Console Toolbar (macOS)
The Console’s Toolbar provides an excellent access point for plug-ins that interact with the Console. For example, adding a plug-in that logs the properties of the selected item, to the Console Toolbar, provides a quick one-click way to view all of the attributes of a selected item.
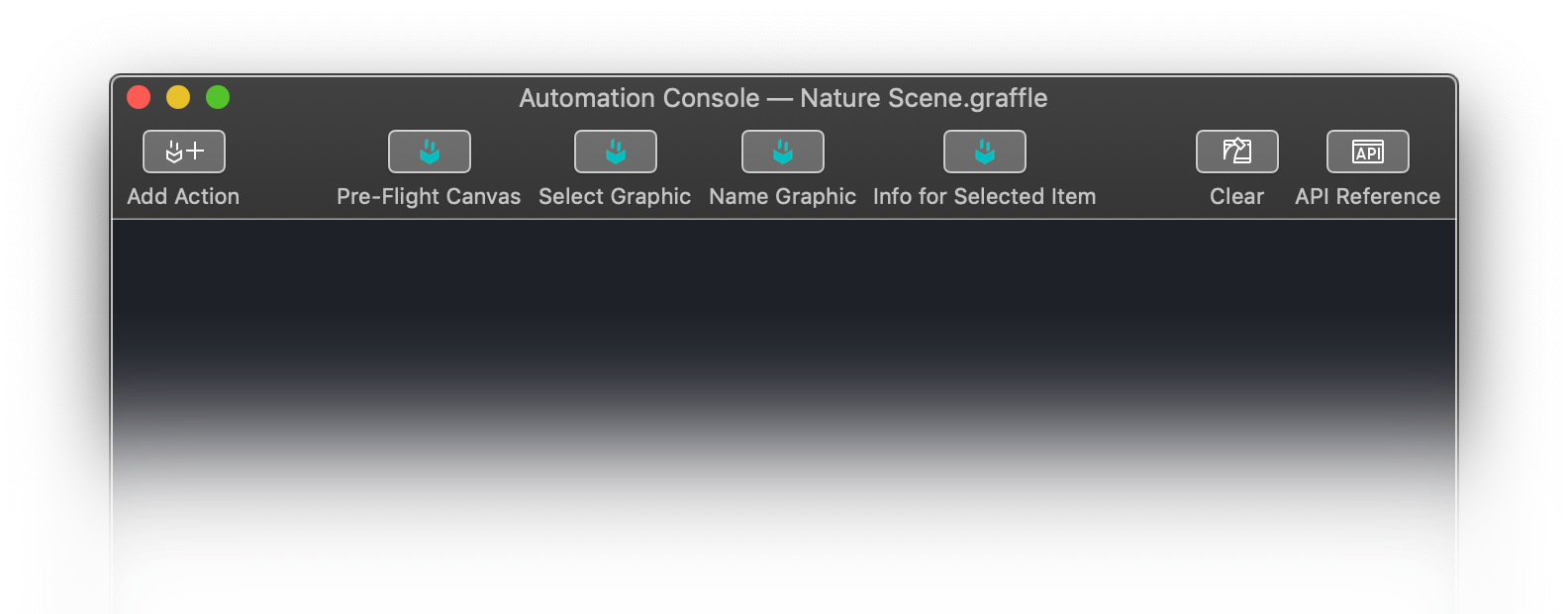
Adding Plug-Ins to Toolbar
To add, reorder, or remove plug-ins to or from the Console Toolbar, follow these steps:
- Control-click (right-click) the Console Toolbar to summon the Toolbar contextual menu.
- Select the “Customize Toolbar…” option from the menu.
- The forthcoming sheet will display rows of icons for all of the installed plug-ins.
- You may add plug-ins to the Toolbar by dragging their icons to the Toolbar. Existing Toolbar plug-ins can be re-ordered or removed by dragging their icons off the Toolbar. Plug-ins removed from the Toolbar will stil remain installed and available.
