Passing Data from the Action
One of the more powerful features of Shortcuts is the ability for its actions to pass data to other actions in their parent workflow. This passed data is can be in a variety of formats, such as a string (text), an array (list), a number, or a dictionary (record). This section demonstrates how to pass data from the “Omni Automation Script” action to the parent shortcut.
Script Template for Displaying Forms or Errors
The following script template can be used with the “Omni Automation Script” action when it necessary for the script to display form content or error messages. Use this as a starting point for your scripts.
1-12 Asynchronous Function • The script code is placed within a self-invoking asynchronous wrapper that enables the display of dialogs, forms, and alerts by preceding display commands with the await keyword.
2-5 Error Handler • Code is placed within an error handler to trap any issues that arise during the execution of the code.
4 Script Result • The resulting value (string, number, array, or dictionary) is passed to the next action in the parent shortcut by returning the value to be passed.
6-12 Error Handler • This section “catches” any thrown error, displays it to the user, and then throws another error to trigger the halting of the shortcut.
Script Template for Displaying Forms or Errors
(async () => {
try {
// SCRIPT STATEMENTS GO HERE
return resultingValue
}
catch(err){
if(!err.message.includes("cancelled")){
await new Alert(err.name, err.message).show()
}
throw `${err.name}\n${err.message}`
}
})()
Return the Title of the Chosen Form Menu Item
1 “Open App” action • Although the code for this example works with any of the Omni apps, it is shown using OmniFocus.
2 Omni Automation Script action • Although the code for this example works with any of the Omni apps, it is shown using OmniFocus.
3 Asynchronous Script Function • Since Omni Automation forms incorporate the use of JavaScript Promises, an asynchronous script function is used to present a simple options menu to the user.
