangeWordRange(sentenceLowValue, sentenceHighValue, wordLowValue, wordHighValue)
- generateNSentences(sentenceCount, resultIsArray)
- generateNParagraphs(paragraphCount, resultIsArray)
- placeRandomSentenceOnClipboard()
- placeNSentencesOnClipboard(sentenceCount)
- placeNParagraphsOnClipboard(paragraphCount)
The library plug-in code:
Omni Automation “Lorem Ipsum” Library (@)
/*{
"type": "library",
"targets": ["omnigraffle","omnioutliner","omnifocus","omniplan"],
"identifier": "com.omni-automation.all.lorem-ipsum",
"author": "Otto Automator",
"description": "A Omni Automation library for generating lorem ipsum content.",
"version": "1.0"
}*/
(() => {
const loremLib = new PlugIn.Library(new Version("1.0"));
// INSTALLATION: PLACE LIBRARY FILE IN PLUG-INS FOLDER
const loremWords = ["lorem", "ipsum", "dolor", "sit", "amet", "consectetuer", "adipiscing", "elit", "integer", "in", "mi", "a", "mauris", "ornare", "sagittis", "suspendisse", "potenti", "suspendisse", "dapibus", "dignissim", "dolor", "nam", "sapien", "tellus", "tempus", "et", "tempus", "ac", "tincidunt", "in", "arcu", "duis", "dictum", "proin", "magna", "nulla", "pellentesque", "non", "commodo", "et", "iaculis", "sit", "amet", "mi", "mauris", "condimentum", "massa", "ut", "metus", "donec", "viverra", "sapien", "mattis", "rutrum", "tristique", "lacus", "eros", "semper", "tellus", "et", "molestie", "nisi", "sapien", "eu", "massa", "vestibulum", "ante", "ipsum", "primis", "in", "faucibus", "orci", "luctus", "et", "ultrices", "posuere", "cubilia", "curae;", "fusce", "erat", "tortor", "mollis", "ut", "accumsan", "ut", "lacinia", "gravida", "libero", "curabitur", "massa", "felis", "accumsan", "feugiat", "convallis", "sit", "amet", "porta", "vel", "neque", "duis", "et", "ligula", "non", "elit", "ultricies", "rutrum", "suspendisse", "tempor", "quisque", "posuere", "malesuada", "velit", "sed", "pellentesque", "mi", "a", "purus", "integer", "imperdiet", "orci", "a", "eleifend", "mollis", "velit", "nulla", "iaculis", "arcu", "eu", "rutrum", "magna", "quam", "sed", "elit", "nullam", "egestas", "integer", "interdum", "purus", "nec", "mauris", "vestibulum", "ac", "mi", "in", "nunc", "suscipit", "dapibus", "duis", "consectetuer", "ipsum", "et", "pharetra", "sollicitudin", "metus", "turpis", "facilisis", "magna", "vitae", "dictum", "ligula", "nulla", "nec", "mi", "nunc", "ante", "urna", "gravida", "sit", "amet", "congue", "et", "accumsan", "vitae", "magna", "praesent", "luctus", "nullam", "in", "velit", "praesent", "est", "curabitur", "turpis", "class", "aptent", "taciti", "sociosqu", "ad", "litora", "torquent", "per", "conubia", "nostra", "per", "inceptos", "hymenaeos", "cras", "consectetuer", "nibh", "in", "lacinia", "ornare", "turpis", "sem", "tempor", "massa", "sagittis", "feugiat", "mauris", "nibh", "non", "tellus", "phasellus", "mi", "fusce", "enim", "mauris", "ultrices", "turpis", "eu", "adipiscing", "viverra", "justo", "libero", "ullamcorper", "massa", "id", "ultrices", "velit", "est", "quis", "tortor", "quisque", "condimentum", "lacus", "volutpat", "nonummy", "accumsan", "est", "nunc", "imperdiet", "magna", "vulputate", "aliquet", "nisi", "risus", "at", "est", "aliquam", "imperdiet", "gravida", "tortor", "praesent", "interdum", "accumsan", "ante", "vivamus", "est", "ligula", "consequat", "sed", "pulvinar", "eu", "consequat", "vitae", "eros", "nulla", "elit", "nunc", "congue", "eget", "scelerisque", "a", "tempor", "ac", "nisi", "morbi", "facilisis", "pellentesque", "habitant", "morbi", "tristique", "senectus", "et", "netus", "et", "malesuada", "fames", "ac", "turpis", "egestas", "in", "hac", "habitasse", "platea", "dictumst", "suspendisse", "vel", "lorem", "ut", "ligula", "tempor", "consequat", "quisque", "consectetuer", "nisl", "eget", "elit", "proin", "quis", "mauris", "ac", "orci", "accumsan", "suscipit", "sed", "ipsum", "sed", "vel", "libero", "nec", "elit", "feugiat", "blandit", "vestibulum", "purus", "nulla", "accumsan", "et", "volutpat", "at", "pellentesque", "vel", "urna", "suspendisse", "nonummy", "aliquam", "pulvinar", "libero", "donec", "vulputate", "orci", "ornare", "bibendum", "condimentum", "lorem", "elit", "dignissim", "sapien", "ut", "aliquam", "nibh", "augue", "in", "turpis", "phasellus", "ac", "eros", "praesent", "luctus", "lorem", "a", "mollis", "lacinia", "leo", "turpis", "commodo", "sem", "in", "lacinia", "mi", "quam", "et", "quam", "curabitur", "a", "libero", "vel", "tellus", "mattis", "imperdiet", "in", "congue", "neque", "ut", "scelerisque", "bibendum", "libero", "lacus", "ullamcorper", "sapien", "quis", "aliquet", "massa", "velit", "vel", "orci", "fusce", "in", "nulla", "quis", "est", "cursus", "gravida", "in", "nibh", "lorem", "ipsum", "dolor", "sit", "amet", "consectetuer", "adipiscing", "elit", "integer", "fermentum", "pretium", "massa", "morbi", "feugiat", "iaculis", "nunc", "aenean", "aliquam", "pretium", "orci", "cum", "sociis", "natoque", "penatibus", "et", "magnis", "dis", "parturient", "montes", "nascetur", "ridiculus", "mus", "vivamus", "quis", "tellus", "vel", "quam", "varius", "bibendum", "fusce", "est", "metus", "feugiat", "at", "porttitor", "et", "cursus", "quis", "pede", "nam", "ut", "augue", "nulla", "posuere", "phasellus", "at", "dolor", "a", "enim", "cursus", "vestibulum", "duis", "id", "nisi", "duis", "semper", "tellus", "ac", "nulla", "vestibulum", "scelerisque", "lobortis", "dolor", "aenean", "a", "felis", "aliquam", "erat", "volutpat", "donec", "a", "magna", "vitae", "pede", "sagittis", "lacinia", "cras", "vestibulum", "diam", "ut", "arcu", "mauris", "a", "nunc", "duis", "sollicitudin", "erat", "sit", "amet", "turpis", "proin", "at", "libero", "eu", "diam", "lobortis", "fermentum", "nunc", "lorem", "turpis", "imperdiet", "id", "gravida", "eget", "aliquet", "sed", "purus", "ut", "vehicula", "laoreet", "ante", "mauris", "eu", "nunc", "sed", "sit", "amet", "elit", "nec", "ipsum", "aliquam", "egestas", "donec", "non", "nibh", "cras", "sodales", "pretium", "massa", "praesent", "hendrerit", "est", "et", "risus", "vivamus", "eget", "pede", "curabitur", "tristique", "scelerisque", "dui", "nullam", "ullamcorper", "vivamus", "venenatis", "velit", "eget", "enim", "nunc", "eu", "nunc", "eget", "felis", "malesuada", "fermentum", "quisque", "magna", "mauris", "ligula", "felis", "luctus", "a", "aliquet", "nec", "vulputate", "eget", "magna", "quisque", "placerat", "diam", "sed", "arcu", "praesent", "sollicitudin", "aliquam", "non", "sapien", "quisque", "id", "augue", "class", "aptent", "taciti", "sociosqu", "ad", "litora", "torquent", "per", "conubia", "nostra", "per", "inceptos", "hymenaeos", "etiam