Scenarios
A Scenario represents a set of tasks and resources and associated schedules. The actuals for a project are one scenario, and saved baselines are the others.
Instance Properties
The properties of a scenario:
completed (Number) • Overall project percentage completed.
endDate (Date) • Overall project end date. Setting a date value to this property will change scenario to: “Backward from fixed end” 01 (see below)
hasFixedEndDate (Boolean) • Whether tasks in this scenario scheduled forward from a fixed overall start, or backwards from a fixed overall end.
(true = “Backward from fixed end”) (false = “Forward from fixed start”) 01 (see below)hasGenericDates (Boolean) • Generic dates (e.g. “Day 1”, “Day 12”) vs actual dates (e.g. “Jan 1, 2022”).
(true = Dates:To Be Determined) (false = Dates:Specific) 02 (see below)milestones (Array of Task r/o) • A list of all milestones in the project.
name (String) • The name of this scenario.
rootResource (Resource r/o) • The root resource of the project. This is a hidden group which contains all top-level resources.
rootTask (Task r/o) • Root task of the project. This is a hidden group which contains all top-level tasks.
schedule (Schedule r/o) • The overall project schedule.
startDate (Date) • Overall project start date. Setting a date value to this property will change scenario to: “Forward from fixed start” 01 (see below)
totalCost (Number r/o) • Overall project total cost.
The Fixed Start/End and Specific/Relative Dates Properties
(⬇ see below ) The hasFixedEndDate and hasGenericDates properties control the input and display of dates for the scenario. 03 This illustration shows a project with a fixed end: 01