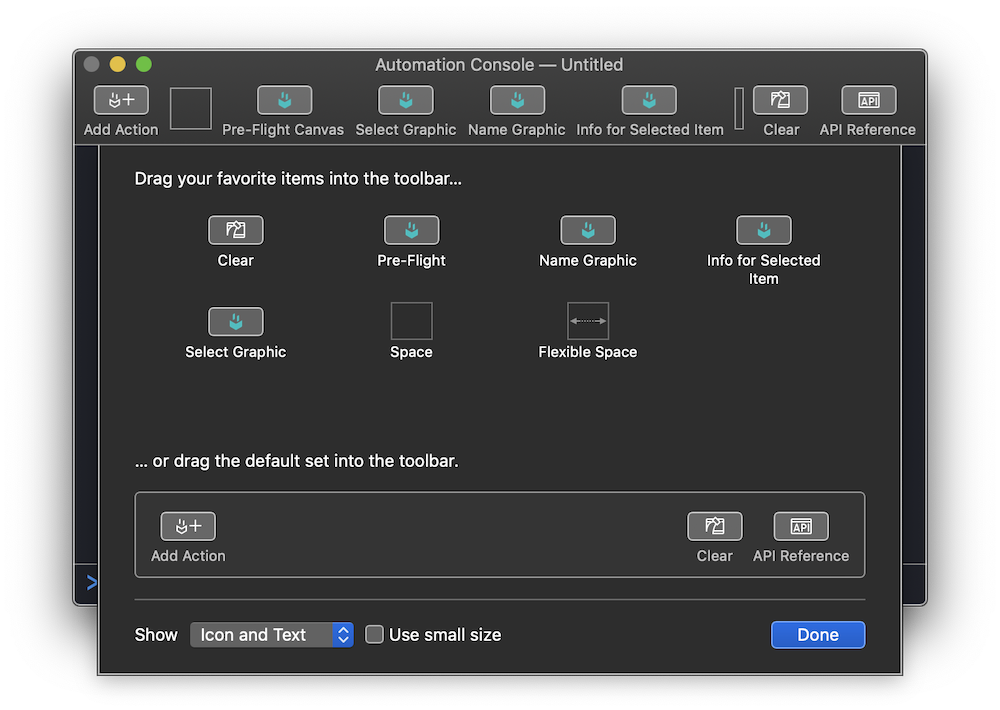
The following video demonstrates how plug-ins may be added to the Console Toolbar and used to interact with a document (OmniGraffle):
|
“Pre-Flight” Plug-Ins
As shown in the video, this group of plug-ins can be used to scan and interact with the graphics in an OmniGraffle document, in order to check for potential issues before printing.
The “Pre-Flight Current Canvas” plug-in scans all of the graphics on the current canvas to reveal any that are unnamed or are set to be non-printing. You can edit the plug-in to include any specific checks to fit your requirements.
Canvas Pre-Flight
/*{
"type": "action",
"targets": ["omnigraffle"],
"identifier": "com.omni-automation.og.pref-flight-canvas",
"version": "1.0",
"author": "Otto Automator",
"description": "Checks the current canvas for unnamed or non-printing graphics.",
"label": "Pre-Flight Current Canvas",
"shortLabel": "Pre-Flight Canvas",
"paletteLabel": "Pre-Flight",
"image": "checkmark.circle"
}*/
(() => {
const action = new PlugIn.Action(function(selection, sender){
// action code
// selection options: canvas, document, graphics, lines, solids, view
console.clear()
let cnvs = document.windows[0].selection.canvas
let cnvsName = cnvs.name
cnvs.graphics.forEach(function(graphic, index){
let obj = new Object()
obj.canvasName = cnvsName
obj.layerName = graphic.layer.name
obj.name = graphic.name
obj.id = graphic.id
obj.type = graphic instanceof Line ? "Line" : graphic.shape
if (obj.type != "Line"){
obj.image = graphic.image instanceof ImageReference ? true : false
} else {
obj.image = false
}
obj.locked = graphic.locked
obj.prints = graphic.layer.prints
let objStr = JSON.stringify(obj)
if (obj.prints === false){
console.warn("Non-Printing Graphic " + String(index), objStr)
} else if (obj.name === null){
console.warn("Unnamed Graphic " + String(index), objStr)
} else {
console.info("Graphic " + String(index), objStr)
}
})
});
action.validate = function(selection, sender){
// validation code
// selection options: canvas, document, graphics, lines, solids, view
return true
};
return action;
})();
Safety Wrapper Plug-In
To avoid issues with the re-use of declared variables in the Console, here's a plug-in for the Console toolbar that when activated, will place a self-invoking code wrapper on the clipboard for you to paste into the Console. Put your code within the wrapper to avoid any namespace conflicts.
