Point
A Point is a class describing the position of a graphic. It has two elements, the horizontal offset (in points) and the vertical offset (in points) of the object from the canvas origin. A Point is also the first component of a Rect, which describes both the location (Point) and dimensions (Size) of a graphic.
Creating a Point
An instance of the Point class is created by preceding the class name with the new item constructor, and following the class name with the horizontal offset (in points), and vertical offset (in points) of the object, enclosed in a pair of parens (). The result is a new instance of the Point class:
new Point(horizontalOffset, verticalOffset) (Point) • Returns a new Point with the specified coordinates.
Create a New Point
pt = new Point(72, 90)
//--> [object Point: (72.0, 90.0)]
Class Properties
By default, the Point class has three intrinsic properties for creating a defined point.
unitX (Point r/o) • Returns a Point with coordinates (1, 0)
unitY (Point r/o) • Returns a Point with coordinates (0, 1)
zero (Point r/o) • Returns the Point (0, 0), the origin.
Point Properties
ptX = Point.unitX
//--> [object Point: (1.0, 0.0)]
ptY = Point.unitY
//--> [object Point: (0.0, 1.0)]
pt0 = Point.zero
//--> [object Point: (0.0, 0.0)]
Instance Properties
An instance of the Point class has five properties which can be accessed by scripts: length, negative, normalized, x, and y
length (Number r/o) • Returns the distance between the receiver and the origin.
The Length of a Point
pt = new Point(200, 200)
pt.length
//--> 282.842712474619
The value of the length property is the Point’s distance from the canvas origin. In essence, this value is length of the hypotenuse of the right triangle formed by the horizontal and vertical offsets of the point. It is determined using the Pythagorean theorem.
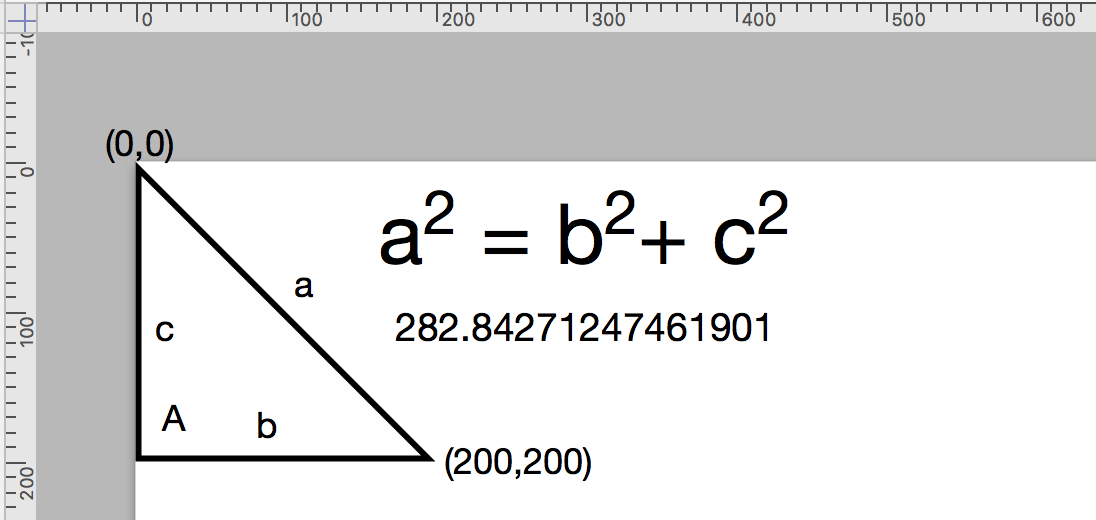
negative (Number r/o) • Returns the component-wise negative of the receiver.
The Negative of a Point
pt = new Point(100, 200)
pt.negative
//--> [object Point: (-100.0, -200.0)]
normalized (Number r/o) • For a non-zero point, returns a point with a distance of one from the origin, but in the same direction as the original. For the zero point, this returns the receiver.
Normalized Point
pt = new Point(100, 200)
pt.normalized
//--> [object Point: (0.447213595499958, 0.894427190999916)]
x (Number r/o) • The horizontal axis coordinate of the Point.
The Horiontal Component of a Point
pt = new Point(100, 200)
pt.x
//--> 100
y (Number r/o) • The verticql axis coordinate of the Point.
The Vertical Component of a Point
pt = new Point(100, 200)
pt.y
//--> 200
Instance Functions
There are three functions you can use to derive a new point based upon an instance of a point, and two functions for deriving a measurement from a point instance.
add(point: Point) → (Point) • Returns a new Point that is the component-wise sum of the receiver and the argument Point
subtract(point: Point) → (Point) • Returns a new Point that is the component-wise difference of the receiver and the argument Point
scale(factor: Number) → (Point) • Returns a new Point where each component is scaled by the given factor.
distanceTo(point: Point) → (Number) • Returns the distance between the receiver and the given Point
dot(point: Point) → (Number) • Returns the dot product between the receiver and the given Point.
