Tags
This section of the tutorial will examine how to create, find, assign, and delete tags — one of the most powerful features of OmniFocus.
In OmniFocus, a tag represents an association that a task has to the world around it. A tag could represent a person, place, or thing most relevant to completion of a project, or it could represent a mindset most applicable to execution of an action item.
An item can have as many tags as you find useful, and there is no specific purpose assigned to them; use tags to assign priority, group items by energy level or required equipment, or don’t use them at all.
To begin, let’s examine where tags are surfaced in the OmniFocus interface.
DO THIS ► | The Tags Perspective displays a list of your tags in the sidebar, and a list of all your actions grouped by the tags they belong to in the outline. Run the following script that sets the value of the perspective property of the Window class so that the Tags Perspective is displayed: |
Show the Tags Perspective
document.windows[0].perspective = Perspective.BuiltIn.Tags
The Tags Perspective will now be displayed in OmniFocus.
TIP: you can use this script to change the currently displayed perspective by replacing the Tags property of the Perspective.BuiltIn class to: Flagged, Forecast, Inbox, Nearby, Projects, Search, or Review
Creating Tags
Instances of the Tag class are created using the standard JavaScript new item constructor.
new Tag(name:String, position:Tag or Tag.ChildInsertionLocation or null) → (Tag r/o) • The new Tag object titled with the provided string. Unless otherwise specified, tags are added to the end of the array of the tags within their parent container.
As with the creation of tasks, the new Tag constructor has a second optional parameter (Tag.ChildInsertionLocation) that indicates where the new tag should be placed when it is created. In this example, the tag’s position in the list of tags is expressed as to whether the new tag is to be added to the start, or the end of the list of existing tags, which is derived as the value of the tags property of the Database class.
DO THIS ► | Enter and run this script for creating a new tag at the start of the list of tags displayed in the Tags Perspective:
|
An object reference to the new tag will be returned in the console, and a new tag will be added to the top (beginning) of the tags list:
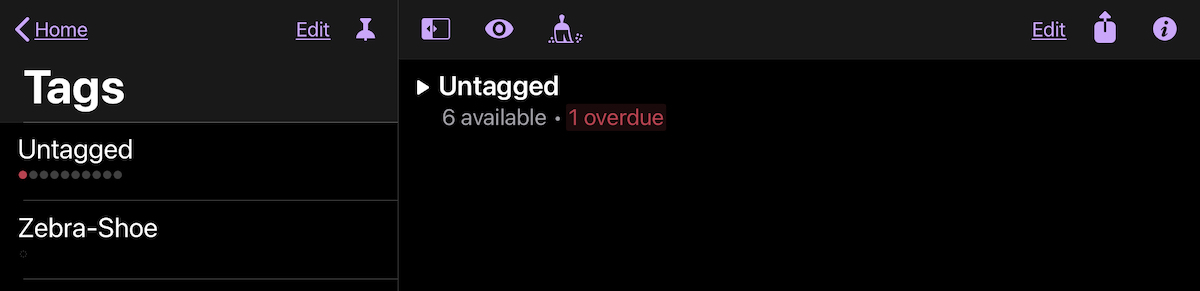
Creating tags is a straightforward process but there is one issue you need to be aware of:
in OmniFocus, tag titles are not required to be unique, and so multiple tags may be created using the same name.
Let’s see if that is true.
DO THIS ► | Enter and run the previous script again:
|
A second tag with the same name will be created.