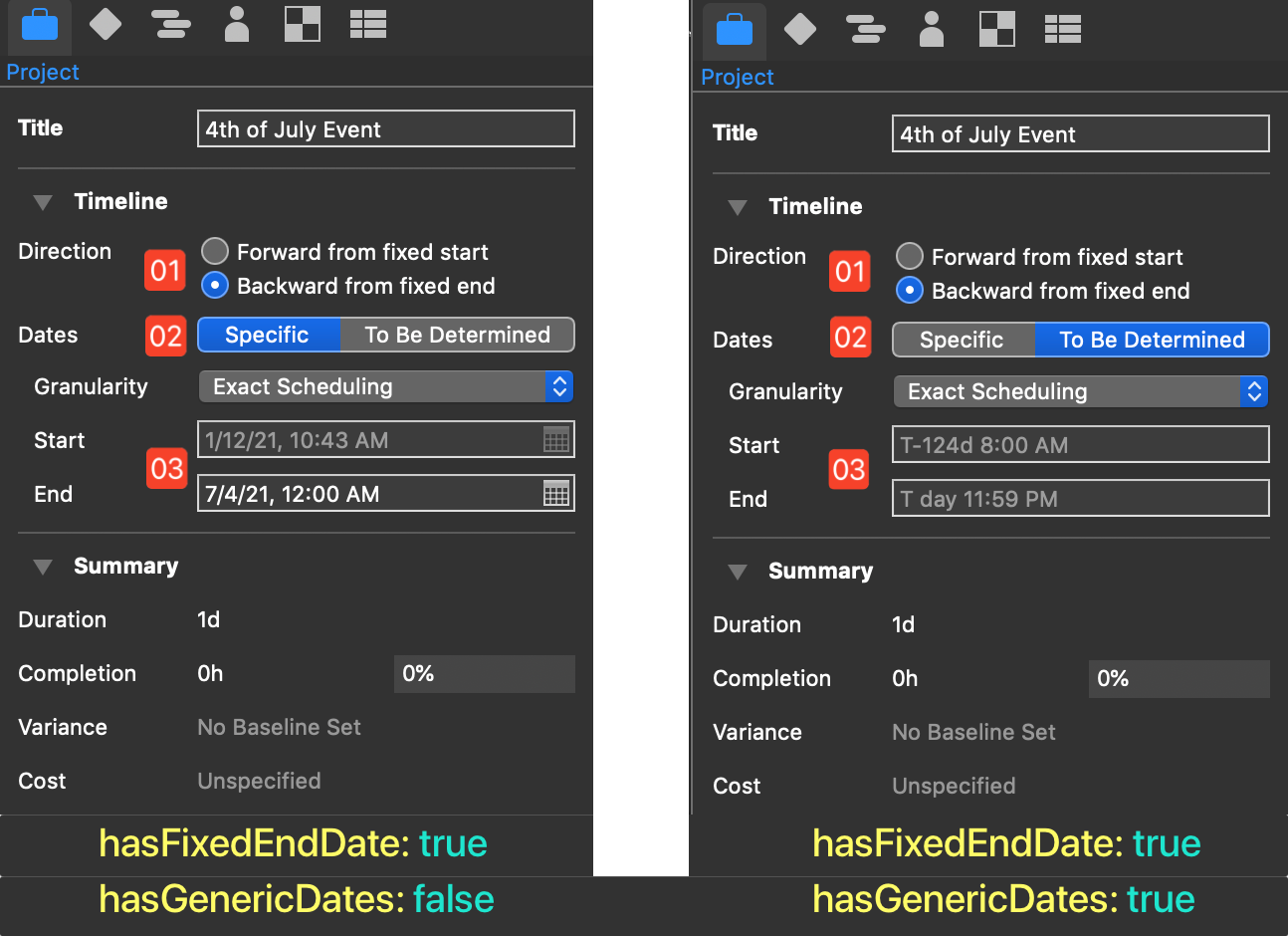
(⬇ see below ) The hasFixedEndDate and hasGenericDates properties control the input and display of dates for the scenario. 03 This illustration shows a project with a fixed start: 01
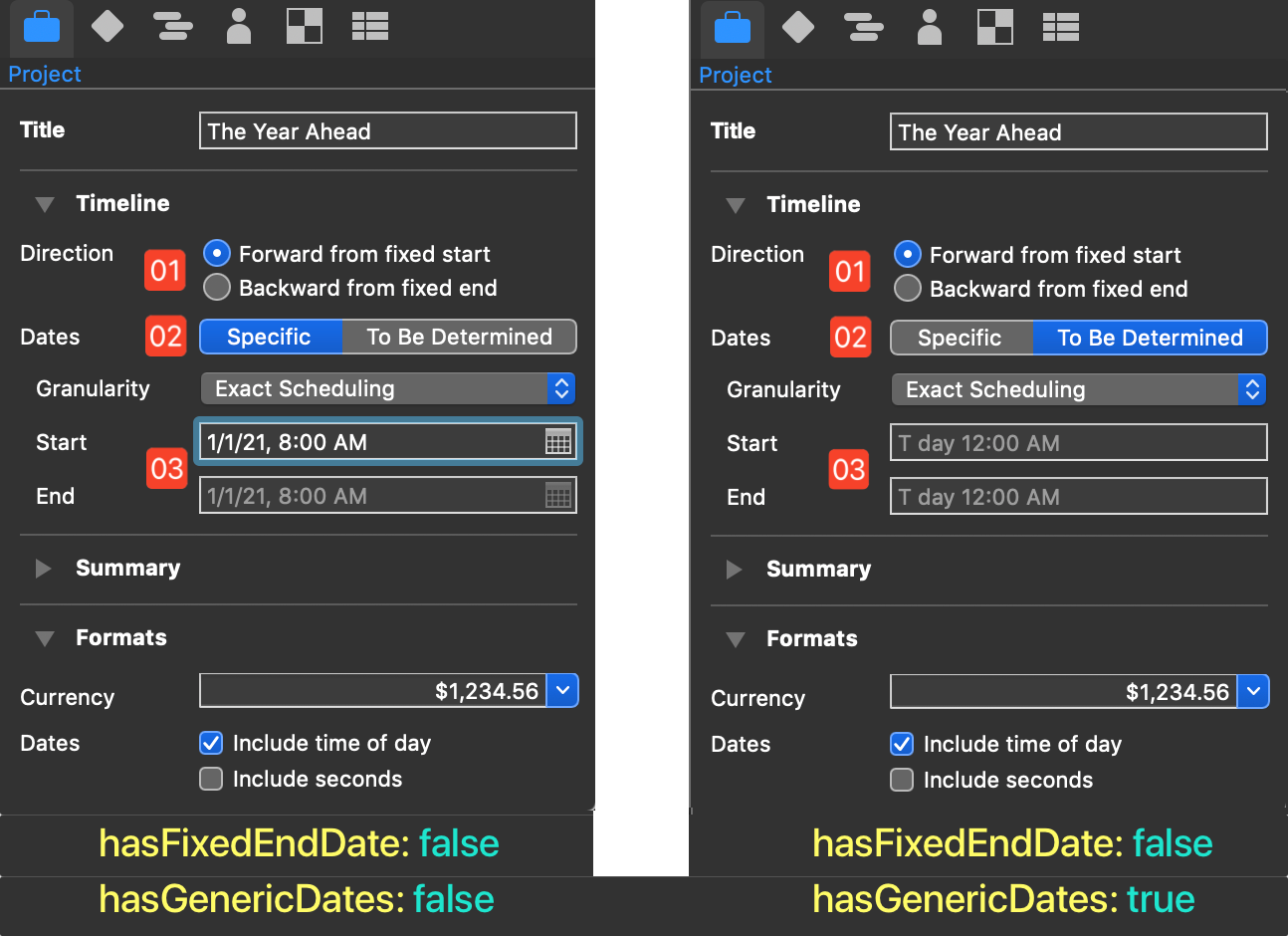
Examples of Scenario properties used in scripts:
Scenario Properties
actual.name
//--> "Actual"
actual.completed
//--> 23
// top-level tasks
var tasks = actual.rootTask.subtasks
// all tasks
var allTasks = actual.rootTask.descendents()
// new top-level task
var newTask = actual.rootTask.addSubtask()
// top-level resources
var resources = actual.rootResource.members
// all resources)
var allResources = actual.rootResource.descendents()
// new top-level resource
var newResource = actual.rootResource.addMember()
// all milestones
actual.milestones
Total Cost Property
Show Total Project Cost (USA Dollars)
var projectCost = actual.totalCost
//--> [object Decimal: 9000]
var code = new Locale("en_US").currencyCode
//--> "USD"
var fmtr = Formatter.Decimal.currency(code)
var costString = fmtr.stringFromDecimal(projectCost)
//--> "$9,000.00"
new Alert("Total Cost", costString).show()
NOTE: Information regarding Locale codes can be found in the Calendar class documentation. Documentation regarding the Decimal Formatter class can be found here.
Instance Functions
Instance functions for the Scenario class.
resourceNamed(name:String) → (Resource or null) • Get a given resource by name. If there is more than one with the same name, only returns the first.
taskNamed(name:String) → (Task or null) • Get a given task by name. If there is more than one with the same name, only returns the first.