4 Passed Result • The title of the chosen menu item is passed to the next action by using the return keyword in the function. IMPORTANT: if the script is not wrapped in a function, and a return statement is not used, then the result of the last statement of the script is passed on as the result of the action.
(⬇ see below ) A shortcut containing an instance of the Omni Automation Script action that presents a form and returns the chosen result:
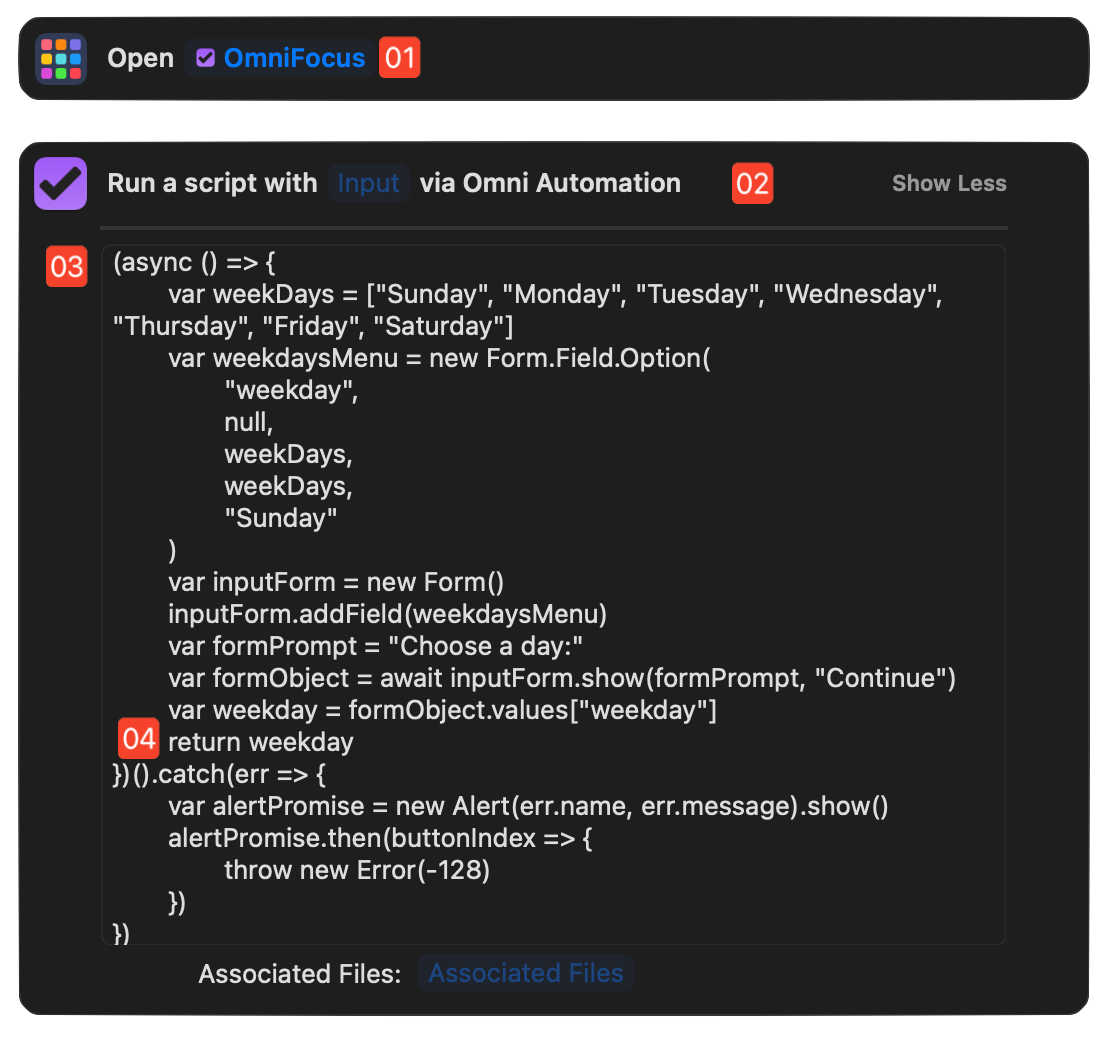
Return Title of Chosen Menu Item
(async () => {
try {
var weekDays = ["Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"]
var weekdaysMenu = new Form.Field.Option(
"weekday",
null,
weekDays,
weekDays,
"Sunday"
)
var inputForm = new Form()
inputForm.addField(weekdaysMenu)
var formPrompt = "Choose a day:"
var buttonTitle = "Continue"
var formObject = await inputForm.show(formPrompt, buttonTitle)
var weekday = formObject.values["weekday"]
return weekday
}
catch(err){
if(!err.message.includes("cancelled")){
await new Alert(err.name, err.message).show()
}
throw `${err.name}\n${err.message}`
}
})()
OmniFocus • Add Task to Selected Project
IMPORTANT: OmniFocus 4.5 introduces new Shortcuts actions for generating Object References from passed IDs and URLS. It is no longer necessary to pass a JSON object. Documentation here.
When used in an Omni Automation script targeting the OmniFocus application, the selection parameter represents the Selection object generated by the script statement: document.windows[0].selection
In OmniFocus, the types that can be appended to the selection parameter are: tasks, projects, folders, tags, database, window, databaseObjects, and allObjects
(⬇ see below ) An example Shortcuts workflow (shortcut) demonstrating the use of the selection parameter in a script placed in the “Omni Automation Script” action for OmniFocus. (DOWNLOAD)
1 The selection Parameter • An array of references to the currently selected instances of the Project class is generated by appending the term “projects” to the parameter: selection.projects
2 Validation Check • If there is not a single selected project, throw an error to the in-script error handler.
3 Error Handler • Display the error information to the user, and then return an Error object to stop the workflow (shortcut).