", "lacus", "lectus", "mollis", "quis", "mattis", "nec", "commodo", "facilisis", "nibh", "sed", "sodales", "sapien", "ac", "ante", "duis", "eget", "lectus", "in", "nibh", "lacinia", "auctor", "fusce", "interdum", "lectus", "non", "dui", "integer", "accumsan", "quisque", "quam", "curabitur", "scelerisque", "imperdiet", "nisl", "suspendisse", "potenti", "nam", "massa", "leo", "iaculis", "sed", "accumsan", "id", "ultrices", "nec", "velit", "suspendisse", "potenti", "mauris", "bibendum", "turpis", "ac", "viverra", "sollicitudin", "metus", "massa", "interdum", "orci", "non", "imperdiet", "orci", "ante", "at", "ipsum", "etiam", "eget", "magna", "mauris", "at", "tortor", "eu", "lectus", "tempor", "tincidunt", "phasellus", "justo", "purus", "pharetra", "ut", "ultricies", "nec", "consequat", "vel", "nisi", "fusce", "vitae", "velit", "at", "libero", "sollicitudin", "sodales", "aenean", "mi", "libero", "ultrices", "id", "suscipit", "vitae", "dapibus", "eu", "metus", "aenean", "vestibulum", "nibh", "ac", "massa", "vivamus", "vestibulum", "libero", "vitae", "purus", "in", "hac", "habitasse", "platea", "dictumst", "curabitur", "blandit", "nunc", "non", "arcu", "ut", "nec", "nibh", "morbi", "quis", "leo", "vel", "magna", "commodo", "rhoncus", "donec", "congue", "leo", "eu", "lacus", "pellentesque", "at", "erat", "id", "mi", "consequat", "congue", "praesent", "a", "nisl", "ut", "diam", "interdum", "molestie", "fusce", "suscipit", "rhoncus", "sem", "donec", "pretium", "aliquam", "molestie", "vivamus", "et", "justo", "at", "augue", "aliquet", "dapibus", "pellentesque", "felis", "morbi", "semper", "in", "venenatis", "imperdiet", "neque", "donec", "auctor", "molestie", "augue", "nulla", "id", "arcu", "sit", "amet", "dui", "lacinia", "convallis", "proin", "tincidunt", "proin", "a", "ante", "nunc", "imperdiet", "augue", "nullam", "sit", "amet", "arcu", "quisque", "laoreet", "viverra", "felis", "lorem", "ipsum", "dolor", "sit", "amet", "consectetuer", "adipiscing", "elit", "in", "hac", "habitasse", "platea", "dictumst", "pellentesque", "habitant", "morbi", "tristique", "senectus", "et", "netus", "et", "malesuada", "fames", "ac", "turpis", "egestas", "class", "aptent", "taciti", "sociosqu", "ad", "litora", "torquent", "per", "conubia", "nostra", "per", "inceptos", "hymenaeos", "nullam", "nibh", "sapien", "volutpat", "ut", "placerat", "quis", "ornare", "at", "lorem", "class", "aptent", "taciti", "sociosqu", "ad", "litora", "torquent", "per", "conubia", "nostra", "per", "inceptos", "hymenaeos", "morbi", "dictum", "massa", "id", "libero", "ut", "neque", "phasellus", "tincidunt", "nibh", "ut", "tincidunt", "lacinia", "lacus", "nulla", "aliquam", "mi", "a", "interdum", "dui", "augue", "non", "pede", "duis", "nunc", "magna", "vulputate", "a", "porta", "at", "tincidunt", "a", "nulla", "praesent", "facilisis", "suspendisse", "sodales", "feugiat", "purus", "cras", "et", "justo", "a", "mauris", "mollis", "imperdiet", "morbi", "erat", "mi", "ultrices", "eget", "aliquam", "elementum", "iaculis", "id", "velit", "in", "scelerisque", "enim", "sit", "amet", "turpis", "sed", "aliquam", "odio", "nonummy", "ullamcorper", "mollis", "lacus", "nibh", "tempor", "dolor", "sit", "amet", "varius", "sem", "neque", "ac", "dui", "nunc", "et", "est", "eu", "massa", "eleifend", "mollis", "mauris", "aliquet", "orci", "quis", "tellus", "ut", "mattis", "praesent", "mollis", "consectetuer", "quam", "nulla", "nulla", "nunc", "accumsan", "nunc", "sit", "amet", "scelerisque", "porttitor", "nibh", "pede", "lacinia", "justo", "tristique", "mattis", "purus", "eros", "non", "velit", "aenean", "sagittis", "commodo", "erat", "aliquam", "id", "lacus", "morbi", "vulputate", "vestibulum", "elit"]