Assigning Resource to New Task
var rsc = actual.resourceNamed("Margret Jensen")
if(rsc){
var task = actual.rootTask.addSubtask()
task.addAssignment(rsc)
}
NOTE: Both the taskNamed() and the resourceNamed() functions search the entire chain of tasks and resources contained within the container the function is called on, even locating tasks within other tasks. In the example shown below, the taskNamed() function is called on the main project scenario (actual) and so will search the entire scenario.
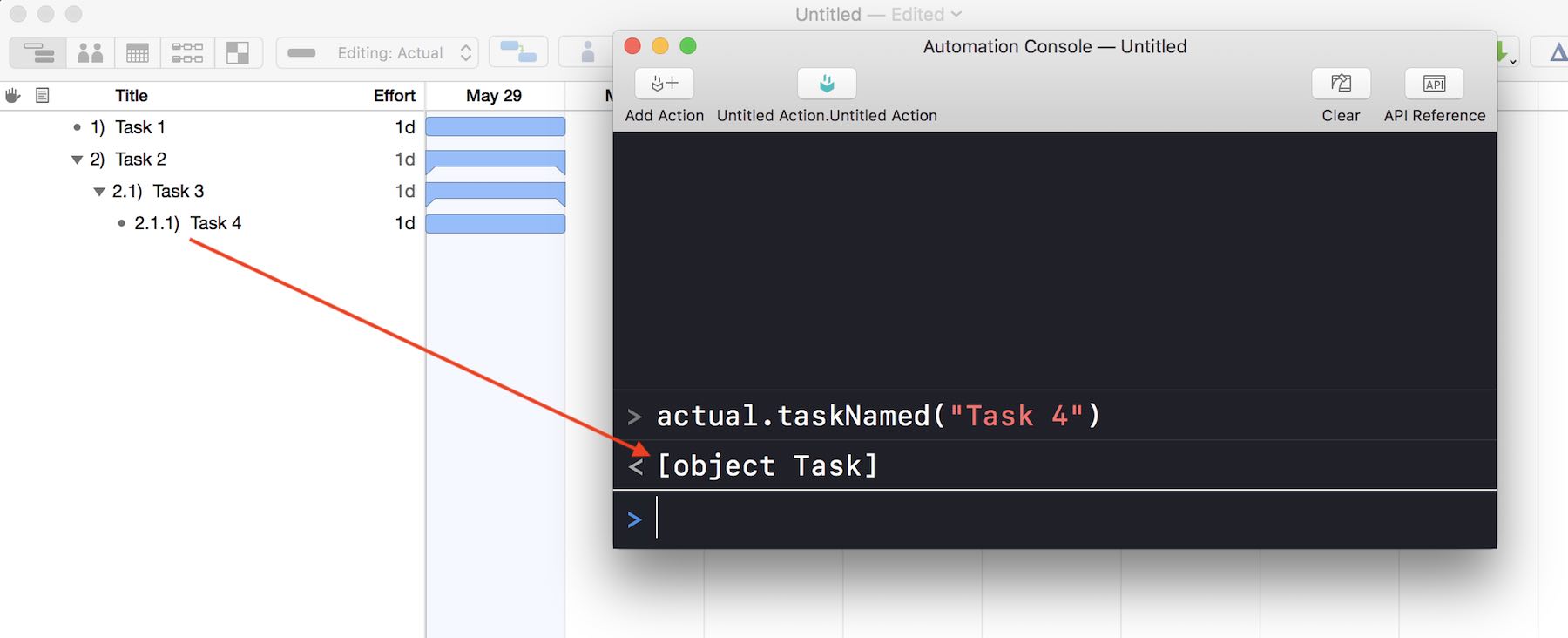
Setting-up a Scenario
Examples of setting up the default scenario (Actual).
Setting-up the main scenario to begin today:
Scenario Begins Today
var sDate = new Date()
sDate = new Date(sDate.setHours(0,0,0,0))
actual.startDate = sDate
actual.hasFixedEndDate = false
actual.hasGenericDates = false
document.project.title = "New Project"
01 Create the start date object for the project by using the standard JavaScript new class constructor to create a new instance of the Date class, describing the current day and time.
02 Use the JavaScript setHours(…) instance function to set the time of the created date object to midnight. The result will be a date reference in milliseconds (eg: 1556866800000), so place the setHours(…) command within a new Date constructor to convert the resulting milliseconds number into a standard Date object. Then, store the resulting Date object in the variable: sDate
03 Set the value of the main scenario’s startDate property to the Date object stored in the variable: sDate