4 Create an Object Reference • A JSON object is created and populated with the keys and project property values accepted as input by the next action. Note the use of the JSON.stringify() function to convert the JavaScript Object into a passable string. The resulting encoded object looks similar to this: "{\"primaryKey\":\"azPqtYRH\",\"type\":\"project\",\"version\":1}"
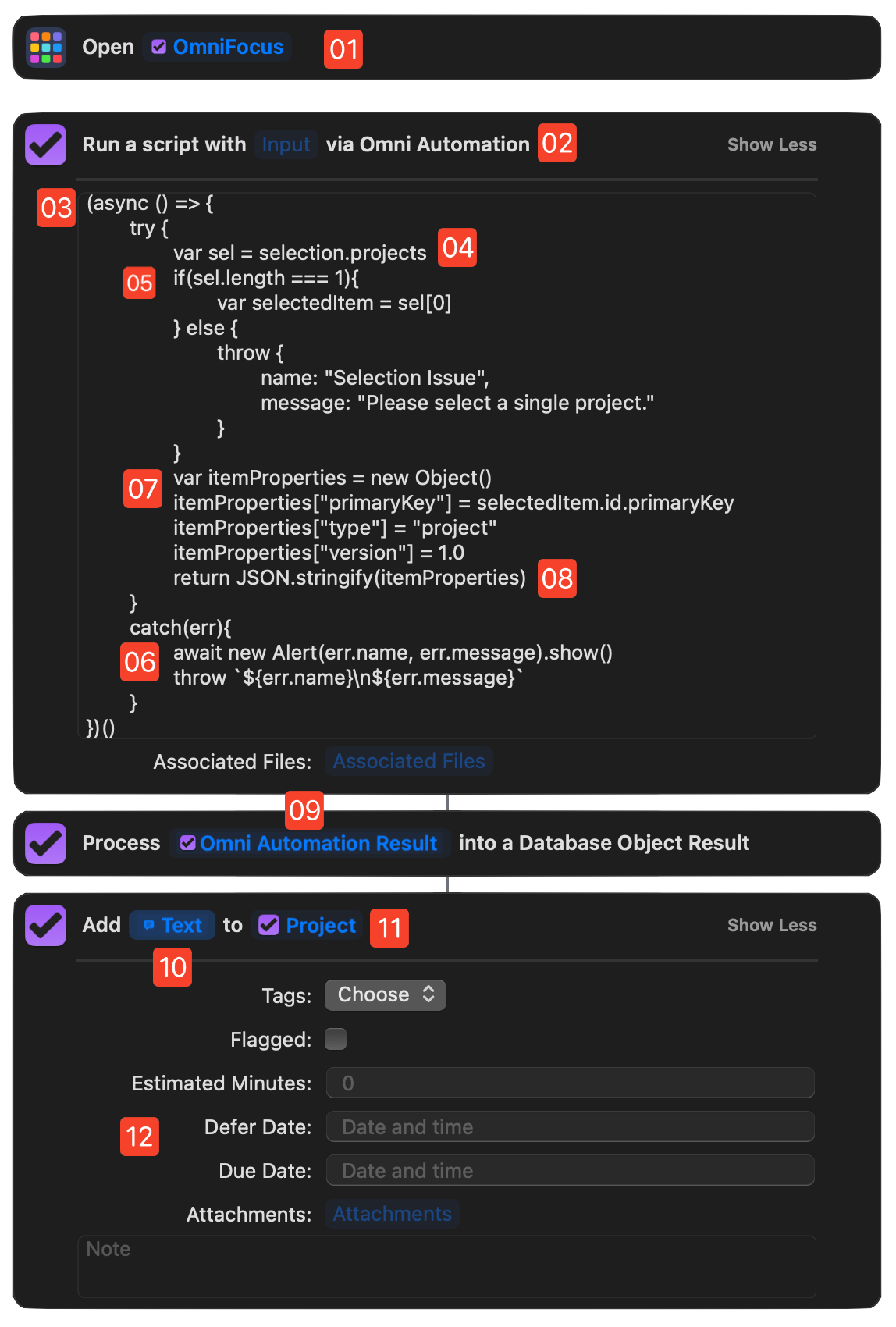
5 Data Input Socket • The encoded object reference to the selected project is passed into the action through a variable inserted into the Data Input Socket. The action converts the passed-in reference into an OmniFocus database object that is passed to the next OmniFocus action that creates and adds a new task to the selected project.
