FileSaver
An instance of the FileSaver class enables the user to save one or more instances of the FileWrapper class to disk using the system-provided file picking interface.
Properties
Here are the properties of the FileSaver class.
message (String) • A message to display describing what file is being saved. This is currently only supported on macOS.
nameLabel (String) • The label shown next to the user-editable file name field. This is currently only supported on macOS.
prompt (String) • The prompt shown on the the save button. This is currently only supported on macOS.
types (Array of TypeIdentifier) • The file types that will be allowed. If null, all file types will be allowed. Defaults to null.
Constructors
The mechanism for creating a new instance of the FileSaver class.
new FileSaver() → (FileSaver) • Returns a new file saver with default settings.
Instance Functions
The functions that can be executed on an instance of the FileSaver class.
show(fileWrapper:FileWrapper) → (Promise) • Presents the system file saving interface to the user, allowing them to choose a location and file name to save the file wrapper. The returned Promise will yield the chosen URL on success. If the user cancels chosing, the Promise will be rejected.
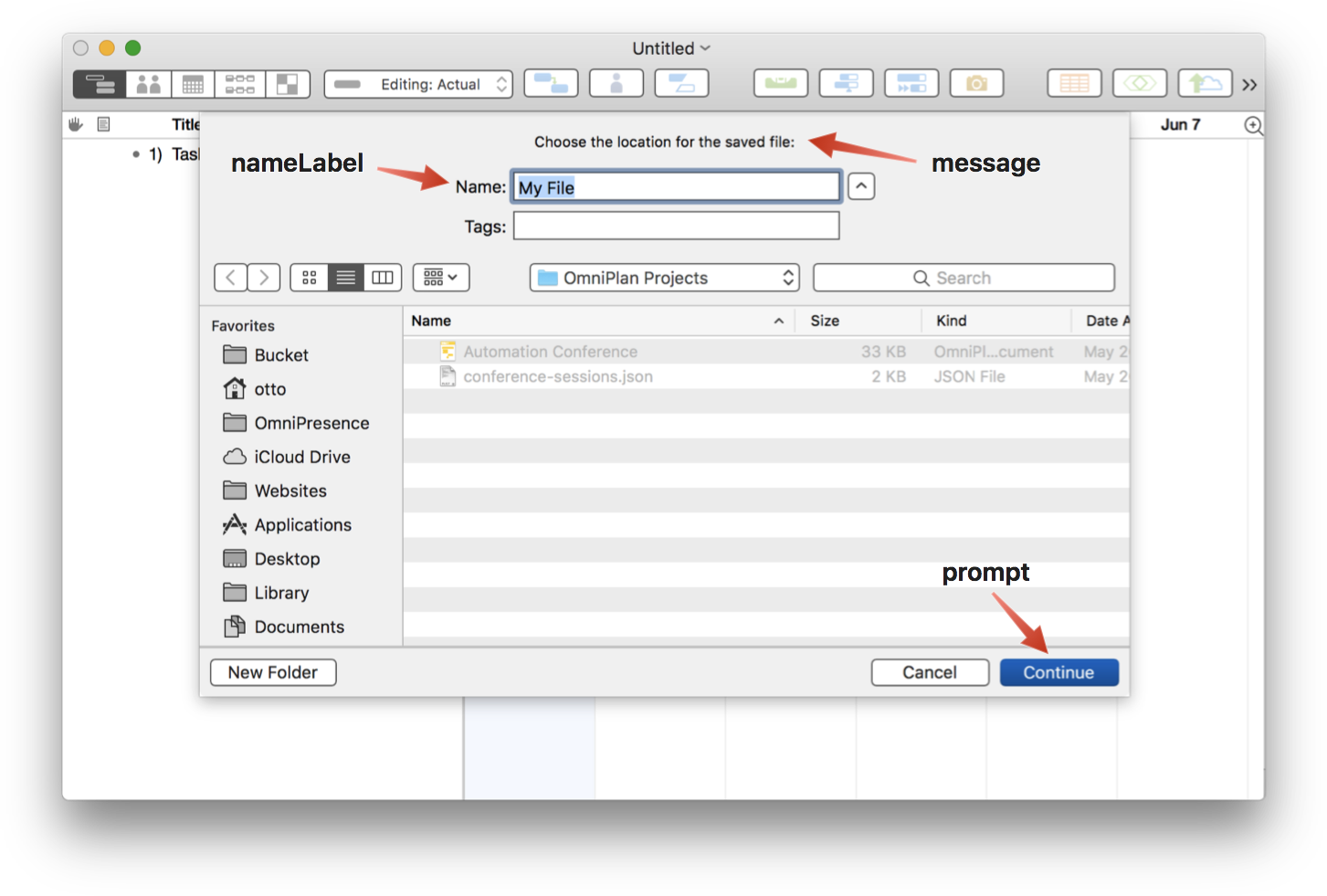
Saving/Exporting a Default Document Type
The following Omni Automation script demonstrates how to save an instance of the default document type to file. Similar procedures are used by all Omni applications to export data and documents to file.