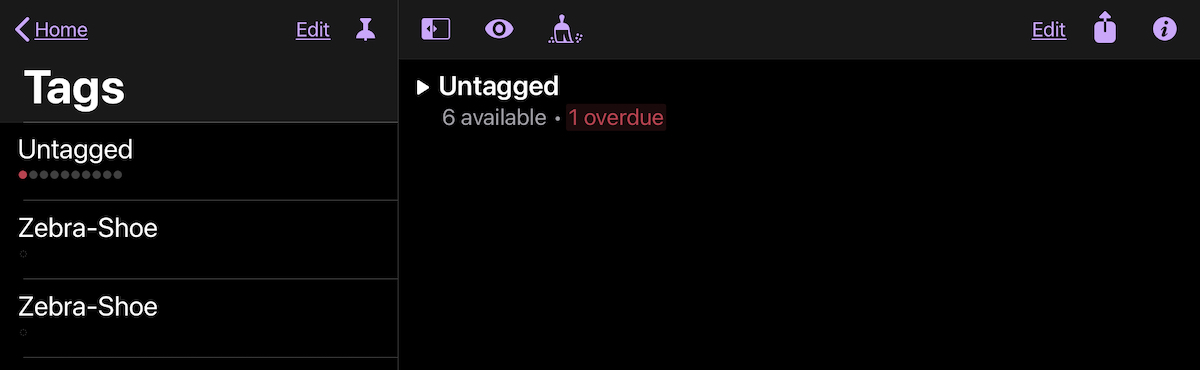
Although both tags share the same name, they possess different identifiers in the OmniFocus database. Their use can create confusion and conflicts, as shown below when a task is selected for tag assignment:
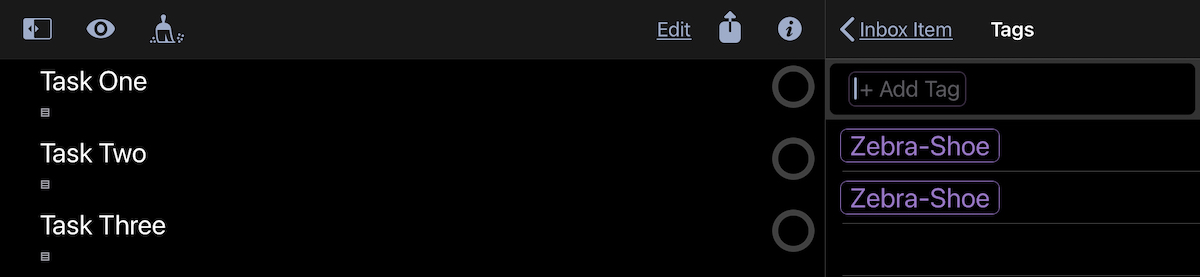
With duplicate tags it is likely that each duplicate tag will be assigned to different tasks or projects, and so the use of a duplicate tag may provide unexpected results.
To ensure that every tag you create is unique, we’ll add a check for existing tags to the creation process. Doing so will ensure that a new tag is created only when a tag with the desired name does not already exist.
But first, let’s delete one of the duplicate tags.
To identify the targeted tag to the script, we’ll retrieve and store an object reference to the tag to delete by using the tagNamed(…) function of the Database class:
DO THIS ► | Enter and run the following script:
|
The result of the tagNamed(…) function will be either an object reference to the first tag whose name matches the provided parameter, or a value of null if no matching tag is located in the top-level set of tags. In our example, an object reference to the first tag will be placed in the variable targetTag which we can then use in another script statement to delete the tag:
DO THIS ► | Enter and run this script that will locate and delete one of the duplicate tag objects:
|
The logic of the second script statement is: if there is a positive result from the previous statement (object reference instead of null) then remove the duplicate tag object from the database by calling the deleteObject(…) function of the Database class and passing the object reference to the tag to remove as the function argument.
With the duplicate tag removed, we can now examine the process for assigning a tag to a task. To assign one or more tags to a task, use either the addTag(…) or addTags(…) instance functions of the Task class.
addTag(tag:Tag) → ( ) • Adds a Tag to this task, appending it to the end of the list of associated tags. If the tag is already present, no change is made. The moveTags(…) function of the Database class can be used to control the ordering of tags within the task.
addTags(tags:TagArray) → ( ) • Adds multiple Tags to this task, appending them to the end of the list of associated tags. For any tags already associated with the Task, no change is made. The moveTags(…) function of the Database class can be used to control the ordering of tags within the task.