6 Data Input Socket • The passed project reference is placed into the Data Input Socket of the action for creating a new task. (see image below)
(⬇ see below ) Selecting the type of data to pass by selecting the variable placed in the Data Input Socket:
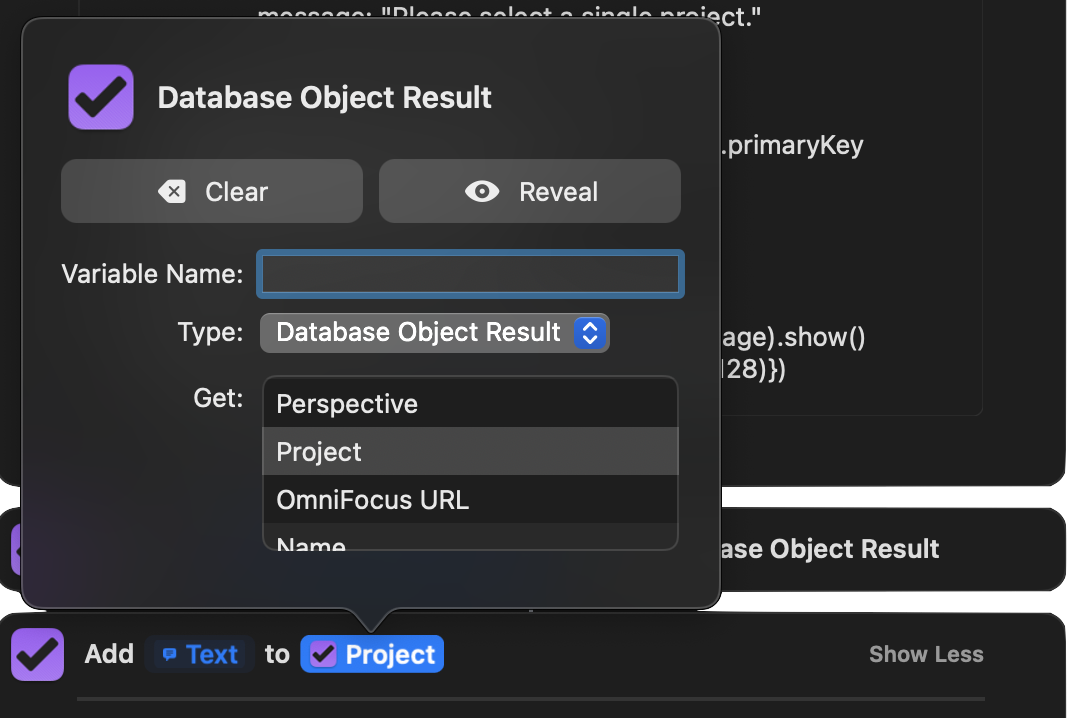
Pass Reference to Selected Project
(async () => {
try {
var sel = selection.projects
if(sel.length === 1){
var selectedItem = sel[0]
} else {
throw {
name: "Selection Issue",
message: "Please select a single project."
}
}
var itemProperties = new Object()
itemProperties["primaryKey"] = selectedItem.id.primaryKey
itemProperties["type"] = "project"
itemProperties["version"] = 1.0
return JSON.stringify(itemProperties)
}
catch(err){
if(!err.message.includes("cancelled")){
await new Alert(err.name, err.message).show()
}
throw `${err.name}\n${err.message}`
}
})()
Passing Object IDs
IMPORTANT: OmniFocus 4.5 introduces new Shortcuts actions for generating Object References from passed IDs and URLS. Documentation here.
When working with objects through a multiple-action shortcut, it may be useful to pass text-based object identifiers between the actions. An “identifier” is a property of an object whose value is unique to the host object. In OmniFocus the “identifier” of database objects is the value of their primaryKey property.