Default Document Export
(async () => {
try {
wrapper = await document.makeFileWrapper("Default Name")
filesaver = new FileSaver()
urlObj = await filesaver.show(wrapper)
new Alert("FILE URL", urlObj.string).show()
}
catch(err){
if(!err.causedByUserCancelling){
new Alert(err.name, err.message).show()
}
}
})();
Saving/Exporting a Specific Document Type
The following Omni Automation script demonstrates how to save an OmniPlan document to file. Similar procedures are used by all Omni applications to export data and documents to file.
Detailed documentation regarding the TypeIdentifer class, and its use to generate application-specific FileWrappers, can be found in the TypeIdentifer topic.
Save OmniPlan Document
(async () => {
try {
// CREATE FILE WRAPPER
fileTypeID = 'com.omnigroup.omniplan2.planfile'
dc = Calendar.current.dateComponentsFromDate(new Date())
dateSlug = dc.month + "-" + dc.day + "-" + dc.year
defaultName = "Backup" + "-" + dateSlug //--> 8-23-21
wrapper = await document.makeFileWrapper(defaultName, fileTypeID)
// CREATE AND DISPLAY FILESAVER
filesaver = new FileSaver()
filesaver.message = "Choose the location for the saved file:" // macOS
filesaver.nameLabel = "Name:" // macOS
filesaver.prompt = "Continue" // macOS
filetype = new FileType(fileTypeID)
filesaver.types = [filetype]
urlObj = await filesaver.show(wrapper)
console.log(urlObj.string)
}
catch(err){
if(!err.causedByUserCancelling){
new Alert(err.name, err.message).show()
}
}
})();
Multiple Exports
In an application such as OmniGraffle, the need to export multiple instances of a canvas in various formats, in not an unusal occurence. The FileWrapper class includes a function for creating a folder wrapper that contains other file wrappers as its contents. The folder wrapper is passed to the FileSaver instance and when executed, a new directory containing the exported files is created on disk.
withChildren(name:String or null, children:Array of FileWrapper) → (FileWrapper r/o) • Returns a new file wrapper that represents a directory with the given child file wrappers. Each child file wrapper must have a unique name specified.
In the following script example, the current OmniGraffle canvas is exported as both an image in PNG format, and as a PDF file, both placed within a created directory.
Multiple Canvas Export to Folder
(async () => {
try {
cnvsName = document.windows[0].selection.canvas.name
PNGwrapper = await document.makeFileWrapper(cnvsName, "public.png")
PDFwrapper = await document.makeFileWrapper(cnvsName, "com.adobe.pdf")
fileWrappers = [PNGwrapper, PDFwrapper]
// Create and Show File Saver
filesaver = new FileSaver()
folderType = new FileType("public.folder")
filesaver.types = [folderType, FileType.png, FileType.pdf]
fldrwrapper = FileWrapper.withChildren("Export Folder", fileWrappers)
urlObj = await filesaver.show(fldrwrapper)
console.log(urlObj.string)
}
catch(err){
if(!err.causedByUserCancelling){
new Alert(err.name, err.message).show()
}
}
})();
Export Layers
Here’s another example plug-in that exports the layers of the current canvas as either individual PNG files, or as images composited from the bottom to the topmost layer.