Code Wrapper
/*{
"type": "action",
"targets": ["omnifocus","omniplan","outliner","omnigraffle"],
"author": "Otto Automator",
"identifier": "com.omni-automation.all.code-wrapper",
"version": "1.0",
"description": "Places a code safety wrapper on the clipboard for pasting into the Console.",
"label": "Code Wrapper",
"paletteLabel": "Code Wrapper"
"image": "chevron.left.slash.chevron.right"
}*/
(() => {
const action = new PlugIn.Action(function(selection, sender){
// action code
wrapper = `(() => {
// CODE GOES HERE
})();`
Pasteboard.general.string = wrapper
});
action.validate = function(selection, sender){
// validation code
return true
};
return action;
})();
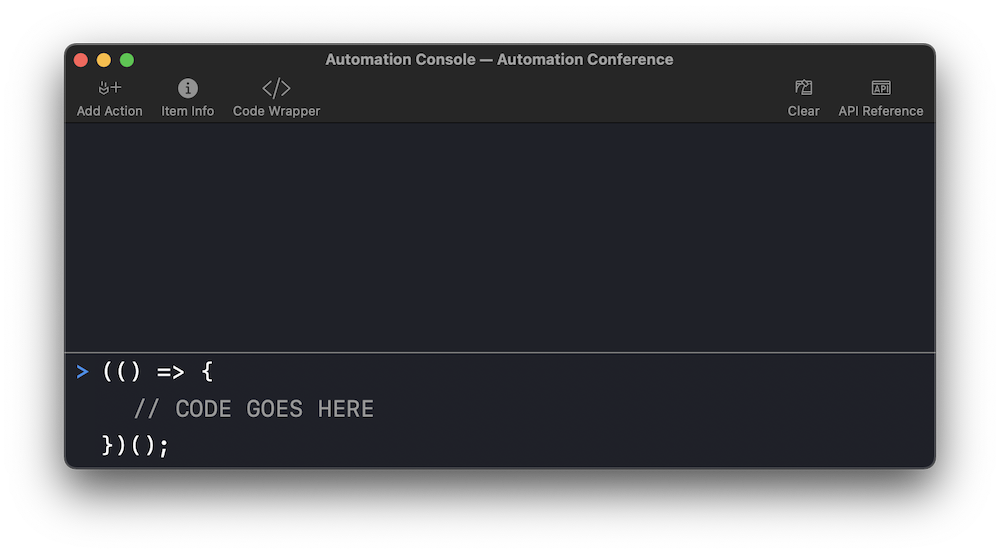
Info for Selected OmniFocus Item
This plug-in logs to the Console all of the property names and values for the selected OmniFocus database object: folder, project, task, or tag
Info for Selected Item (OmniFocus)
/*{
"author": "Otto Automator",
