Console Functions
In addition to providing a means for running Omni Automation scripts, an Omni application’s console window allows scripts to “log” debugging, warning, or error information where it can be viewed in the system console or in the console output area. A single instance of the Console class is available to scripts as the console global variable.
Here are the functions supported by the Console:
console.log()
log(message:Object, additional:Array of Object or null) (Boolean) • Appends a line to the application console formed by concatenating the given message (after converting it to a String), any additional arguments separated by spaces, and finally a newline.
A simple logging of a text string:
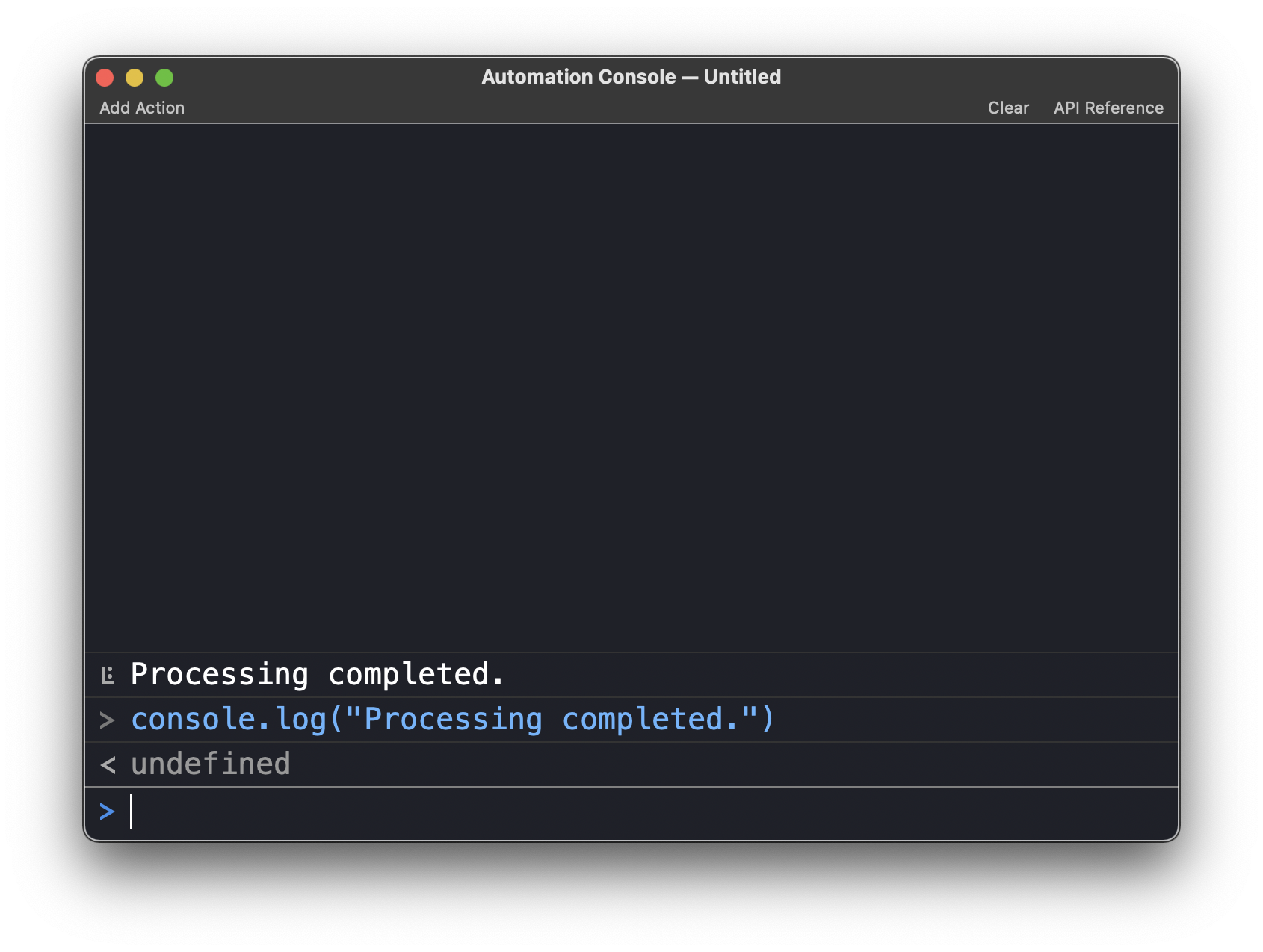
An example using the (optional) “additional” parameter to include the shape of the item being processed in the log statement:
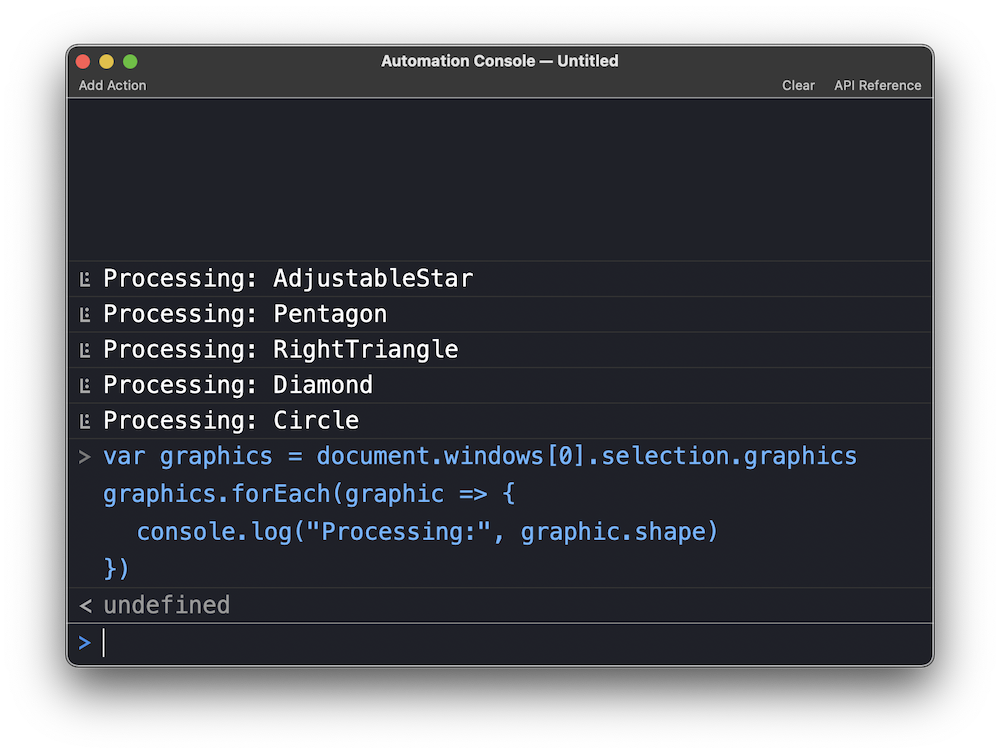
Another example passing in an object rather than a string:
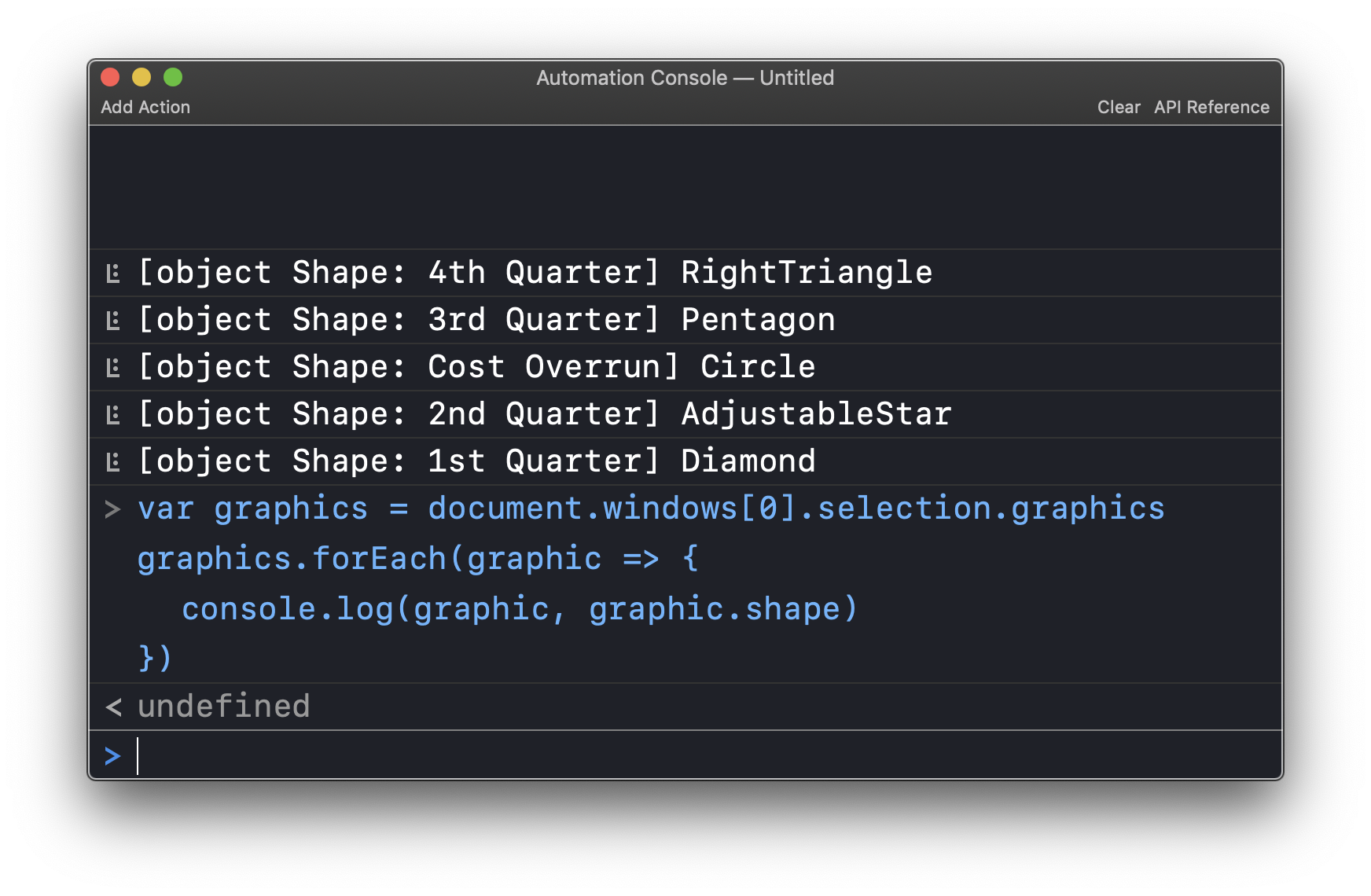
