“Omni Automation Script” selection Parameter
Omni Automation plug-ins use the special parameter selection for representing the current document or application selection. This parameter is automatically passed into the plug-in action and validation handlers.
The various app-specific versions of the “Omni Automation Script” action employ a similar construct to provide access to the current Selection object to hosted script.
In the action’s script, the selection parameter can be used one or more times, anywhere within the script, and usually is appended with other terms to specify the type of objects to target. For example:
- OmniFocus: selection.projects • The currently selected projects.
- OmniGraffle: selection.canvas • The currently selected canvas.
- OmniOutliner: selection.items • The currently selected outline rows.
- OmniPlan: selection.resources • The currently selected project resources.
OmniGraffle • Set Fill Color of Selected Shapes
When used in an Omni Automation script targeting the OmniGraffle application, the selection parameter represents the Selection object generated by the script statement: document.windows[0].selection
In OmniGraffle, the types that can be appended to the selection parameter are: document, canvas, view, graphics, solids, and lines
(⬇ see below ) An example Shortcuts workflow (shortcut) demonstrating the use of the selection parameter in a script placed in the “Omni Automation Script” action for OmniGraffle. (DOWNLOAD)
1 “Open App” Shortcuts action • Make OmniGraffle the active application.
2 “Dictionary” Shortcuts action • A dictionary containing the numeric values to use for generating an RGB color object.
3 Data Input Socket • The variable containing the passed dictionary is placed in the Data Input Socket of the “Omni Automation Script” action.
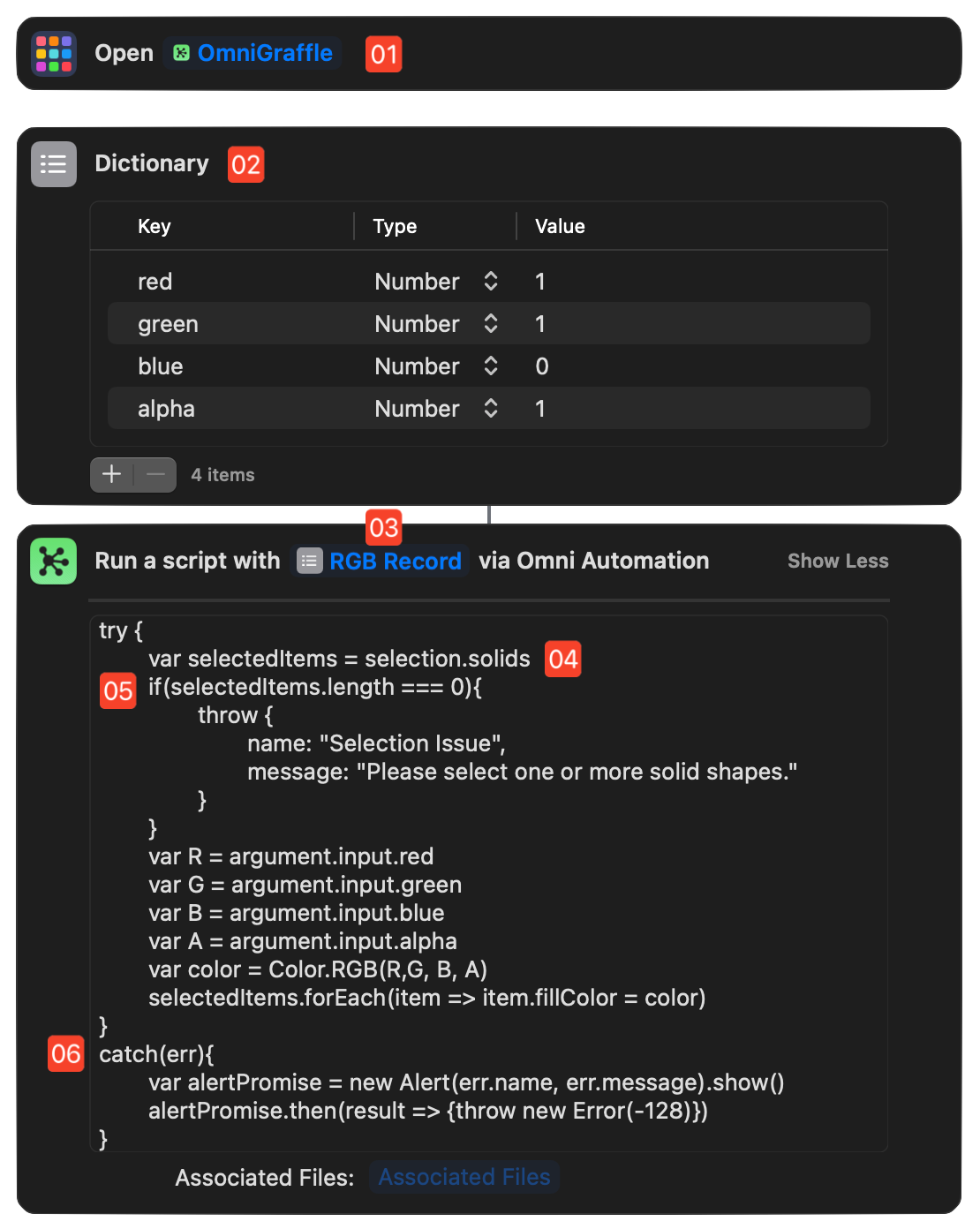
4 The selection Parameter • An array of references to the currently selected instance of the Solid class (non-line graphics) is generated by appending the term “solids” to the parameter: selection.solids
5 Validation Check • If there are no selected items, throw an error to the in-script error handler.
6 Error Handler • Display the error information to the user, and then return an Error object to stop the workflow (shortcut).
Change Fill Color of Selected Solids
try {
var selectedItems = selection.solids
if(selectedItems.length === 0){
throw {
name: "Selection Issue",
message: "Please select one or more shapes."
}
}
var R = argument.input.red
var G = argument.input.green
var B = argument.input.blue
var A = argument.input.alpha
var color = Color.RGB(R,G, B, A)
selectedItems.forEach(item => item.fillColor = color)
}
catch(err){
var alertPromise = new Alert(err.name, err.message).show()
alertPromise.then(result => {throw new Error(-128)})
}
OmniOutliner • Mark Selected Rows as DRAFT
When used in an Omni Automation script targeting the OmniOutliner application, the selection parameter represents the Selection object generated by the script statement: document.editors[0].selection
In OmniOutliner, the types that can be appended to the selection parameter are: allObjects, columns, document, editor, items, nodes, outline, and styles
(⬇ see below ) An example Shortcuts workflow (shortcut) demonstrating the use of the selection parameter in a script placed in the “Omni Automation Script” action for OmniOutliner. (DOWNLOAD)
1 “Open App” Shortcuts action • Make OmniOutliner the active application.
2 Data Input Socket • The Data Input Socket can hold text as well as data variables. In this workflow, the string “DRAFT” is passed into the script via the socket to be retrieved using the argument.input parameter.
3 The selection Parameter • An array of references to the currently selected outline rows is generated by appending the term “items” to the parameter: selection.items
4 Validation Check • If there are no selected items, throw an error to the in-script error handler.
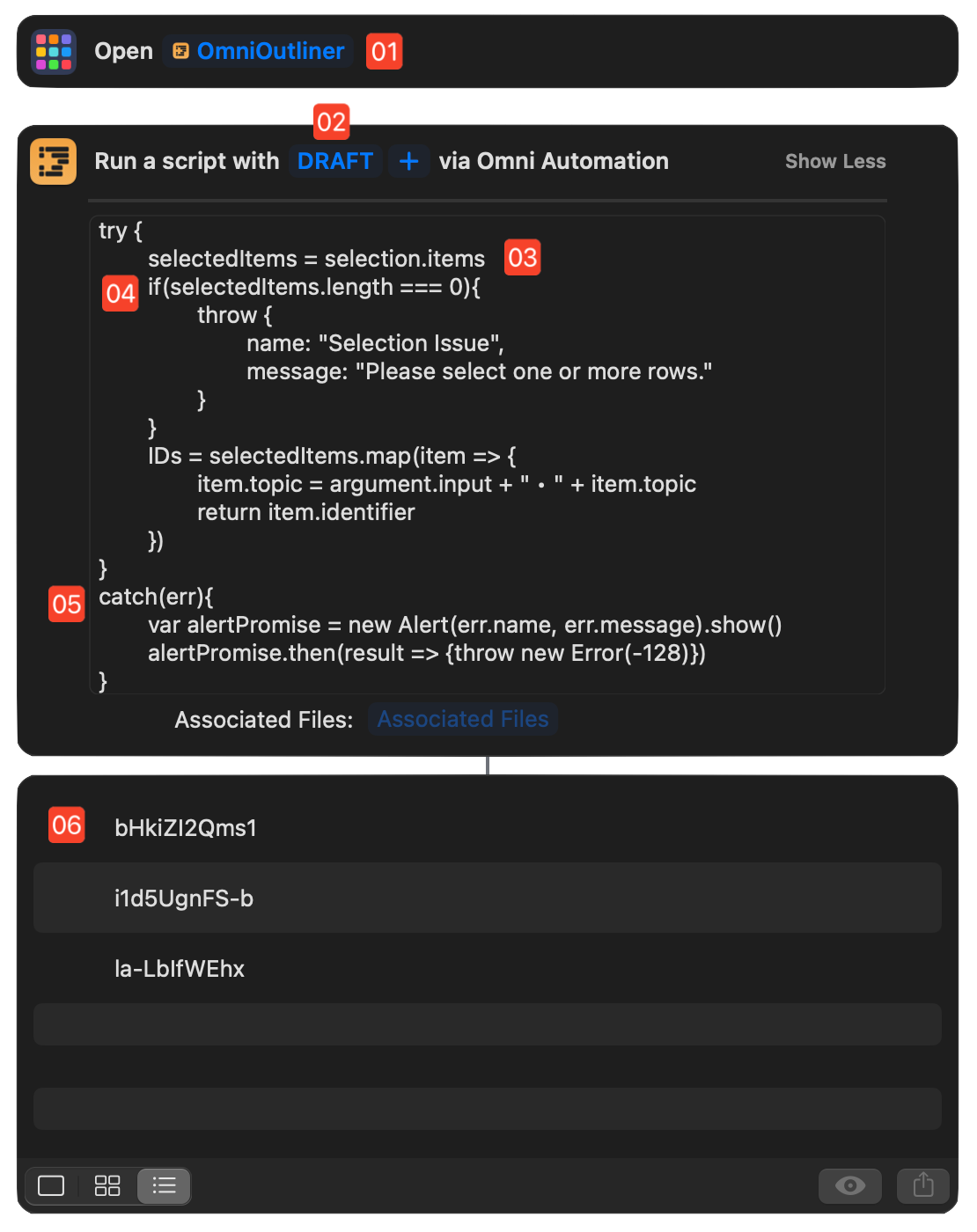
5 Error Handler • Display the error information to the user, and then return an Error object to stop the workflow (shortcut).
6 Action Result • The JavaScript map() function is used to process the rows and return an array of identifiers to the selected rows. The passed identifiers can be used by other actions to target the rows, like with this URL: omnioutliner:///open?row=aIAgE10lKLS
Mark Selected Rows as DRAFT
try {
selectedItems = selection.items
if(selectedItems.length === 0){
throw {
name: "Selection Issue",
message: "Please select one or more rows."
}
}
IDs = selectedItems.map(item => {
item.topic = argument.input + " • " + item.topic
return item.identifier
})
}
catch(err){
var alertPromise = new Alert(err.name, err.message).show()
alertPromise.then(result => {throw new Error(-128)})
}
OmniFocus • Add Task to Selected Project
When used in an Omni Automation script targeting the OmniFocus application, the selection parameter represents the Selection object generated by the script statement: document.windows[0].selection
In OmniFocus, the types that can be appended to the selection parameter are: tasks, projects, folders, tags, database, window, databaseObjects, and allObjects
(⬇ see below ) An example Shortcuts workflow (shortcut) demonstrating the use of the selection parameter in a script placed in the “Omni Automation Script” action for OmniFocus. (DOWNLOAD)
1 The selection Parameter • An array of references to the currently selected instances of the Project class is generated by appending the term “projects” to the parameter: selection.projects
2 Validation Check • If there is not a single selected project, throw an error to the in-script error handler.
3 Error Handler • Display the error information to the user, and then return an Error object to stop the workflow (shortcut).
4 Create an Object Reference • A JSON object is created and populated with the keys and project property values accepted as input by the next action. Note the use of the JSON.stringify() function to convert the JavaScript Object into a passable string. The resulting encoded object looks similar to this: "{\"primaryKey\":\"azPqtYRH\",\"type\":\"project\",\"version\":1}"
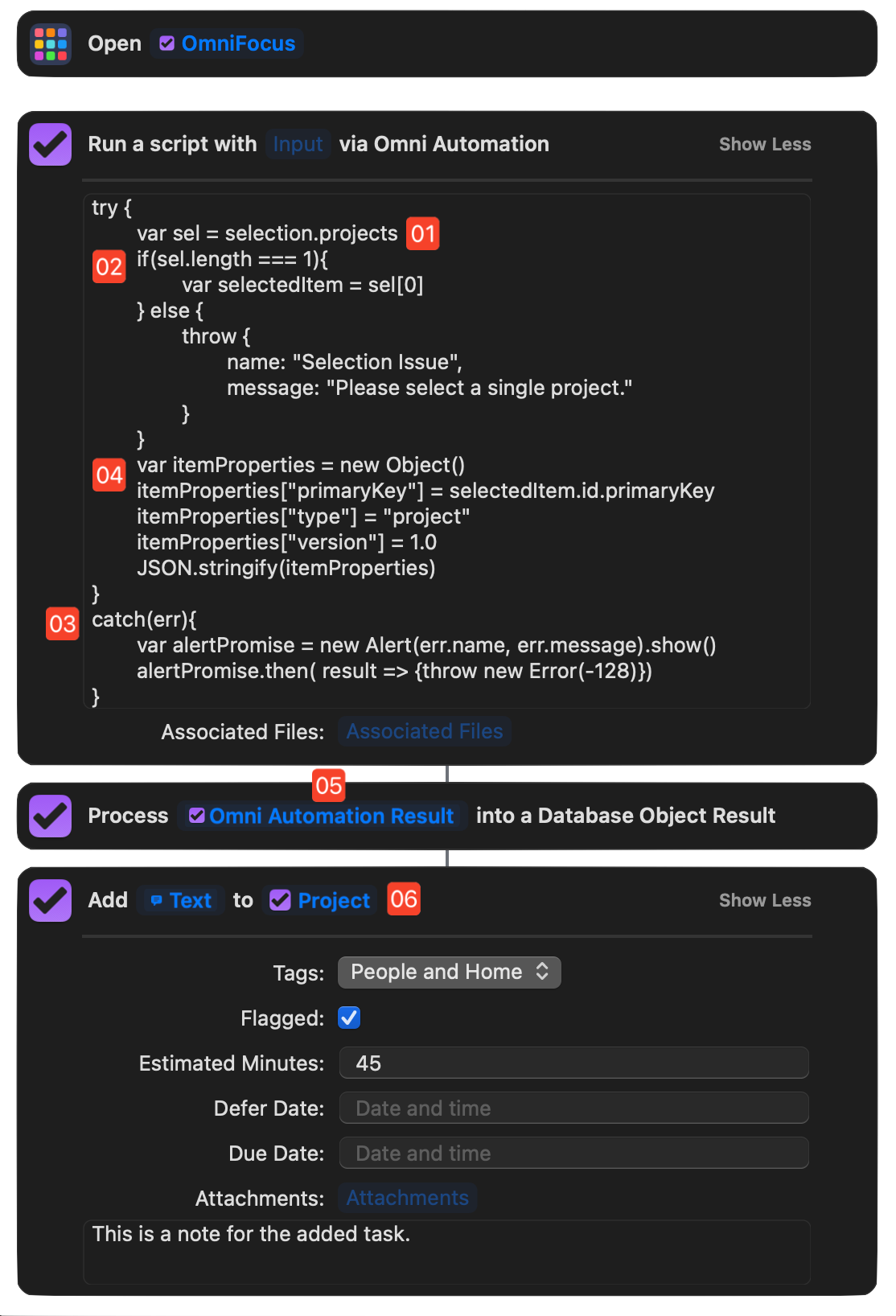
5 Data Input Socket • The encoded object reference to the selected project is passed into the action through a variable inserted into the Data Input Socket. The action converts the passed-in reference into an OmniFocus database object that is passed to the next OmniFocus action that creates and adds a new task to the selected project.
6 Data Input Socket • The passed project reference is placed into the Data Input Socket of the action for creating a new task. (see image below)
(⬇ see below ) Selecting the type of data to pass by selecting the variable placed in the Data Input Socket:
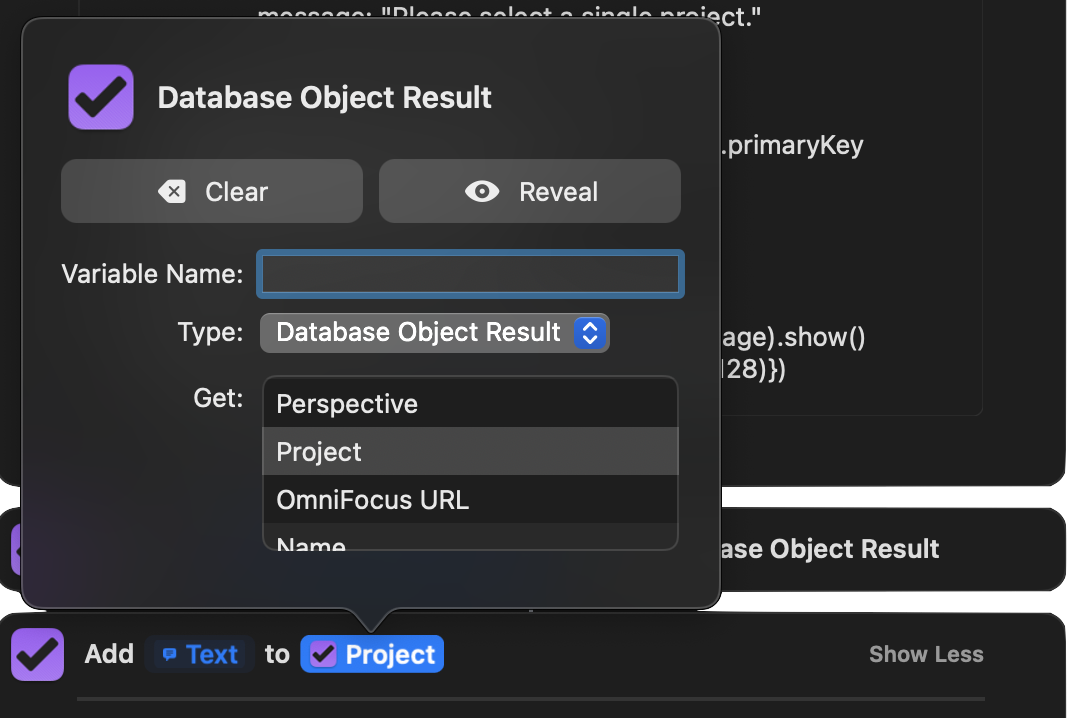
Pass Reference to Selected Project
try {
var sel = selection.projects
if(sel.length === 1){
var selectedItem = sel[0]
} else {
throw {
name: "Selection Issue",
message: "Please select a single project."
}
}
var itemProperties = new Object()
itemProperties["primaryKey"] = selectedItem.id.primaryKey
itemProperties["type"] = "project"
itemProperties["version"] = 1.0
JSON.stringify(itemProperties)
}
catch(err){
var alertPromise = new Alert(err.name, err.message).show()
alertPromise.then(result => {throw new Error(-128)})
}
And here’s a script that passes an array of IDs of the selected tasks:
Pass IDs of Selected Tasks
try {
var sel = selection.tasks
if(sel.length === 0){
throw {
name: "Selection Issue",
message: "Please select one or more tasks."
}
}
IDs = sel.map(item => item.id.primaryKey)
JSON.stringify(IDs)
}
catch(err){
var alertPromise = new Alert(err.name, err.message).show()
alertPromise.then(result => {throw new Error(-128)})
}
OmniPlan • Add Staff Resource to Selected Task from Contacts
When used in an Omni Automation script targeting the OmniGraffle application, the selection parameter represents the Selection object generated by the script statement: document.windows[0].selection
In OmniPlan, the types that can be appended to the selection parameter are: document, project, resources, tasks, and window
(⬇ see below ) An example Shortcuts workflow (shortcut) demonstrating the use of the selection parameter in a script placed in the “Omni Automation Script” action for OmniPlan. (DOWNLOAD)
1 Choose Contacts action • The built-in Shortcuts action for selecting items from the Contacts database.
2 Repeat with Each action • The built-in Shortcuts action for iterating the passed-in contact entries.
3 Dictionary action • Create a data dictionary using the fullname and email address of the contact. This data is used to locate them as a listed resource in the OmniPlan project. If they are not listed as an existing resource, a new Staff resource will be created with their data.
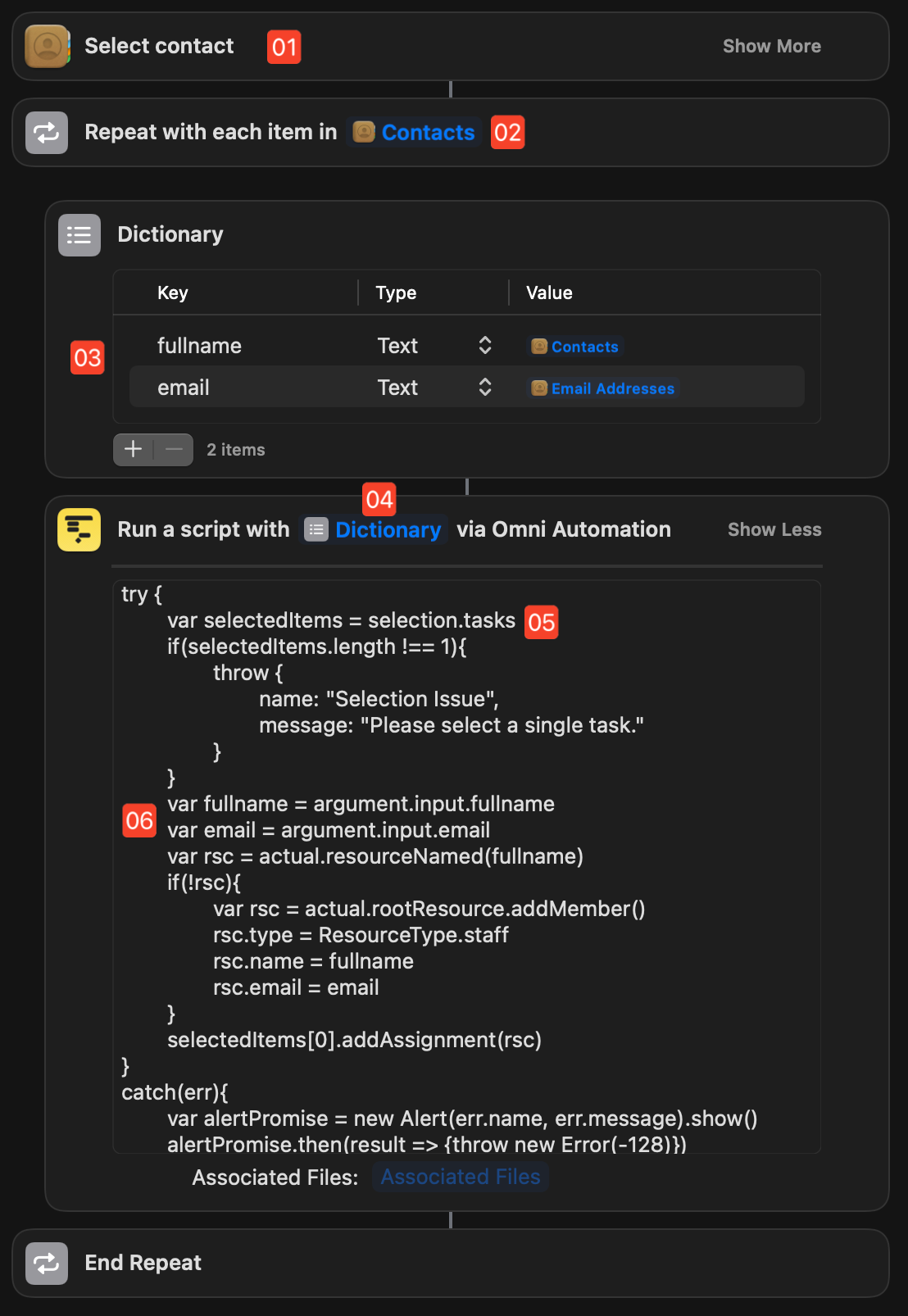
4 Data Input Socket • The dictionary containing the contact’s fullname and email values is passed into the Data Input Socket of the “Omni Automation Script” action.
5 The selection Parameter • The selection parameter is used to return object references to the tasks selected in the project window.
6 The argument.input Parameter • The values for the keys of the passed-in dictionary are retrieved by appending the key titles to the argument.input parameter.
Here’s the script code used in the example shortcut:
Assign/Create Resource from Passed Dictionary
try {
var selectedItems = selection.tasks
if(selectedItems.length !== 1){
throw {
name: "Selection Issue",
message: "Please select a single task."
}
}
var fullname = argument.input.fullname
var email = argument.input.email
var rsc = actual.resourceNamed(fullname)
if(!rsc){
var rsc = actual.rootResource.addMember()
rsc.type = ResourceType.staff
rsc.name = fullname
rsc.email = email.toString()
}
selectedItems[0].addAssignment(rsc)
}
catch(err){
var alertPromise = new Alert(err.name, err.message).show()
alertPromise.then(result => {throw new Error(-128)})
}
Next…
The next section of this documentation demonstrates how to add file references to the “Omni Automation Script” action via the action’s Associated Files control.