const defaultLowEndWordCharacterCount = 1
const defaultHighEndWordCharacterCount = 10
const defaultLowEndSentenceWordCount = 3
const defaultHighEndSentenceWordCount = 12
const defaultLowEndParagraphSentenceCount = 1
const defaultHighEndParagraphSentenceCount = 6
loremLib.listFunctions = function(){
functionList = [
"info()",
"getRandomInt(min, max)",
"randomWord()",
"randomWords(numberOfWords, shouldCapitalize)",
"randomString(numberOfWords)",
"randomSentence()",
"randomSentenceWithWordRange(lowValue, highValue)",
"randomParagraph()",
"randomParagraphWithSentenceRange(lowValue, highValue)",
"randomParagraphWithSentenceRangeWordRange(sentenceLowValue, sentenceHighValue, wordLowValue, wordHighValue)",
"generateNSentences(sentenceCount, resultIsArray)",
"generateNParagraphs(paragraphCount, resultIsArray)",
"placeRandomSentenceOnClipboard()",
"placeNSentencesOnClipboard(sentenceCount)",
"placeNParagraphsOnClipboard(paragraphCount)"
];
for(func of functionList){
console.log(func);
};
};
loremLib.randomWord = function(){
word = loremWords[Math.floor(Math.random() * loremWords.length)];
return word;
};
loremLib.randomWords = function(numberOfWords, shouldCapitalize){
wordArray = new Array();
for (step = 0; step < numberOfWords; step++) {
word = loremLib.randomWord()
if(shouldCapitalize === true){
word = word[0].toUpperCase() + word.substring(1);
}
wordArray.push(word)
}
return wordArray
};
loremLib.randomString = function(numberOfWords){
if(numberOfWords < 1){ numberOfWords = 1 };
aString = "";
for (step = 0; step < numberOfWords; step++) {
word = loremLib.randomWord();
if(step === 0){
aString = word;
} else {
aString += " " + word;
}
};
return aString;
};
loremLib.getRandomInt = function(min, max) {
min = Math.ceil(min);
max = Math.floor(max);
return Math.floor(Math.random() * (max - min + 1)) + min;
};
loremLib.randomSentence = function(){
numberOfWords = loremLib.getRandomInt(defaultLowEndSentenceWordCount, defaultHighEndSentenceWordCount);
aString = loremLib.randomString(numberOfWords);
aString = aString[0].toUpperCase() + aString.substring(1);
aString = aString + ".";
return aString;
};
loremLib.randomSentenceWithWordRange = function(lowValue, highValue){
numberOfWords = loremLib.getRandomInt(lowValue, highValue);
aString = loremLib.randomString(numberOfWords);
aString = aString[0].toUpperCase() + aString.substring(1);
aString = aString + ".";
return aString;
};
loremLib.randomParagraph = function(){
numberOfSentences = loremLib.getRandomInt(defaultLowEndParagraphSentenceCount, defaultHighEndParagraphSentenceCount);
intArray = Array(numberOfSentences).fill().map((element, index) => index + 0)
sentences = new Array();
for(anInt of intArray){
sentence = loremLib.randomSentence();
sentences.push(sentence);
};
paragraph = sentences.join(" ");
return paragraph
}
loremLib.randomParagraphWithSentenceRange = function(lowValue, highValue){
if(lowValue < 1){lowValue = 1};