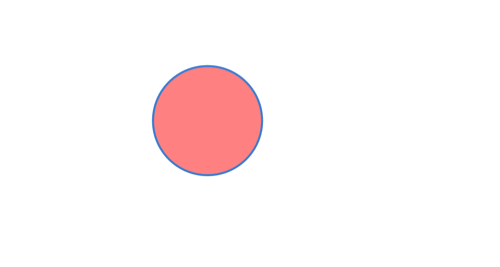
Export Layers to Images
/*{
"type": "action",
"targets": ["omnigraffle"],
"author": "Otto Automator",
"identifier": "com.omni-automation.og.export-layers-current-vanvas",
"version": "1.1",
"description": "Exports the layers of the current canvas as either individual PNG files, or as images composited from the bottom to the topmost layer.",
"label": "Export Current Canvas Layers",
"shortLabel": "Export Layers",
"paletteLabel": "Export Layers",
"image": "square.3.layers.3d.top.filled"
}*/
(() => {
const action = new PlugIn.Action(async function(selection, sender){
try {
exportOptions = ["Individual Images","Composited Images"]
exportOptionsMenu = new Form.Field.Option(
"exportOption",
null,
[0,1],
exportOptions,
0
)
inputForm = new Form()
inputForm.addField(exportOptionsMenu)
formPrompt = "Choose the export style:"
buttonTitle = "Continue"
formObject = await inputForm.show(formPrompt,buttonTitle)
exportOptionIndex = formObject.values["exportOption"]
cnvs = document.windows[0].selection.canvas
cnvs.layers.forEach(layer => layer.visible = false)
wrappers = new Array()
layerSet = cnvs.layers.slice().reverse()
counter = 0
for (aLayer of layerSet){
aLayer.visible = true
exportName = encodeURIComponent(counter + "-" + aLayer.name)
fileTypeID = "public.png"
wrapper = await document.makeFileWrapper(
exportName, fileTypeID) wrappers.push(wrapper)
if (exportOptionIndex === 0){aLayer.visible = false}
counter = counter + 1
}
cnvs.layers.forEach(layer => layer.visible = true)
filesaver = new FileSaver()
folderType = new FileType("public.folder")