04 Set the value of the main scenario’s hasFixedEndDate property to false, indicating the project should be calculated from the indicated start date/time.
05 Set the value of the main scenario’s hasGenericDates property to false, indicating that non-relative date values should be used.
06 Set the value of the title property of the top-level project class to the desired String value.
Using the DateComponents class of the Calendar class to calculated and set up the main scenario to end in 90 days from today:
Scenario Ends in 90 Days
var cal = Calendar.current
var now = new Date()
var today = cal.startOfDay(now)
var dc = new DateComponents()
dc.day = 90
var targetDate = cal.dateByAddingDateComponents(today, dc)
actual.endDate = targetDate
actual.hasFixedEndDate = true
actual.hasGenericDates = false
document.project.title = "90-Day Wonder"
1 Store a reference to the current Calendar.
2 Store an instance of the current date object.
3 Use the startOfDay() function to set the time of the stored date object to midnight.
4 Store a blank instance of the DateComponents class.
5 Set the value of day property of the new DateComponents instance to 90.
6 Use the dateByAddingDateComponents() function of the DateComponents class to calculate the date object 90 days from the current date.
7 Set the value of the main scenario’s endDate property to the Date object stored in the variable: targetDate
8 Set the value of the main scenario’s hasFixedEndDate property to true, indicating the project should be calculated from the indicated ending date/time.
9 Set the value of the main scenario’s hasGenericDates property to false, indicating that non-relative date values should be used.