Here’s an example script that uses the add() instance function with the duplicateTo() graphic function to create a diagonal row of squares:
Adding Points
cnvs = document.windows[0].selection.canvas
side = 100
pt = new Point(side,side)
shape = cnvs.addShape('Rectangle',new Rect(0, 0, side, side))
for(i = 0; i < 3; i++){
shape = shape.duplicateTo(shape.geometry.origin.add(pt))
}
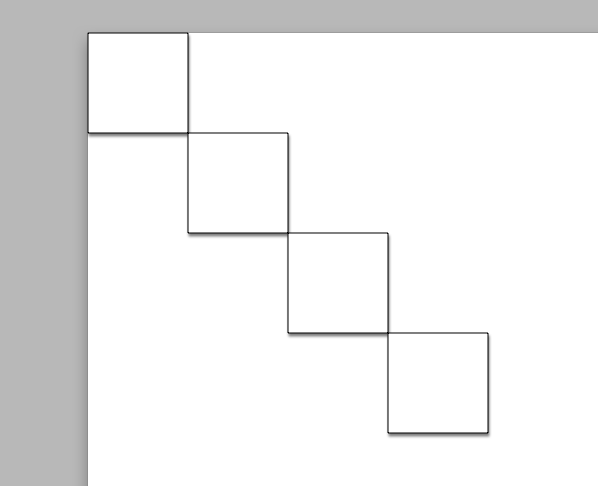
Using the scale function to diagonally duplicate a shape:
Using Scale Function
cnvs = document.windows[0].selection.canvas
shape = cnvs.addShape('Rectangle',new Rect(0, 0, 200, 200))
shape.duplicateTo(shape.geometry.center.scale(2))
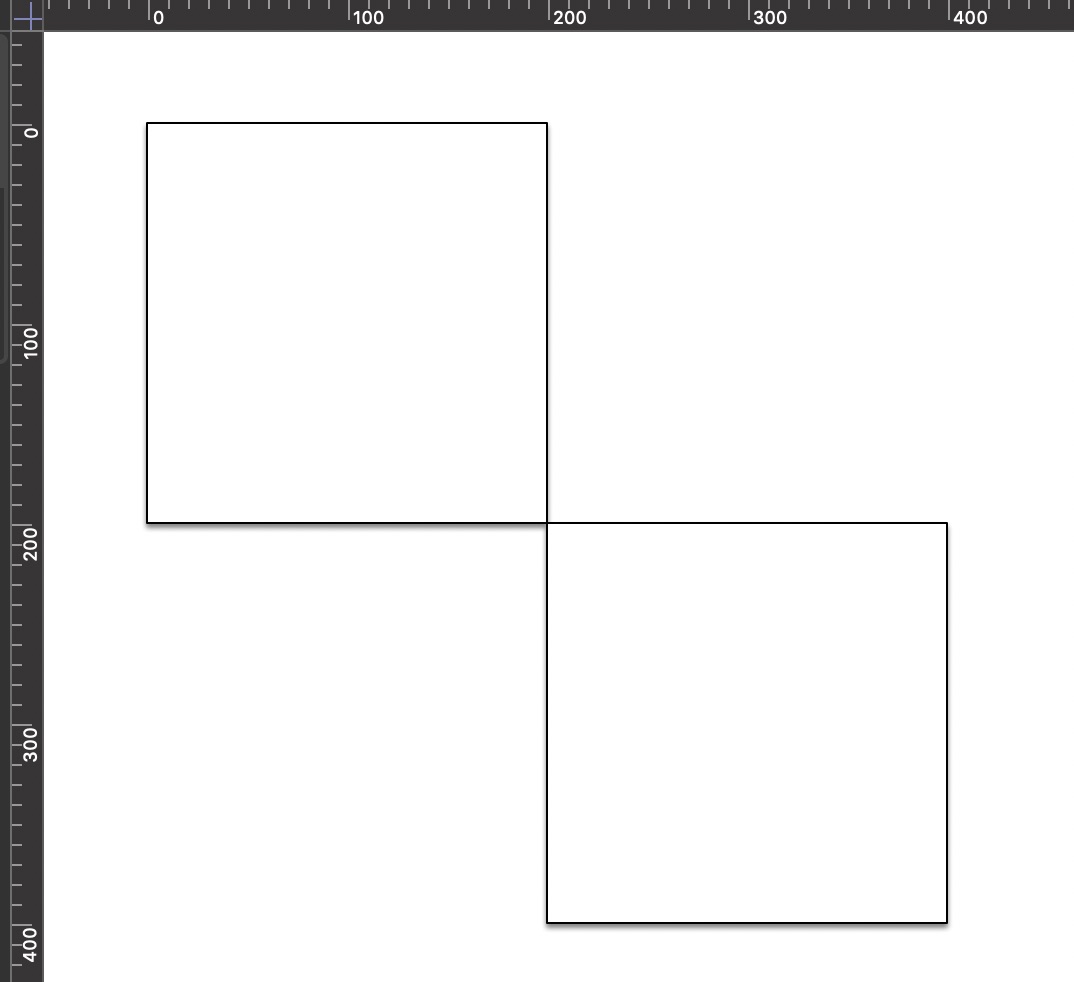