Document and Data Export
OmniFocus supports the export of documents and data to files of various formats. This section examines how to create File Wrappers in preparation for the export of documents and content.
Documentation regarding the other classes involved in the saving and export of OmniFocus documents can be found in FileTypes and FileSavers.
FileWrappers
If you think of an outline as a set of data, then it’s easy to understand that an outline’s data can be packaged in a variety of ways. Each “data package” has a set of parameters that determine how the outline data is stored or presented.
For example, one package may store the data as tabbed data, while another may store the outline data in XML format. Each of the supported file packaging formats has its own set of parameters. In terms of Omni Automation, these file packages are referred to as instances of the FileWrapper class.
Each instance of the FileWrapper class has a unique type identifier that identifies that wrapper. To get a list of the export types supported by OmniOmniFocus, write a simple script to access the value of the writableTypes property of the Document class. The result will be an array of the identifiers for the FileWrapper types supported in OmniFocus.
For documentation of both readable and writable file types in OmniFocus, visit the FileType shared topic.
The following example script generates an array of the identifiers that comprise the value for the writableTypes property:
OmniFocus FileWrapper Writable Types
types = document.writableTypes.map(type => {
return "\"" + type + "\""
})
types = "[" + types.join(",\n") + "]"
console.log(types)
//--> ["com.omnigroup.omnifocus.filetype.ofocus",
"com.omnigroup.omnifocus2.export-filetype.plain-text",
"com.omnigroup.omnifocus2.export-filetype.html",
"com.omnigroup.omnifocus2.export-filetype.comma-separated-values",
"com.omnigroup.omnifocus2.export-filetype.comma-separated-values-unicode",
"com.omnigroup.omnifocus.filetype.ofocus-backup"]
A writable type identifier is used with the makeFileWrapper() function when creating a new file wrapper instance:
New FileWrapper Instance
(async () => {
try {
fileTypeID = "com.omnigroup.omnifocus.filetype.ofocus"
baseName = "OF Backup"
wrapper = await document.makeFileWrapper(baseName, fileTypeID)
// WRAPPER PROCESSING STATEMENTS
}
catch(err){
new Alert(err.name, err.message).show()
}
})();
Instance Properties
Each FileWrapper instance has a set of supported properties, most of which have values that are read-only, with the exception of the preferredFilename property whose value can be set in a script.
children (Array of FileWrapper r/o) • Returns an Array of child FileWrappers, if this represents a directory. Otherwise, an empty array is returned.
contents (Data r/o) • Returns the regular file contents of the wrapper, if this represents a regular file. Otherwise, null is returned.
destination (URL or null r/o) • Returns the destination if this represents a symbolic link. Otherwise, null is returned.
filename (String or null) • Returns the actual file name that was last read for this file wrapper. Depending on the names of other sibling wrappers, this may not be what file name will be written.
preferredFilename (String or null) • Returns the preferred file name that should be used when writing the file wrapper if no other file in the same parent directory wrapper is in use.
type (FileWrapper.Type r/o) • Returns the type of this FileWrapper
The value of the contents property is a representation of the outline data, which can be manipulated using class and instance functions from the Data class:
Base 64 Encode New File Wrapper Instance
(async () => {
try {
fileTypeID = "com.omnigroup.omnifocus.filetype.ofocus"
baseName = "OF Backup"
wrapper = await document.makeFileWrapper(baseName, fileTypeID)
encodedData = wrapper.contents.toBase64()
console.log(encodedData)
}
catch(err){
new Alert(err.name, err.message).show()
}
})();
Class Functions
The class functions for the FileWrapper class:
withContents(name:String or nil, contents:Data or nil) → (FileWrapper) • This method is used to create a folder containing other file exports.
Instance Functions
The instance functions for the FileWrapper class:
filenameForChild(child:FileWrapper) → (String or nil) • Returns the unique file name that will be used for the given child FileWrapper, or null if this file wrapper is not a child of the receiver.
FileWrapper.Type
The class properties of a FileWrapper.Type:
Directory (FileWrapper.Type r/o) • A FileWrapper that represents a directory with zero or more child wrappers.
File (FileWrapper.Type r/o) • A FileWrapper that represents a regular file with data contents.
Link (FileWrapper.Type r/o) • A FileWrapper that represents a symbolic link to another location.
Export Types
Here are the various export formats with their corresponding file type identifiers:
- OmniFocus Document (.ofocus)
- This is an ordinary OmniFocus document, like the one that you use as your database. If you open such a file in OmniFocus, it appears in its own window and you can work with it normally, but settings specific to your database (such as custom perspectives and View options) don’t come along with it.
- com.omnigroup.omnifocus.filetype.ofocus
- Plain Text (.txt)
- This is a lightweight plain-text representation of your data, able to be opened in the text editor of your choice. OmniFocus’s plain text export is inspired by TaskPaper, the light to-do application from Hog Bay Software. As such the output should be roughly compatible and able to be imported to TaskPaper with a minimum of fuss.
- com.omnigroup.omnifocus2.export-filetype.plain-text
- Simple HTML (.html)
- This is a single-file HTML representation of your data; the stylesheet and even the icons are embedded in the HTML. If you are proficient with CSS, you should be able to restyle the result however you like.
- com.omnigroup.omnifocus2.export-filetype.html
- Comma Separated Values (.csv)
- CSV is a common syntax for applications old and new on all platforms: all of your data in a plain text file with its columns separated by commas. Once you have your data in CSV format, it’s easy to run scripts on it, convert it to some other format, or open it in applications that understand it (like OmniPlan). If you’re having trouble persuading other applications to read the non-ASCII characters in your CSV file, such as accented letters or non-Roman characters, try exporting with the UTF–16 CSV option.