10 Set the value of the title property of the top-level project class to the desired String value.
Setting-up the main scenario to end on a specific date:
Project with End Date
actual.hasFixedEndDate = true
actual.hasGenericDates = false // specific
actual.endDate = new Date("7/4/2021")
document.project.title = "4th of July Event"
task = actual.rootTask.addSubtask()
task.title = "1st Planning Session"
task.manualStartDate = new Date("2/1/2021")
task.manualEndDate = new Date("2/2/2021")
task = actual.rootTask.addSubtask()
task.title = "2nd Planning Session"
task.manualStartDate = new Date("3/1/2021")
task.manualEndDate = new Date("3/2/2021")
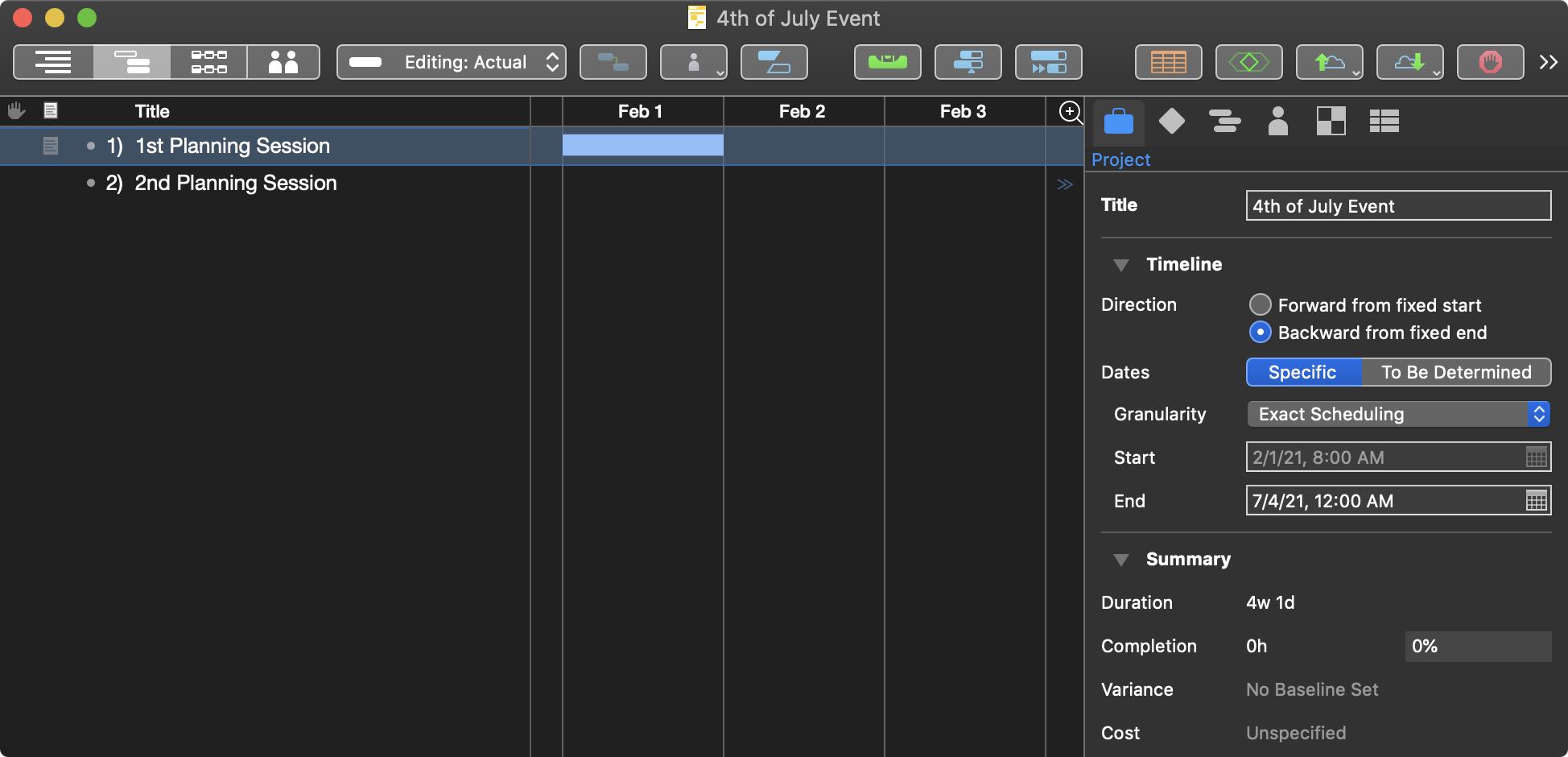
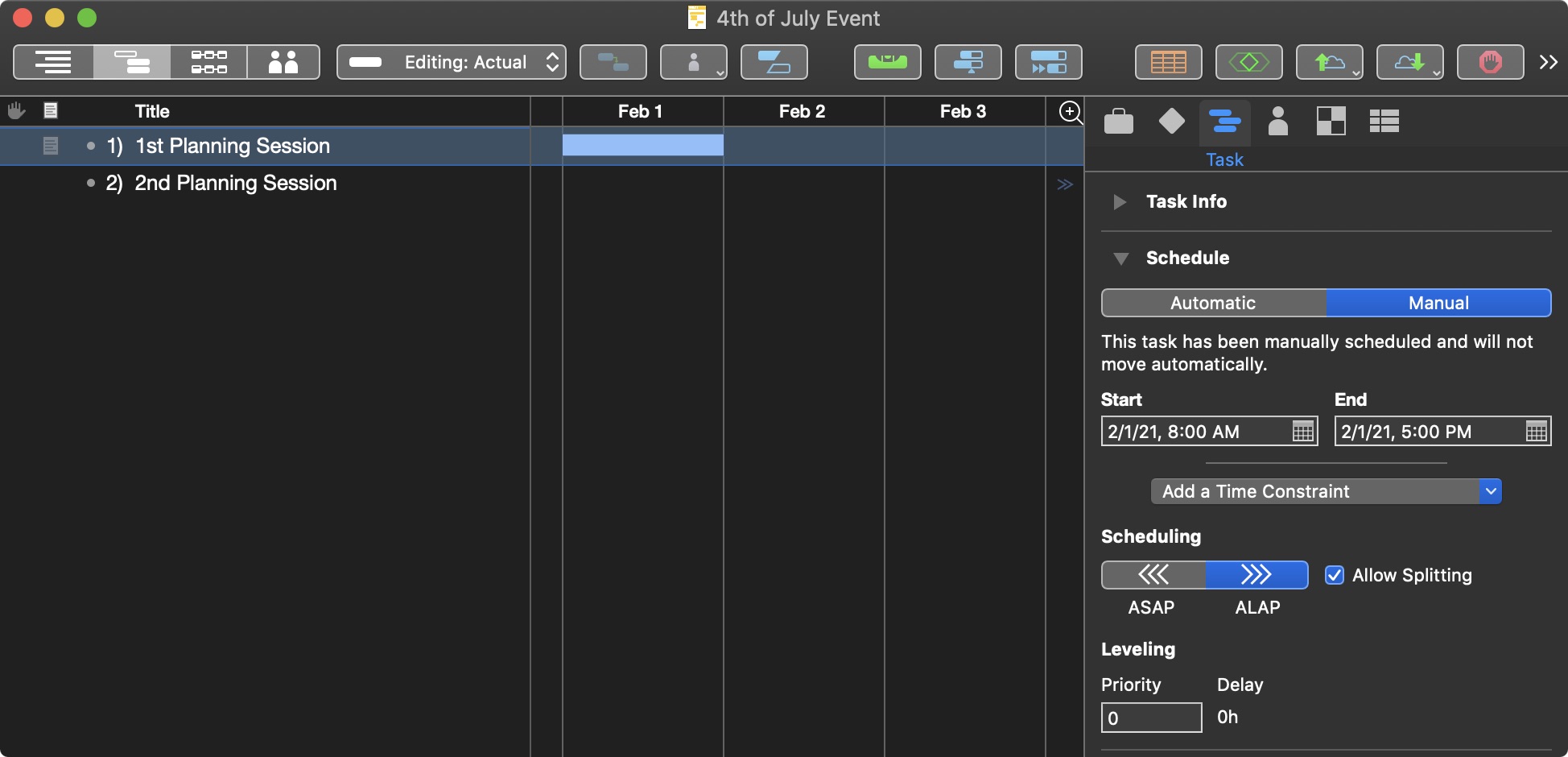