console.error()
error(message:Object, additional:Array of Object or null) (Boolean) • Similar in function to the log function, except the log statement is highlighted as an error. Note that logging a console error does not stop the script. To stop a script use the throw command.
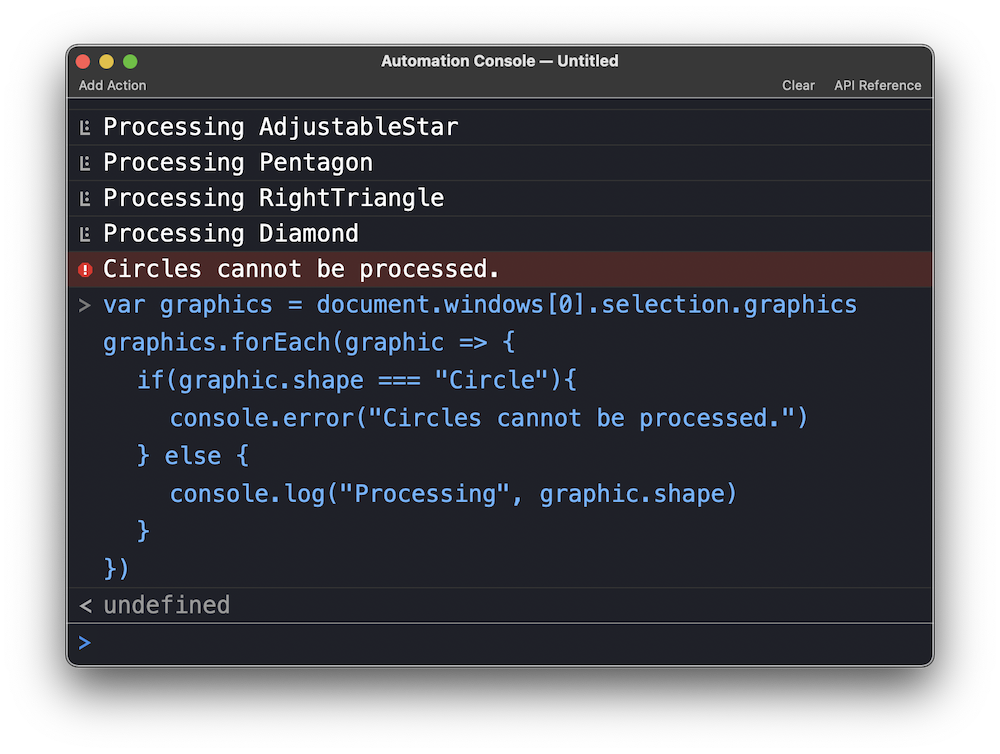
Using the (optional) “additional” parameter to include unique identifying information about the object causing the error:
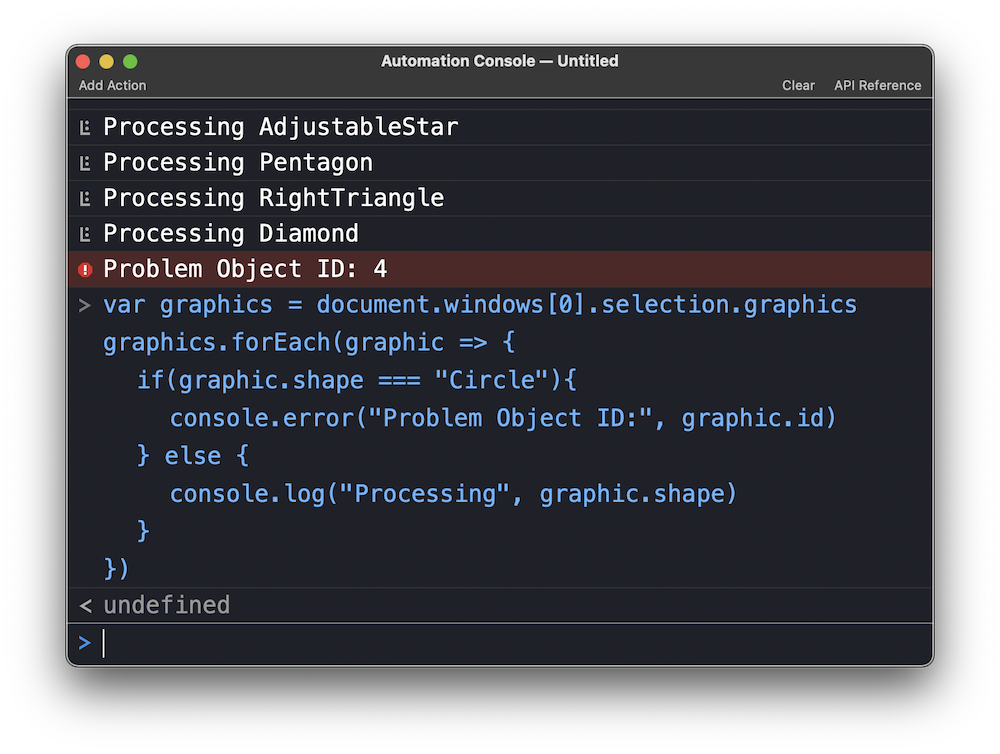
