Plug-In: Schedule Medication
This plug-in will create a project with sequential tasks for tracking doses during the course of a medication.
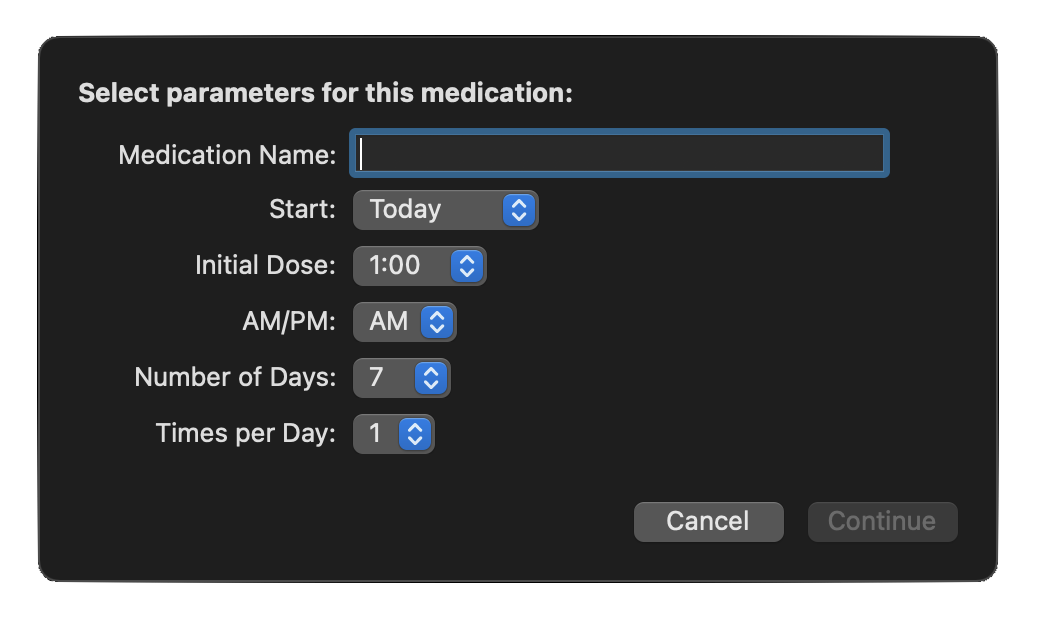
NOTE: The OmniFocus Voice Commands Collection contains a matching voice command for this plug-in.
Return to: OmniFocus Plug-In Collection
Schedule Medication
/*{
"type": "action",
"targets": ["omnifocus"],
"author": "Otto Automator",
"identifier": "com.omni-automation.of.schedule-medication-course",
"version": "1.2",
"description": "Creates a project with sequential tasks for tracking the course of a medication.",
"label": "Schedule Medication",
"shortLabel": "Schedule Medication",
"paletteLabel": "Schedule Medication",
"image": "calendar.badge.clock"
}*/
(() => {
const action = new PlugIn.Action(async function(selection, sender){
try {
projectTitleField = new Form.Field.String(
"projectTitle",
"Medication Name",
null,
null
)
startDayMenu = new Form.Field.Option(
"startDay",
"Start",
["Today", "Tomorrow"],
["Today", "Tomorrow"],
"Today"
)
times = ["1:00", "2:00", "3:00", "4:00", "5:00", "6:00", "7:00", "8:00", "9:00", "10:00", "11:00", "12:00"]
timeIndexes = times.map((item, index) => index)
timesMenu = new Form.Field.Option(
"startTime",
"Initial Dose",
times,
times,
"1:00"
)
meridiemMenu = new Form.Field.Option(
"meridiemOption",
"AM/PM",
["AM", "PM"],
["AM", "PM"],
"AM"
)
courseDurationMenu = new Form.Field.Option(
"courseDuration",
"Number of Days",
["5", "7", "10", "12", "28", "30"],
["5", "7", "10", "12", "28", "30"],
"7"
)
timesPerDayMenu = new Form.Field.Option(
"timesPerDay",
"Times per Day",
["1", "2", "3", "4"],
["1", "2", "3", "4"],
"1"
)
inputForm = new Form()
inputForm.addField(projectTitleField)
inputForm.addField(startDayMenu)
inputForm.addField(timesMenu)
inputForm.addField(meridiemMenu)
inputForm.addField(courseDurationMenu)
inputForm.addField(timesPerDayMenu)
inputForm.validate = function(formObject){
projectTitle = formObject.values['projectTitle']
return (!projectTitle) ? false:true
}
formPrompt = "Select parameters for this medication:"
buttonTitle = "Continue"
formObject = await inputForm.show(formPrompt, buttonTitle)
projectTitle = formObject.values["projectTitle"]
startDay = formObject.values["startDay"]
startTime = formObject.values["startTime"]
meridiemOption = formObject.values["meridiemOption"]
targetDate = new Date()
if (startDay === "Tomorrow"){
targetDate = new Date(targetDate.setHours(24, 0, 0, 0))
}
dateString = targetDate.toLocaleDateString()
startDueDate = new Date(dateString + " " + startTime + " " + meridiemOption)
courseDuration = Number(formObject.values["courseDuration"])
courseDurationString = String(courseDuration)
timesPerDay = Number(formObject.values["timesPerDay"])
timeInterval = 24 / timesPerDay
timeIntervalString = String(timeInterval)
dosageCount = courseDuration * timesPerDay
dosageCountString = String(dosageCount)
dc = Calendar.current.dateComponentsFromDate(startDueDate)
dc.day = dc.day + courseDuration
completionDate = Calendar.current.dateFromDateComponents(dc)
completionDateString = completionDate.toLocaleDateString()
project = new Project(`${projectTitle} (${courseDurationString}-day to ${completionDateString})`)
project.sequential = true
project.completedByChildren = true
taskDueDateTime = startDueDate.getTime()
millisecondsToAdd = timeInterval * 3600000
for (i = 0; i < dosageCount; i++) {
task = new Task(`Dose ${i + 1}`, project)
if (i !== 0){
taskDueDateTime = taskDueDateTime + millisecondsToAdd
}
newDueDate = new Date(taskDueDateTime)
task.dueDate = newDueDate
}
document.windows[0].perspective = Perspective.BuiltIn.Projects
document.windows[0].focus = [project]
}
catch(err => {
if (!err.causedByUserCancelling){
new Alert(err.name, err.message).show()
}
})
});
action.validate = function(selection, sender){
return true
};
return action;
})();