"targets": ["omnifocus"],
"type": "action",
"identifier": "com.omni-automation.of.info-for-selected-item",
"version": "1.0",
"description": "Logs to the console all of the property names and values for the selected database object.",
"label": "Info for Selected Item",
"shortLabel": "Item Info",
"paletteLabel": "Item Info",
"image": "info.circle.fill"
}*/
(() => {
const action = new PlugIn.Action(function(selection) {
let objRef = selection.databaseObjects[0]
let propertyNames = Object.getOwnPropertyNames(objRef)
propertyNames = propertyNames.sort()
console.clear()
propertyNames.forEach(pname => {
console.log("• " + pname + ":", objRef[pname])
})
});
action.validate = function(selection){
return (selection.databaseObjects.length === 1)
};
return action;
})();
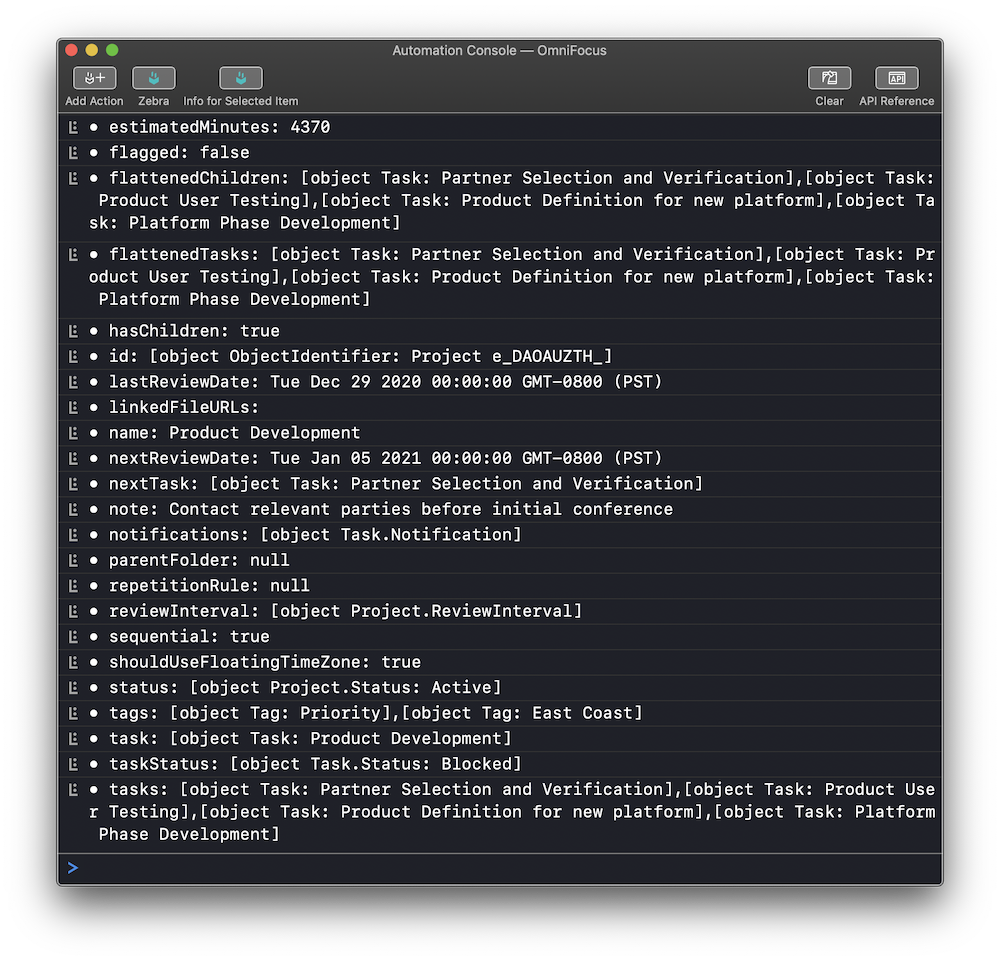
Info for Selected OmniGraffle Item
This plug-in logs to the Console all of the property names and values for the selected OmniGraffle graphic object.