console.info()
info(message:Object, additional:Array of Object or null) (Boolean) • Similar in function to the log function, except the log statement is highlighted as informational.
An informational example that creates and displays an informational data dictionary for each processed object:
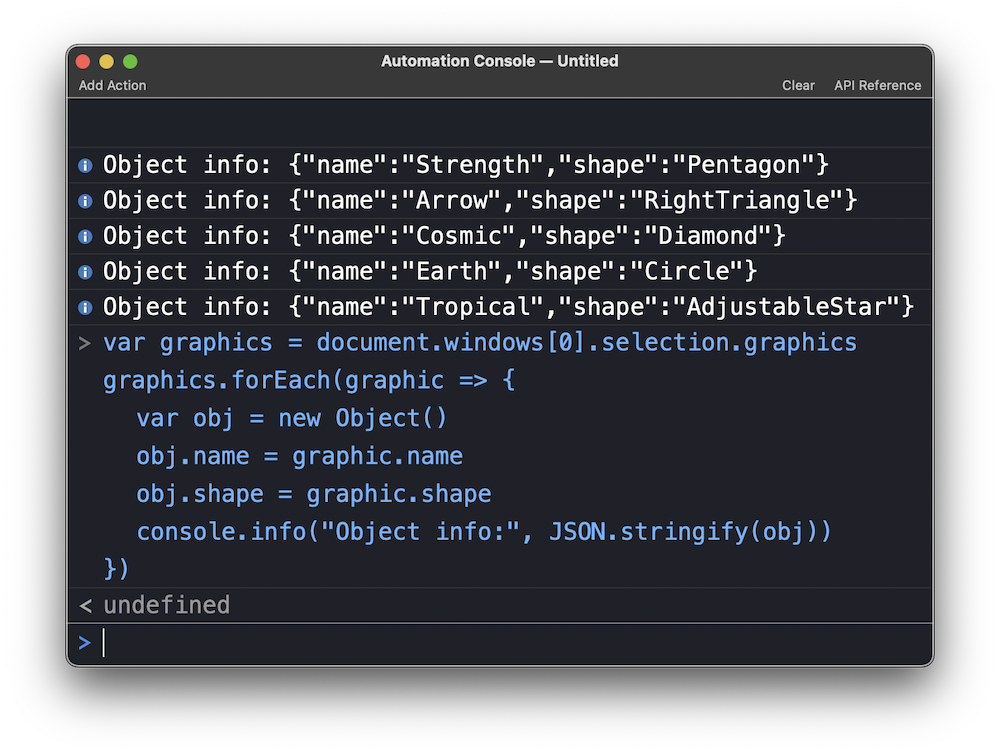
console.warn()
warn(message:Object, additional:Array of Object or null) (Boolean) • Similar in function to the log function, except the log statement is highlighted as warning.