Both of these functions require object references to the tag(s) to be assigned to the task, as the function input. So in order to assign a tag to a specific task, we need script statements that return an object reference to a tag specified by name.
We’ve already used the tagNamed(…) function of the Database class to locate a top-level tag by name. We can use this function again, but what happens if the tag we’re looking for doesn’t exist? Is it possible to have a script statement that returns an object reference to a tag specified by name, even if does not exist?
The following script statement will return an object reference to an existing tag specified by name, but if there is not one, will create a new tag using the specified name:
Object Reference for Tag Specified by Name
var tagObj = flattenedTags.byName("Zebra-Shoe") || new Tag("Zebra-Shoe")
The script uses the byName() function with the flattenedTags property of the Database class to search the entire list of all tags in the database to reference the first tag it finds that matches the given name. If no matching tag is found, a null value is returned as the result.
The JavaScript logical operator (||) indicates that if the first part of the script statement (up to the bars) does not return a positive result (an object reference to a tag), then the second part of the script statement (following the bars) will be executed, creating a new instance of the Tag class.
Either way, the result of this single script statement will be an object reference to the the tag named “Zebra-Shoe” and will be stored in the variable: tagObj
Using this conditional script statement, let’s generate an object reference to a tag with a specified name, and then assign the tag to the previously created task: “Staff Meeting”
DO THIS ► | Enter and run the following script. (Reminder: type Option-Return to add a new line to a script in the Console without evoking the script.) |
Assign Tag to Task
var tagName = "Attendance Required"
var tagObj = flattenedTags.byName(tagName) || new Tag(tagName)
var taskObj = inbox.byName("Staff Meeting") || new Task("Staff Meeting")
taskObj.addTag(tagObj)
document.windows[0].perspective = Perspective.BuiltIn.Inbox
document.windows[0].selectObjects([taskObj])
The task “Staff Meeting” will now have the newly created tag “Attendance Required” assigned to it:
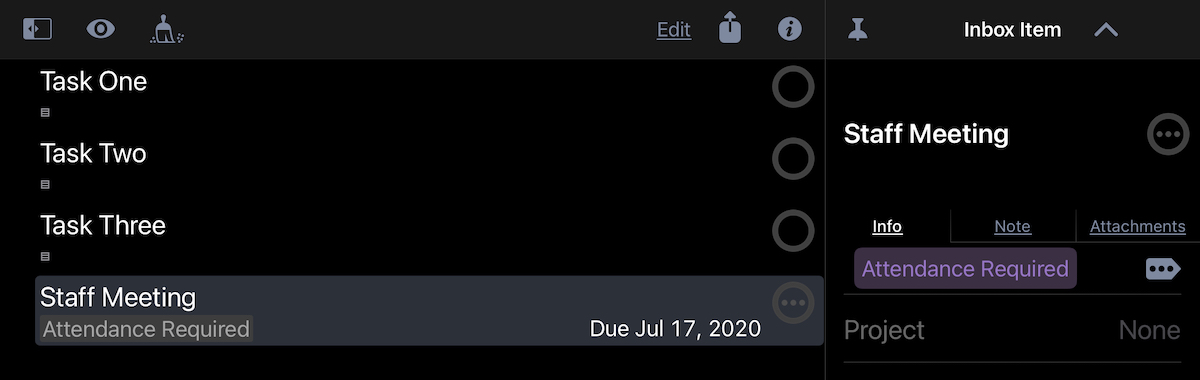
TIP: Use the same technique in your scripts whenever you need to generate an object reference to a unique tag.
Object Reference for Tag Specified by Name
var tagName = "name of tag"
var tagObj = flattenedTags.byName(tagName) || new Tag(tagName)
Summary
In this section you learned:
Using the perspective property of the Window class, you can change the perspective currently displayed in OmniFocus.
You learned how to create, reference, and assign tags to tasks.
In the next section, you will learn how to create and manipulate projects.