Info for Selected Item (OmniGraffle)
/*{
"type": "action",
"targets": ["omnigraffle"],
"author": "Otto Automator",
"identifier": "com.omni-automation.og.info-for-selected-item",
"version": "1.0",
"description": "Logs to the console all of the property names and values for the selected database object.",
"label": "Info for Selected Item",
"shortLabel": "Item Info",
"paletteLabel": "Item Info",
"image": "info.circle.fill"
}*/
(() => {
const action = new PlugIn.Action(function(selection) {
let objRef = selection.graphics[0]
let propertyNames = Object.getOwnPropertyNames(objRef)
propertyNames = propertyNames.sort()
console.clear()
propertyNames.forEach(pname => {
console.log(pname + " • ", objRef[pname])
})
});
action.validate = function(selection, sender){
// validation code
// selection options: canvas, document, graphics, lines, solids, view
return (selection.graphics.length === 1)
return true
};
return action;
})();
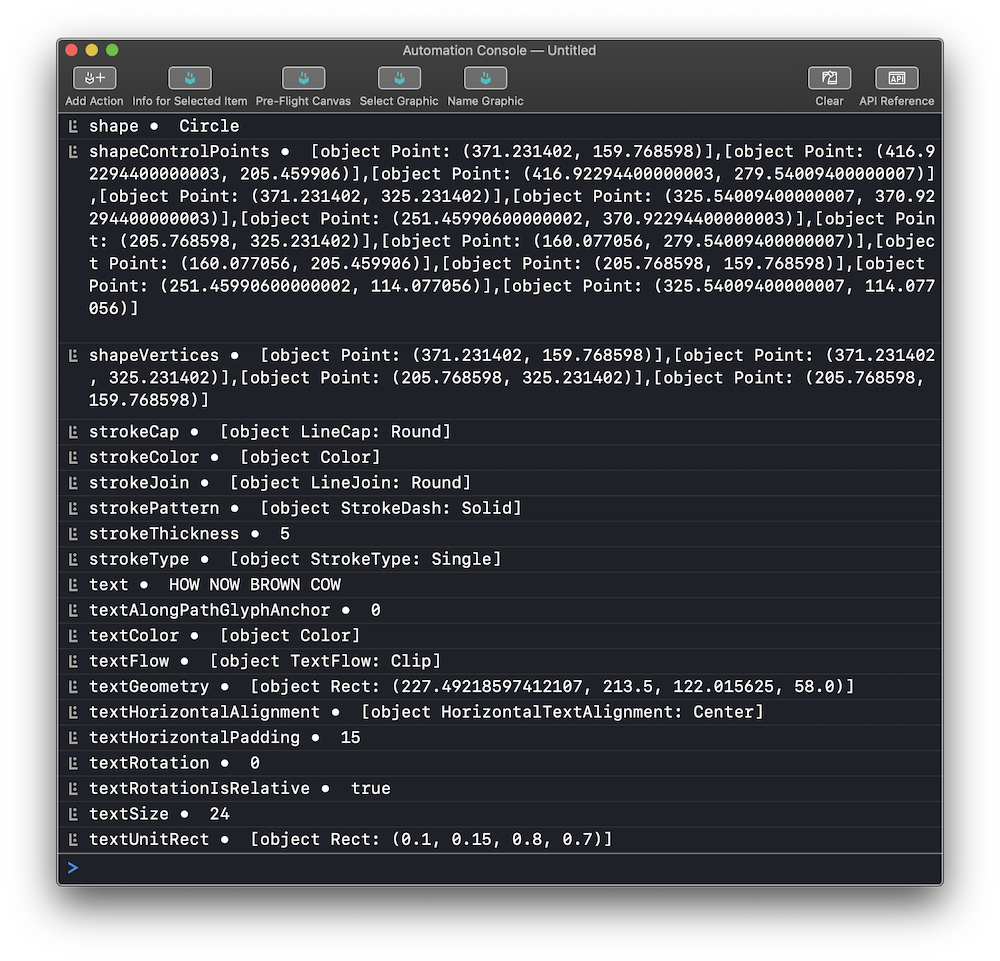
Info for Selected OmniPlan Item
This plug-in logs to the Console all of the property names and values for the selected OmniPlan resource or task.
Info for Selected Item (OmniPlan)
/*{
"type": "action",
"targets": ["omniplan"],
"author": "Otto Automator",
"identifier": "com.omni-automation.op.info-for-selected-item",
"version": "1.0",
"description": "Logs to the console all of the property names and values for the selected task or resource.",
"label": "Info for Selected Item",
"shortLabel": "Item Info",
"paletteLabel": "Item Info",
"image": "info.circle.fill"
}*/
(() => {
const action = new PlugIn.Action(function(selection) {
var selection = document.windows[0].selection
if (selection.tasks.length === 1){
var objRef = selection.tasks[0]
} else {
var objRef = selection.resources[0]
}
var propertyNames = Object.getOwnPropertyNames(objRef)
propertyNames = propertyNames.sort()
console.clear()
propertyNames.forEach(pname => {
console.log(pname + " • ", objRef[pname])
})
});
action.validate = function(selection, sender){
// validation code
// selection options: project, tasks, resources
var selection = document.windows[0].selection
return ((selection.tasks.length === 1 || selection.resources.length === 1) ? true:false)
};
return action;
})();
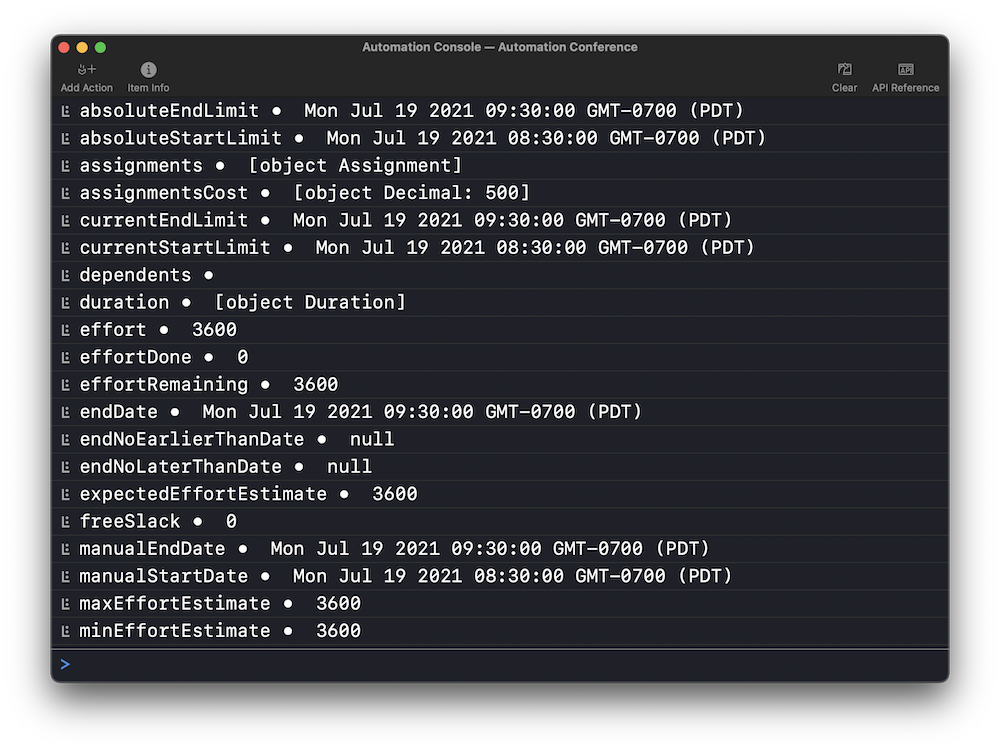
MORE: See the next page about the Console Toolbar Add Action button for details about how to quickly create plug-ins for the Omni application and Console.