Posting a warning about graphic names containing spaces:
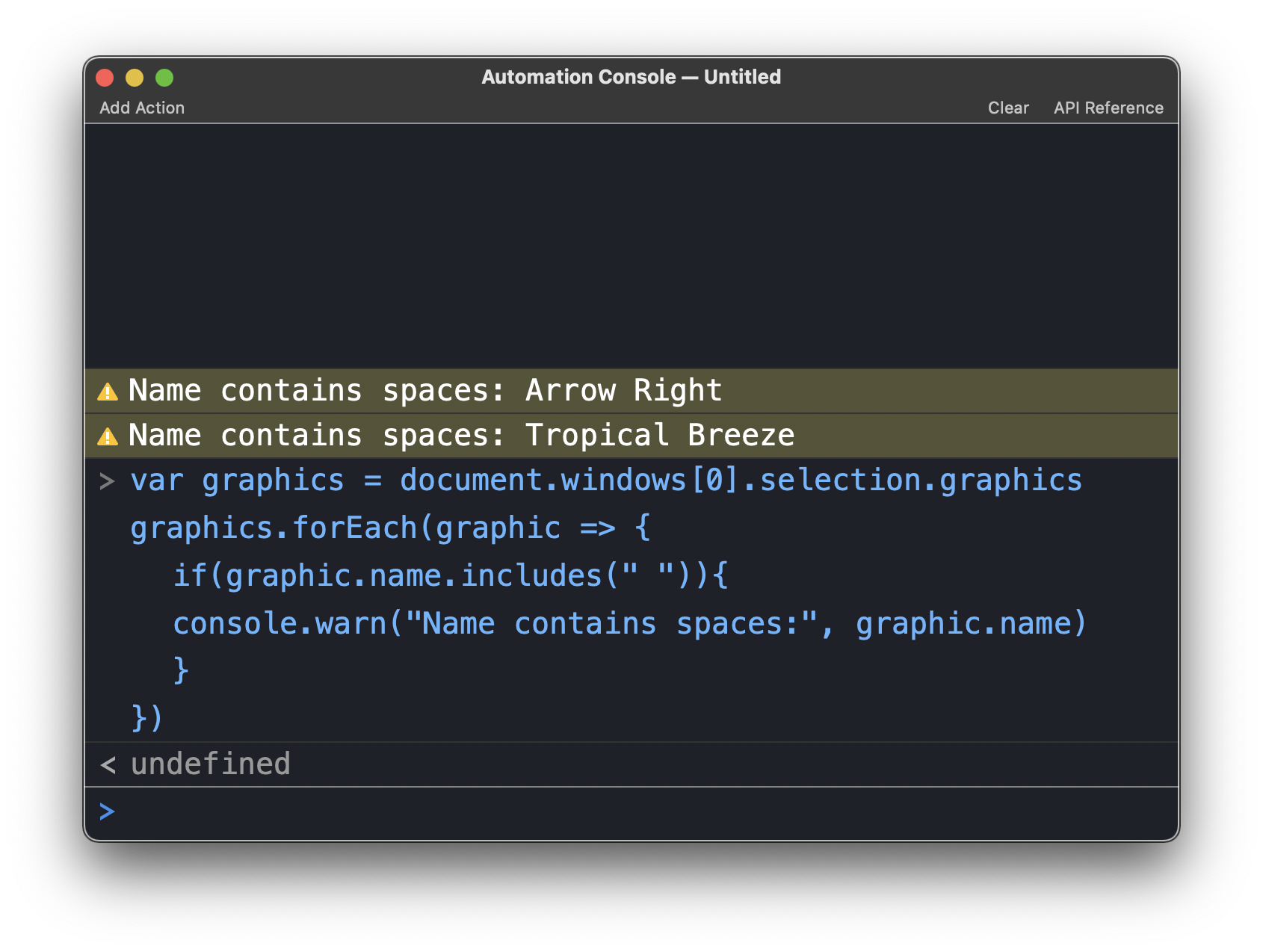
console.clear()
clear() Clears the console in the user-visible window.