filesaver.types = [folderType, FileType.png, FileType.pdf]
docName = document.name.replace(/\.[^/.]+$/, "")
fldrwrapper = FileWrapper.withChildren(
`${docName} Export Folder`, wrappers) urlObj = await filesaver.show(fldrwrapper)
urlObj.open()
}
catch(err){
if(!err.causedByUserCancelling){
new Alert(err.name, err.message).show()
}
}
});
action.validate = function(selection, sender){
return true
};
return action;
})();
Exporting Text to File
Here’s an example OmniGraffle action plug-in that will save the text contents of the selected shape to a file on disk:
Save Shape Text to File
/*{
"type": "action",
"targets": ["omnigraffle"],
"author": "Otto Automator",
"identifier": "com.omni-automation.og.shape-text-to-file",
"version": "1.2",
"description": "This action will save the text contents of the selected shape to a file on disk.",
"label": "Save Shape Text to File",
"shortLabel": "Text to File",
"paletteLabel": "Text to File",
"image": "note.text"
}*/
(() => {
const action = new PlugIn.Action(async function(selection, sender){
shape = selection.solids[0]
shapeText = shape.text
data = Data.fromString(shapeText)
wrapper = FileWrapper.withContents('Shape Text.txt', data)
filesaver = new FileSaver()
urlObj = await filesaver.show(wrapper)
console.log(urlObj.string)
urlObj.open()
});
action.validate = function(selection, sender){
if (selection.solids.length === 1){
return (selection.solids[0].text !== "")
}
return false
};
return action;
})();
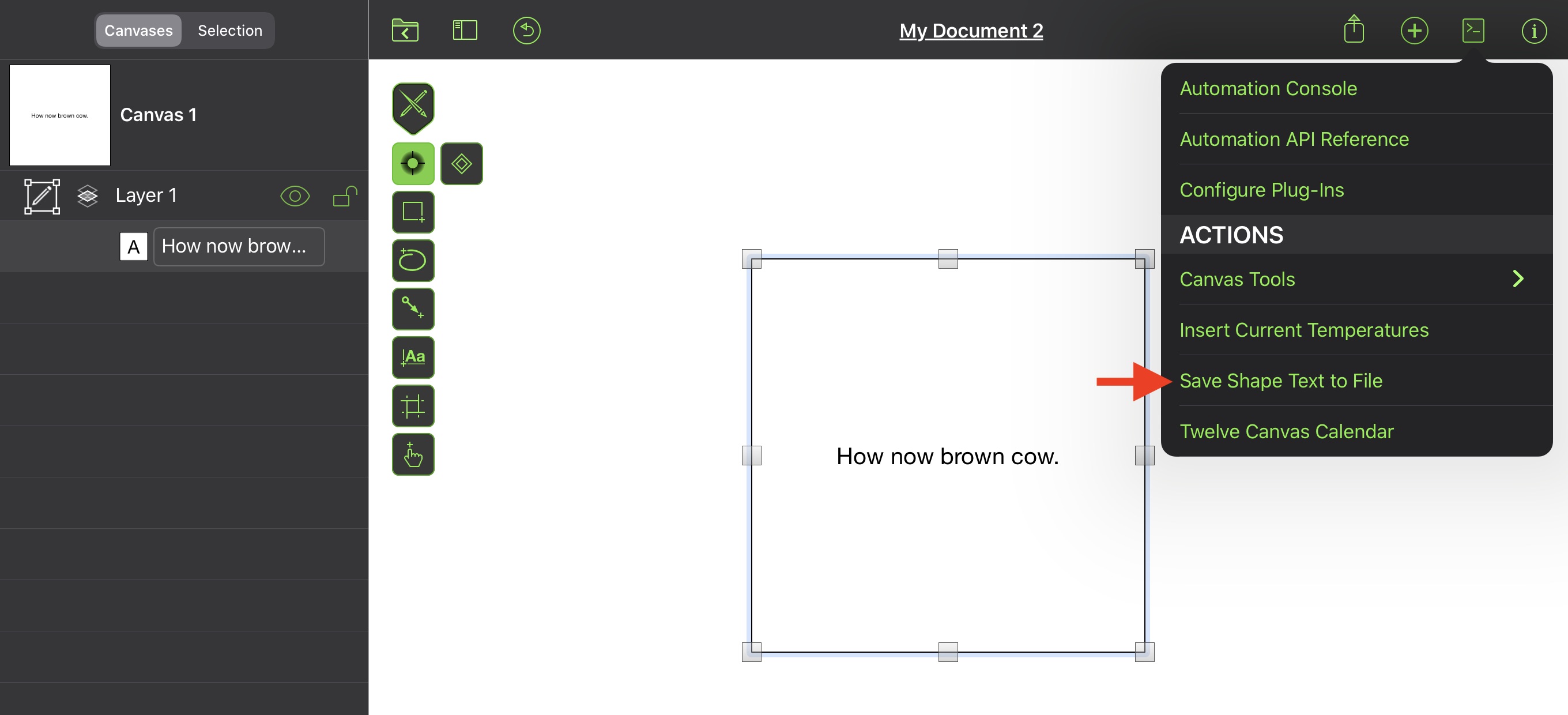
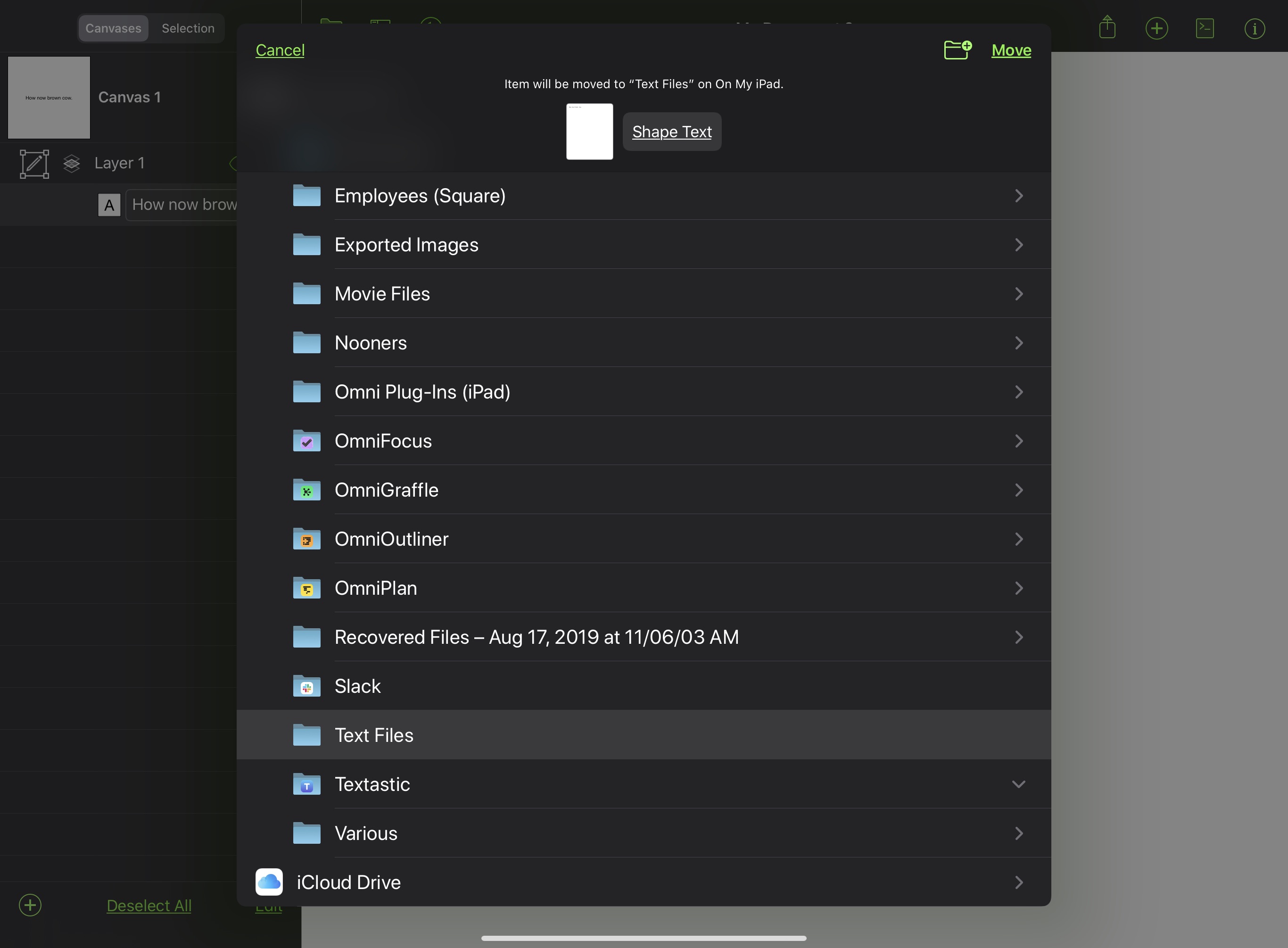
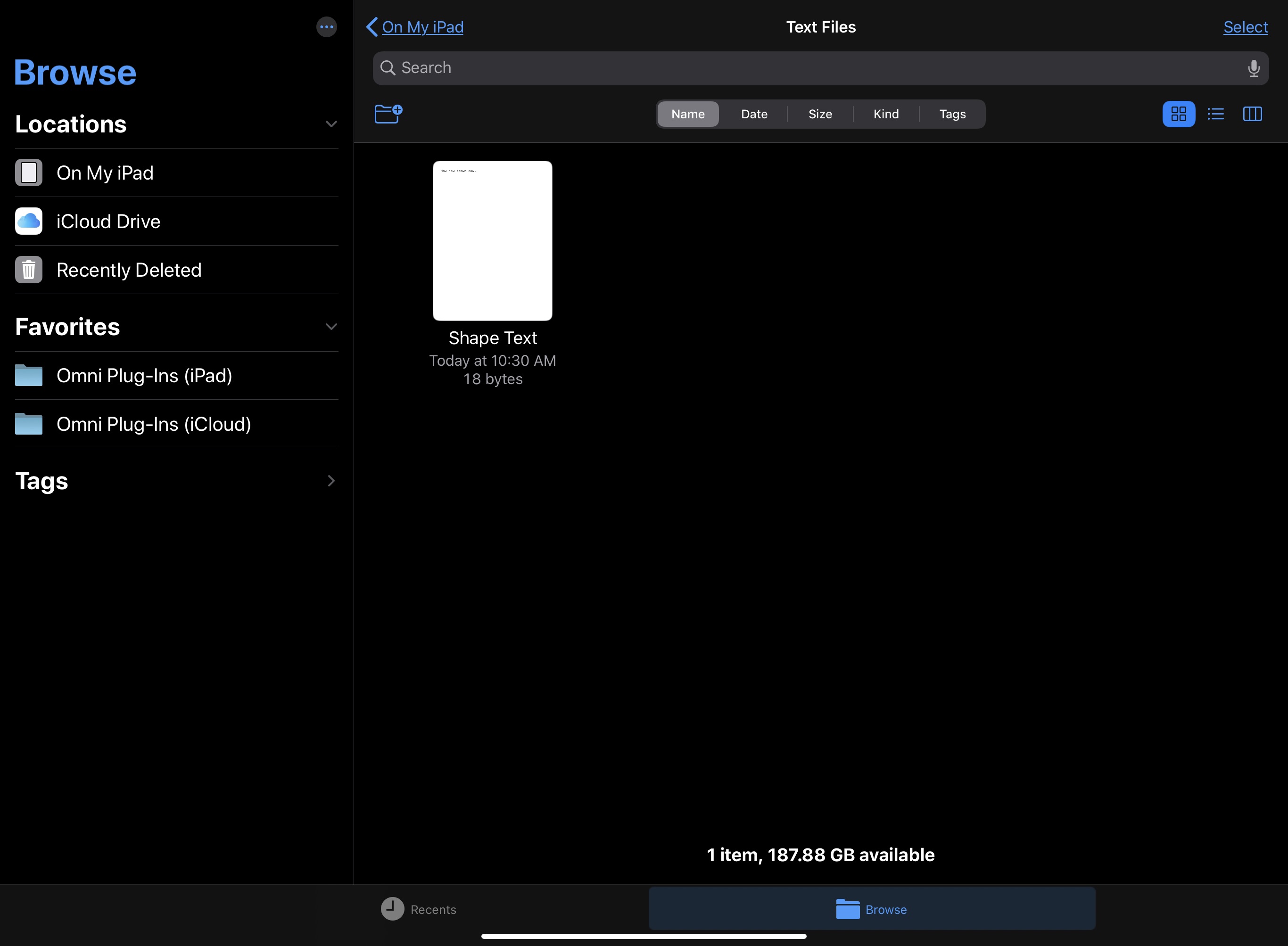