- omnigroup.omnifocus2.export-filetype.comma-separated-values
- omnigroup.omnifocus2.export-filetype.comma-separated-values-unicode
- Backup Document (.ofocus-backup)
- This export option creates a file in a format (.ofocus-backup) that is essentially the same as the standard OmniFocus database format, with one key difference.
- Unlike a standard OmniFocus database file, when you open a backup in OmniFocus, the option to Revert to This Backup appears in a notice bar beneath the toolbar. Click this button to replace your local default database with the database contained in the backup.
- omnigroup.omnifocus.filetype.ofocus-backup
Saving/Exporting a Specific Document Type
The following Omni Automation script demonstrates how to save a backup OmniFocus document to file. Similar procedures are used by all Omni applications to export data and documents to file.
Detailed documentation regarding the FileType class, and its use to generate application-specific FileWrappers, can be found in the FileType topic.
Save OmniFocus Backup Document
(async () => {
try {
fmtr = Formatter.Date.withFormat('eee-MMM-dd-yyyy')
defaultName = "OF Backup " + fmtr.stringFromDate(new Date())
fileTypeID = 'com.omnigroup.omnifocus.filetype.ofocus-backup'
wrapper = await document.makeFileWrapper(defaultName, fileTypeID)
filesaver = new FileSaver()
filetype = new FileType(fileTypeID)
filesaver.types = [filetype]
urlObj = await filesaver.show(wrapper)
}
catch(err){
new Alert(err.name, err.message).show()
}
})();
AirDrop OmniFocus Database Backup
Here is an example script that use the SharePanel class to AirDrop a backup copy of the OmniFocus document:
AirDrop Backup of OmniFocus Document
(async () => {
try {
fileTypeID = 'com.omnigroup.omnifocus.filetype.ofocus-backup'
defaultName = "OmniFocus DB Backup"
wrapper = await document.makeFileWrapper(defaultName, fileTypeID)
new SharePanel([wrapper]).show()
}
catch(err){
new Alert(err.name, err.message).show()
}
})();
When the script is run, the share sheet appears from which you can select the option to AirDrop the exported file:
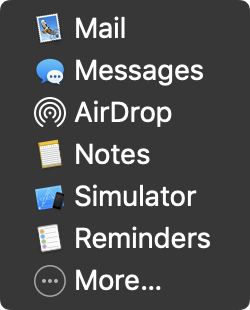
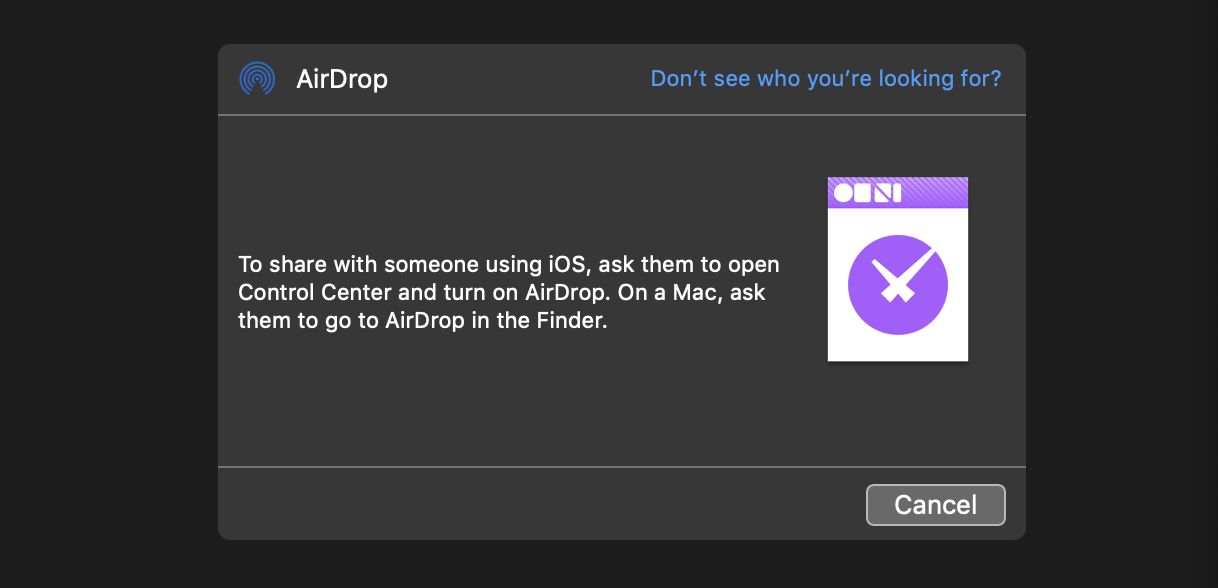
Share Task Attachments
Here’s a plug-in variation of the previous script example, that displays share sheet for the attachments of the selected task:
Share Task Attachements
/*{
"author": "Otto Automator",
"targets": ["omnifocus"],
"type": "action",
"identifier": "com.omni-automation.of.share-attachments",
"version": "1.0",
"description": "Displays a share sheet for sharing the task attachments.",
"label": "Share Attachments",
"mediumLabel": "Share Attachments",
"paletteLabel": "Share Attachments",
"image": "square.and.arrow.up.on.square"
}*/
(() => {
const action = new PlugIn.Action(function(selection, sender){
task = selection.tasks[0]
new SharePanel(task.attachments).show()
});
action.validate = function(selection, sender){
return (
selection.tasks.length === 1 &&
selection.tasks[0].attachments.length !== 0
)
};
return action;
})();