In the example shortcut below, object identifiers are passed both into and from the Omni Automation Script action in order to enable the processing of object to occur over multiple actions. (DOWNLOAD)
1 The “Find Items” action • This built-in OmniFocus Shortcuts action performs a database search for objects based upon the provided criteria.
2 “Repeat with Each” action • The repeat loop action iterates the array of data passed from the previous action. The passed variable has been set to return only the value of each object’s primaryKey property.
3 The Omni Automation Script action • Retrieves the identifier from a variable placed in the action’s Data Input Socket.
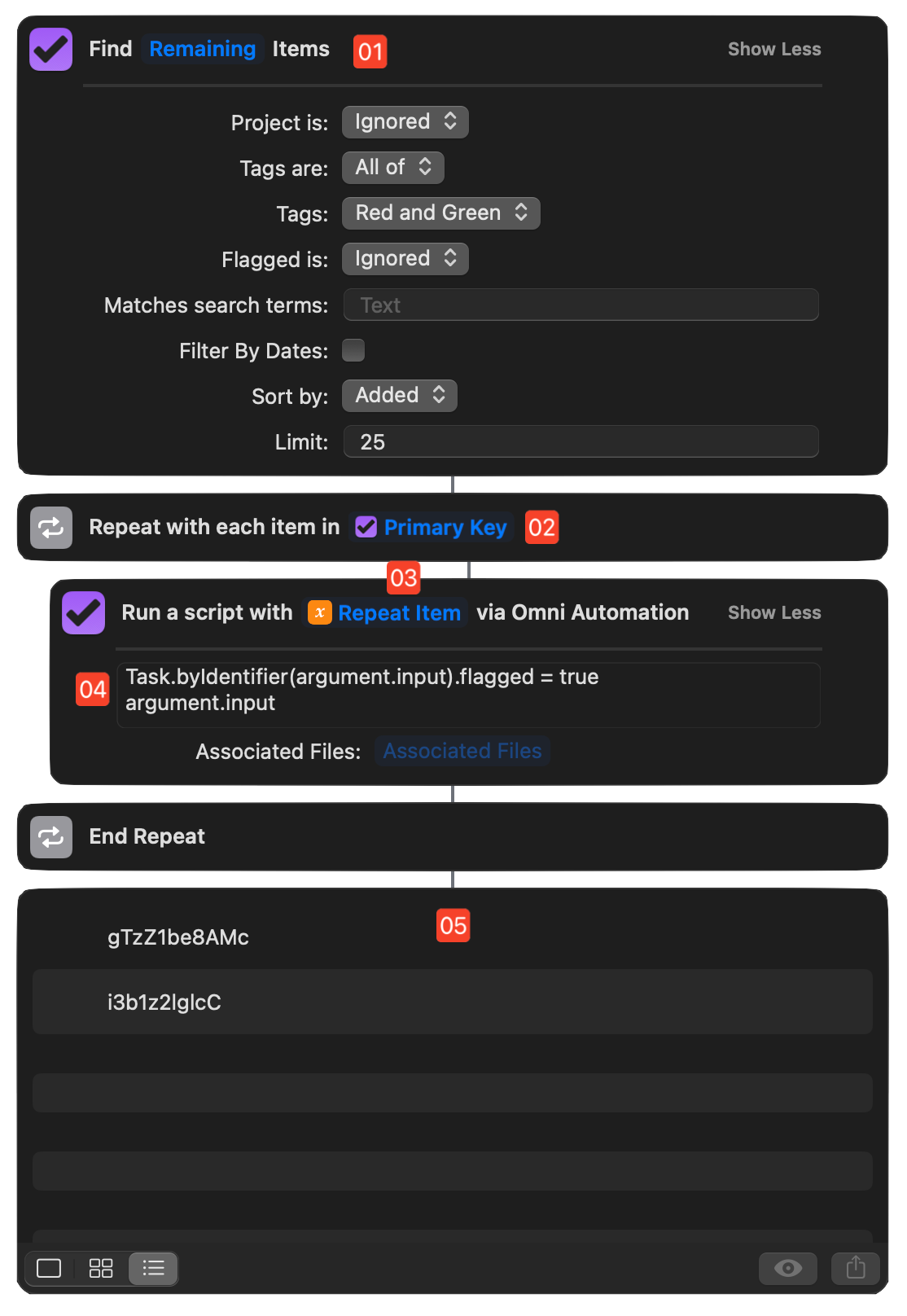
4 The Omni Automation Script action • Retrieves the passed identifier using the argument.input parameter, and then the script locates the parent object using its identifier and turns on its flag. The object’s primaryKey is then passed to the next action by placing the argument.input parameter as the last line of the script. NOTE: unless returned by a script function, the last line of a script is returned as the result of the action.
5 Identifiers of Found Items • The result of the repeat loop is an array of object identifiers that can be used by the next action in the shortcut.
Passing Object IDs as Array
The following shortcut shows how to pass an array of property values as input to the Omni Automation Script action. This accomplished by placing a “Set Variable” action to capture the results of the initial search action:
1 “Find Projects” action • The “Find Project” or “Find Items” action begins the workflow, set to search items matching the indicated parameters.
2 The “Set Variable” action • The variable to added is named, and…
3 Chosen Property • The Primary Key property is chosen from the popup menu summoned by activating this control.
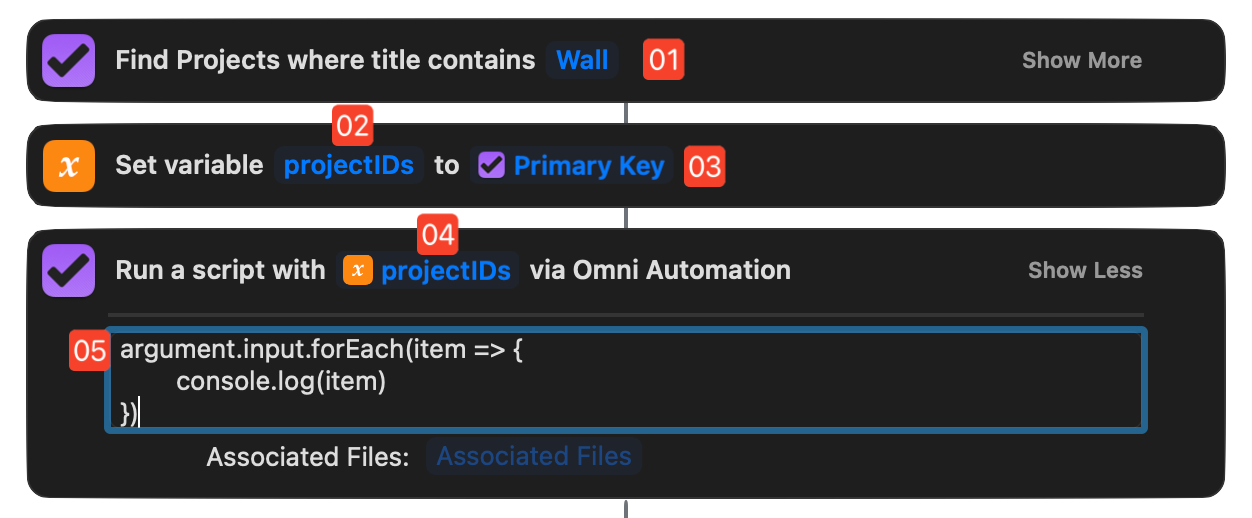
4 Data Input Socket • The resulting variable is added to the Data Input Socket of the Omni Automation Script action.
5 The argument.input Parameter • The array of object IDs is passed into the script via the argument.input parameter. The array of IDs can then be processed within the script using the standard JavaScript forEach() method.
Next…
The next section of this documentation demonstrates how the selection parameter is may be used with the “Omni Automation Script” action.