numberOfSentences = loremLib.getRandomInt(lowValue, highValue);
intArray = Array(numberOfSentences).fill().map((element, index) => index + 0)
sentences = new Array();
for(anInt of intArray){
sentence = loremLib.randomSentence();
sentences.push(sentence);
};
paragraph = sentences.join(" ");
return paragraph;
}
loremLib.randomParagraphWithSentenceRangeWordRange = function(sentenceLowValue, sentenceHighValue, wordLowValue, wordHighValue){
if(sentenceLowValue < 1){sentenceLowValue = 1};
if(wordLowValue < 1){wordLowValue = 1};
numberOfSentences = loremLib.getRandomInt(sentenceLowValue, sentenceHighValue);
intArray = Array(numberOfSentences).fill().map((element, index) => index + 0)
sentences = new Array();
for(anInt of intArray){
sentence = loremLib.randomSentenceWithWordRange(wordLowValue, wordHighValue);
sentences.push(sentence);
};
paragraph = sentences.join(" ");
return paragraph;
}
loremLib.generateNSentences = function(sentenceCount, resultIsArray){
if(sentenceCount < 1){sentenceCount = 1};
intArray = Array(sentenceCount).fill().map((element, index) => index + 0);
sentenceArray = new Array();
for(anInt of intArray){
sentence = loremLib.randomSentence();
sentenceArray.push(sentence);
};
if(resultIsArray){
return sentenceArray
} else {
textBlock = sentenceArray.join(" ");
return textBlock;
};
}
loremLib.generateNParagraphs = function(paragraphCount, resultIsArray){
if(paragraphCount < 1){paragraphCount = 1};
intArray = Array(paragraphCount).fill().map((element, index) => index + 0);
paragraphArray = new Array();
for(anInt of intArray){
paragraph = loremLib.randomParagraph();
paragraphArray.push(paragraph);
};
if(resultIsArray){
return paragraphArray
} else {
textBlock = paragraphArray.join("\n");
return textBlock;
};
}
loremLib.placeRandomSentenceOnClipboard = function(){
textBlock = loremLib.randomSentence();
Pasteboard.general.string = textBlock;
}
loremLib.placeNSentencesOnClipboard = function(sentenceCount){
textBlock = loremLib.generateNSentences(sentenceCount, false);
Pasteboard.general.string = textBlock;
}
loremLib.placeNParagraphsOnClipboard = function(paragraphCount){
textBlock = loremLib.generateNParagraphs(paragraphCount, false);
Pasteboard.general.string = textBlock;
}
loremLib.info = function(){
var libProps = Object.getOwnPropertyNames(loremLib)
libProps.forEach((propName, index) => {
if (index != 0){console.log(" ")}
console.log("•", propName)
var item = loremLib[propName]
if (typeof item === "string"){
console.log(item)
} else if (typeof item === "function"){
console.log(item.toString())
} else if (typeof item === "object"){
if(item instanceof Version){
console.log(item.versionString)
} else if(item instanceof PlugIn){
console.log(item.identifier)
}
}
})
};
return loremLib;
})();
The Outline Builder Plug-In (@)
The Outline Builder Plug-in uses the Omni Automation “Lorem Ipsum” Library (@) to construct outlines in OmniOutliner, displaying “lorem ipsum” content. Perfect for exploring features and providing demonstrations. The plug-in offers controls for: setting the number of rows; adding level 2, 3, and 4 content; and an option to automatically generate notes of random length for all created rows in the outline.
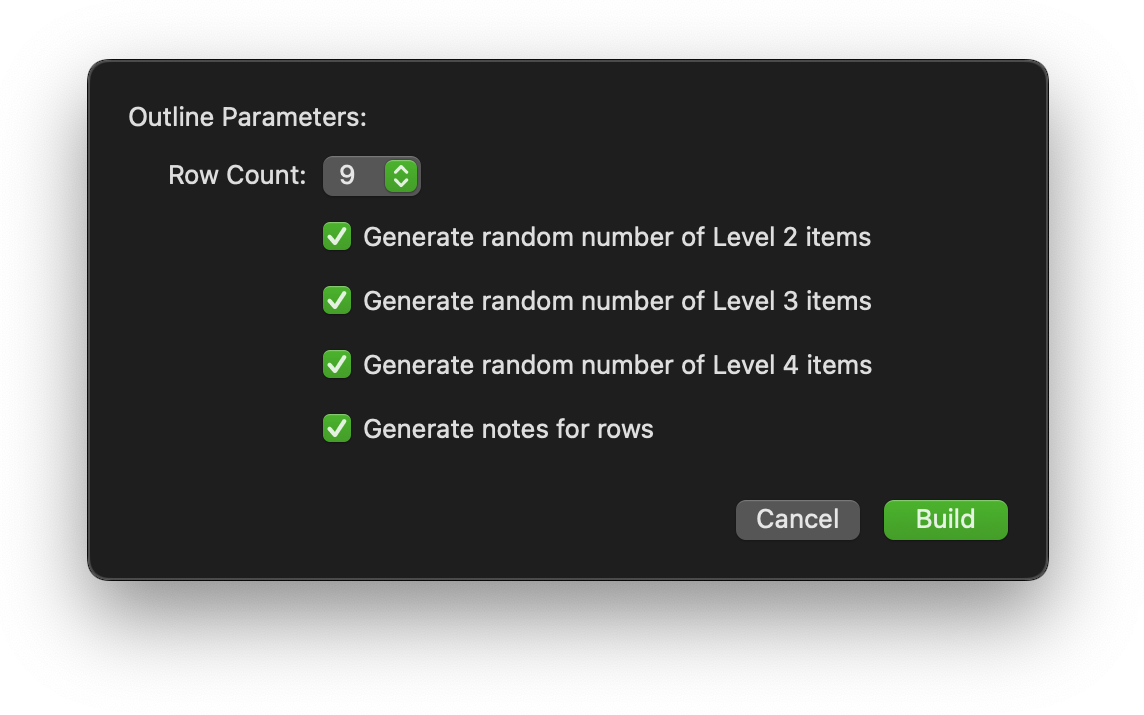
The Outline Builder plug-in is available to install with this link: