Calling the Shortcuts App
This section describes the various techniques for executing installed Shortcuts’ workflows from an Omni Automation plug-in.
1) Launch a Specified Shortcut
The most fundamental way to interact with Shortcuts in an Omni app is to simply run an Omni Automation plug-in that opens a special Shortcuts URL to launch one of the shortcuts you’ve already installed on your device.
To use this plug-in, follow these steps:
TAP|CLICK the “Download Plug-In” button in the example to download and then unpack the ZIP archive containing the plug-in file.
Open the plug-in file with the text-editor application you use to write your scripts.
In the opened plug-in document, replace the placeholder “Shortcut Title” at line 14 with the title of the Shortcut workflow you wish to launch. A corresponding Shortcuts workflow named with the indicated title will need to exist on our device when you run the plug-in!
In the opened plug-in document, you may optionally change the menu/toolbar title of the plug-in by replacing either or both of the “Launch Shortcut” placeholders at line 8 and 9 in the script.
Save your edits, and place the plug-in file in your designated Omni Plug-Ins folder. Detailed instructions for plug-in installation are available here.
NOTE: By default, this plug-in is designed to work with any of the Omni suite of productivity apps: OmniFocus, OmniGraffle, OmniPlan, and OmniOutliner. If you wish, you can edit the list of supported apps in line 3 to include just those Omni apps in which you want this plug-in to be available.
NOTE: Documentation concerning the Shortcuts URL schema is available in the Apple Support Documentation
Launch Specified Shortcut
/*{
"type": "action",
"targets": ["omnifocus",
"omnigraffle", "omniplan", "omnioutliner"], "author": "Otto Automator",
"identifier": "com.omni-automation.all.launch-shortcut",
"version": "1.0",
"description": "This action will launch the specified Shortcuts workflow.",
"label": "Launch Shortcut",
"shortLabel": "Launch Shortcut",
"paletteLabel": "Shortcut",
"image": "gearshape.2.fill"
}*/
(() => {
const action = new PlugIn.Action(function(selection, sender){
let shortcutTitle = "Shortcut Title"
shortcutTitle = encodeURIComponent(shortcutTitle)
let urlStr = "shortcuts://run-shortcut?name=" + shortcutTitle
URL.fromString(urlStr).open()
});
action.validate = function(selection, sender){
return true
};
return action;
})();
2) Launch a Chosen Shortcut
While the following action plug-in is functionally the same as the previous one, it provides the extra ability to choose the targeted workflow from a list of workflows when the plug-in is run.
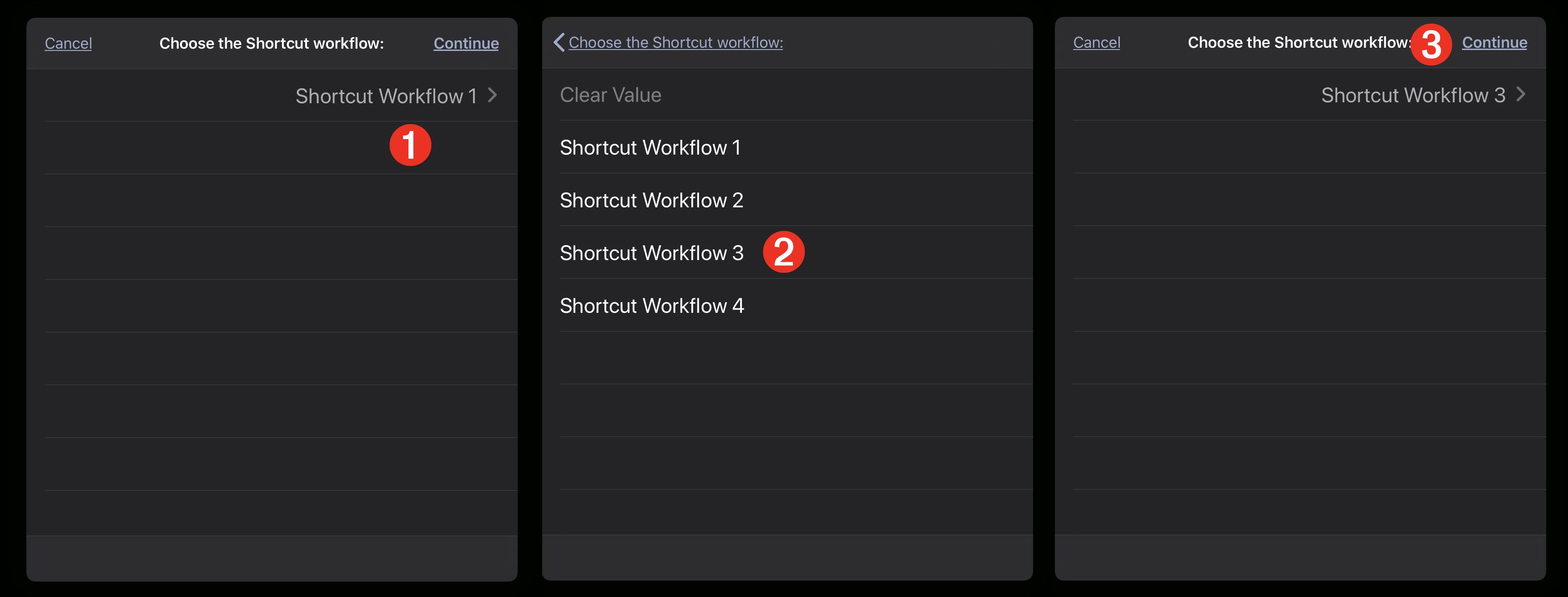
(⬆ see above ) 1 Tap the default item to reveal the full list of specified workflows. 2 Select one of the workflow titles from the list of workflow titles 3 Tap “Continue” to launch the chosen workflow.
To use this plug-in, follow these steps:
TAP|CLICK the “Download Plug-In” button in the example to download and then unpack the ZIP archive containing the plug-in file.
Open the plug-in file with the text-editor application you use to write your scripts.
In the opened plug-in document, replace the list of placeholder shortcut titles at lines 15-20 with the titles of the installed Shortcuts workflows you wish to be available for launch. Your list of titles can be as long as you want, but remember that a corresponding Shortcuts workflow named with each of the indicated titles will need to exist on our device before you run the plug-in!
In the opened plug-in document, you may optionally change the menu/toolbar title of the plug-in by replacing either or both of the “Launch Chosen Shortcut” placeholders at line 8 and 9 in the script.
Save your edits, and place the plug-in file in your designated Omni Plug-Ins folder. Detailed instructions for plug-in installation are available here.
NOTE: By default, this plug-in is designed to work with any of the Omni suite of productivity apps: OmniFocus, OmniGraffle, OmniPlan, and OmniOutliner. If you wish, you can edit the list of supported apps in line 3 to include just those Omni apps in which you want this plug-in to be available.
Launch Chosen Shortcut
/*{
"type": "action",
"targets": ["omnifocus",
"omnigraffle", "omniplan", "omnioutliner"], "author": "Otto Automator",
"identifier": "com.omni-automation.all.launch-chosen-shortcut",
"version": "1.0",
"description": "This action will launch the Shortcuts shortcut (workflow) chosen from the presented form menu.",
"label": "Launch Chosen Shortcut",
"shortLabel": "Launch Chosen Shortcut",
"paletteLabel": "Shortcut",
"image": "gearshape.2.fill"
}*/
(() => {
const action = new PlugIn.Action(function(selection, sender){
// action code
var menuItems = [
"Shortcut 1",
"Shortcut 2",
"Shortcut 3",
"Shortcut 4"
]
var menuIndexes = menuItems.map((item,index) => {return index})
var menuElement = new Form.Field.Option(
"menuElement",
null,
menuIndexes,
menuItems,
0
)
var inputForm = new Form()
inputForm.addField(menuElement)
var formPrompt = "Choose the Shortcuts workflow:"
var buttonTitle = "Continue"
var formPromise = inputForm.show(formPrompt,buttonTitle)
inputForm.validate = function(formObject){
return true
}
formPromise.then(function(formObject){
var menuIndex = formObject.values['menuElement']
var shortcutTitle = menuItems[menuIndex]
console.log(shortcutTitle)
shortcutTitle = encodeURIComponent(shortcutTitle)
var urlStr = "shortcuts://run-shortcut?name=" + shortcutTitle
URL.fromString(urlStr).open()
})
formPromise.catch(function(err){
console.error("form cancelled", err.message)
})
});
action.validate = function(selection, sender){
// validation code
return true
};
return action;
})();
Here is an asynchronous version of the previous plug-in:
Launch Chosen Shortcut
/*{
"type": "action",
"targets": ["omnifocus","omnigraffle","omniplan","omnioutliner"],
"author": "Otto Automator",
"identifier": "com.omni-automation.all.launch-chosen-shortcut",
"version": "1.0",
"description": "This action will launch the Shortcuts shortcut (workflow) chosen from the presented form menu.",
"label": "Launch Chosen Shortcut",
"shortLabel": "Launch Chosen Shortcut",
"paletteLabel": "Shortcut",
"image": "gearshape.2.fill"
}*/
(() => {
const action = new PlugIn.Action(async function(selection, sender){
try {
menuItems = [
"Shortcut 1",
"Shortcut 2",
"Shortcut 3",
"Shortcut 4"
]
menuIndexes = menuItems.map((item, index) => {return index})
menuElement = new Form.Field.Option(
"menuElement",
null,
menuIndexes,
menuItems,
0
)
inputForm.validate = function(formObject){
return true
}
var inputForm = new Form()
inputForm.addField(menuElement)
formPrompt = "Choose workflow:"
buttonTitle = "Continue"
formObject = await inputForm.show(formPrompt, buttonTitle)
menuIndex = formObject.values['menuElement']
shortcutTitle = menuItems[menuIndex]
shortcutTitle = encodeURIComponent(shortcutTitle)
urlStr = "shortcuts://run-shortcut?name=" + shortcutTitle
URL.fromString(urlStr).open()
}
catch(err){
if(!err.causedByUserCancelling){
new Alert(err.name, err.message).show()
}
}
});
action.validate = function(selection, sender){
// validation code
return true
};
return action;
})();
3) Run and Process Shortcut
In the following example plug-in, a specified Shortcuts workflow is launched and the result of the workflow is processed by the plug-in. This plug-in incorporates the same Shortcuts URL as the previous examples, but the URL is executed using the call() function of the URL class instead of the open() handler. The call() function employs an x-callback-url that enables the Shortcuts app to return the result of its workflow execution to the calling plug-in.
To adapt this plug-in for your use, follow these steps:
TAP|CLICK the “Download Plug-In” button in the example to download and then unpack the ZIP archive containing the plug-in file.
Open the plug-in file with the text-editor application you use to write your scripts.
In the opened plug-in document, replace the placeholder “Shortcuts Title” at line 14 with the title of the Shortcut workflow you wish to launch. A corresponding Shortcuts workflow named with the indicated title will need to exist on our device when you run the plug-in. The target workflow also needs to return the value used by the script.
In the opened plug-in document, you may optionally change the menu/toolbar title of the plug-in by replacing either or both of the “Run Shortcut” placeholders at line 8 and 9 in the script.
In the opened plug-in document, replace lines 20-22 with your statements for processing the workflow result.
Save your edits, and place the plug-in file in your designated Omni Plug-Ins folder. Detailed instructions for plug-in installation are available here.
NOTE: By default, this plug-in is designed to work with any of the Omni suite of productivity apps: OmniFocus, OmniGraffle, OmniPlan, and OmniOutliner. If you wish, you can edit the list of supported apps in line 3 to include just those Omni apps in which you want this plug-in to be available.
Run Shortcut and Process Results
/*{
"type": "action",
"targets": ["omnifocus",
"omnigraffle", "omniplan", "omnioutliner"], "author": "Otto Automator",
"identifier": "com.omni-automation.all.run-shortcut",
"version": "1.1",
"description": "This action will run the specified Shortcuts workflow and process the result passed back to the script.",
"label": "Run Shortcut",
"shortLabel": "Run Shortcut",
"paletteLabel": "Shortcut",
"image": "gear"
}*/
(() => {
const action = new PlugIn.Action(function(selection, sender){
// action code
var shortcutTitle = "Shortcut Title"
shortcutTitle = encodeURIComponent(shortcutTitle)
var urlStr = "shortcuts://run-shortcut?name=" + shortcutTitle
var url = URL.fromString(urlStr)
url.call(function(result){
console.log(result)
// PROCESS RESULTS OF WORKFLOW
}, function(err){
new Alert("SCRIPT ERROR", err.errorMessage).show()
console.error(err.errorMessage)
})
});
action.validate = function(selection, sender){
return true
};
return action;
})();
In this example a Shortcuts workflow titled “Address Book” is called to retrieve the name and phone number of a chosen contact:
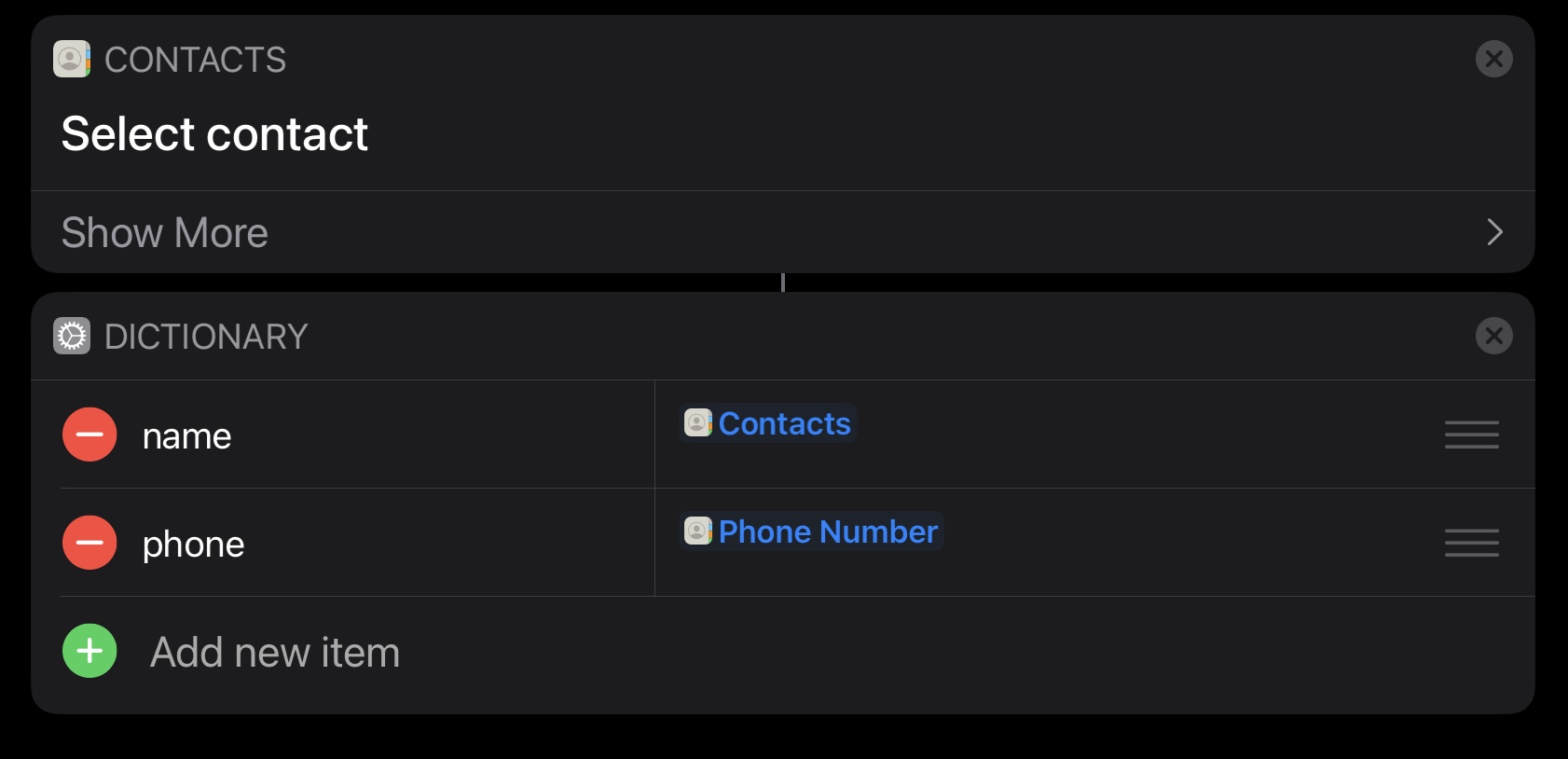
Address Book Example
/*{
"type": "action",
"targets": ["omnifocus",
"omnigraffle", "omniplan", "omnioutliner"], "author": "Otto Automator",
"identifier": "com.omni-automation.all.address-book",
"version": "1.0",
"description": "This action will run the specified Shortcuts workflow and process the result passed back to the script.",
"label": "Address Book",
"shortLabel": "Address Book",
"paletteLabel": "Address",
"image": "person.circle"
}*/
(() => {
const action = new PlugIn.Action(function(selection, sender){
// action code
var shortcutTitle = "Address Book"
shortcutTitle = encodeURIComponent(shortcutTitle)
var urlStr = "shortcuts://run-shortcut?name=" + shortcutTitle
var url = URL.fromString(urlStr)
url.call(function(resultObj){
var contactName = resultObj.name
var contactPhone = resultObj.phone
// PROCESS RESULTS OF WORKFLOW
}, function(err){
new Alert("SCRIPT ERROR", err.errorMessage).show()
console.error(err.errorMessage)
})
});
action.validate = function(selection, sender){
return true
};
return action;
})();