Placing the console.clear() function at the start of the script will remove previous messaging from the console window and only display the results of the current script:
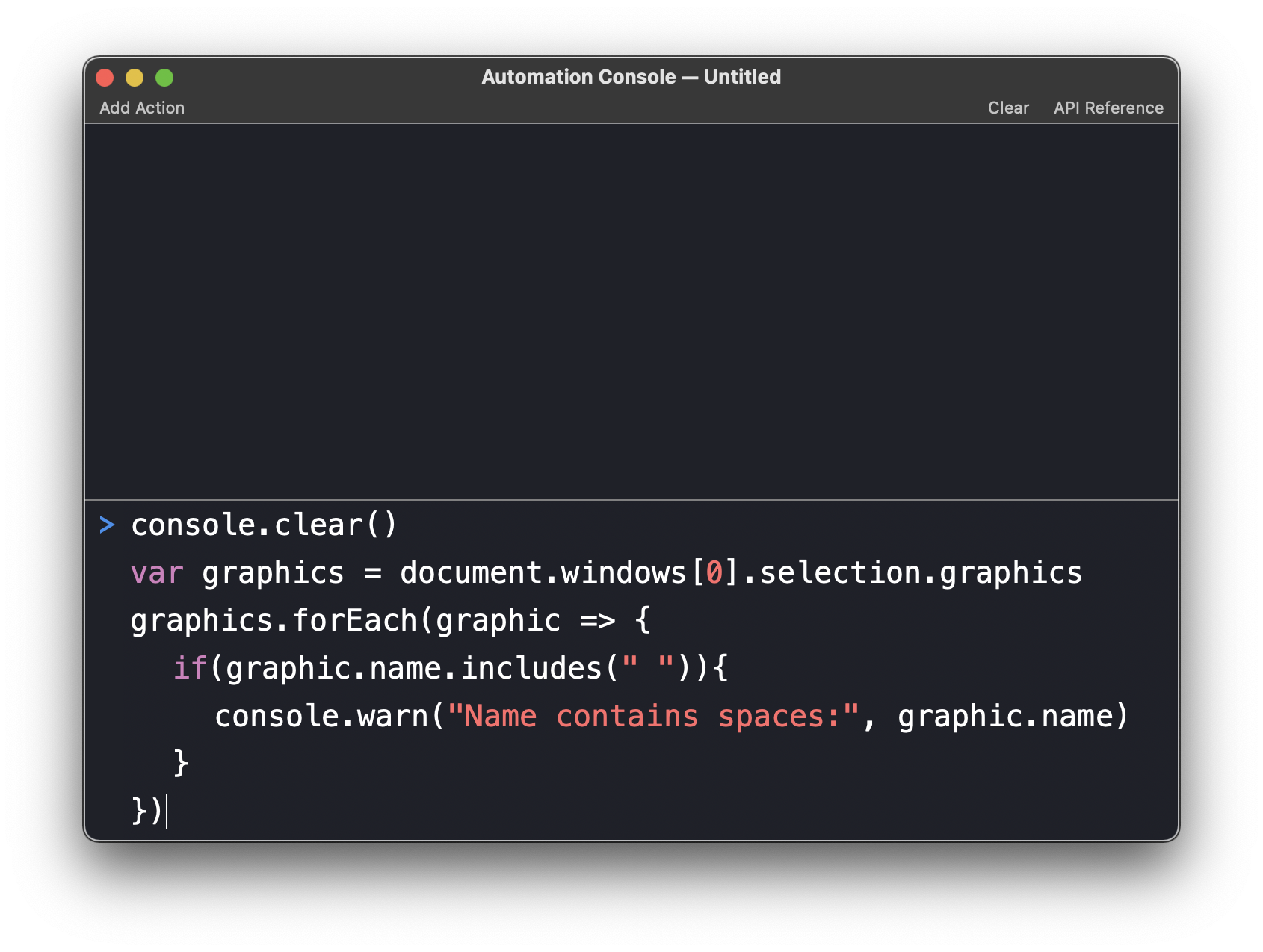
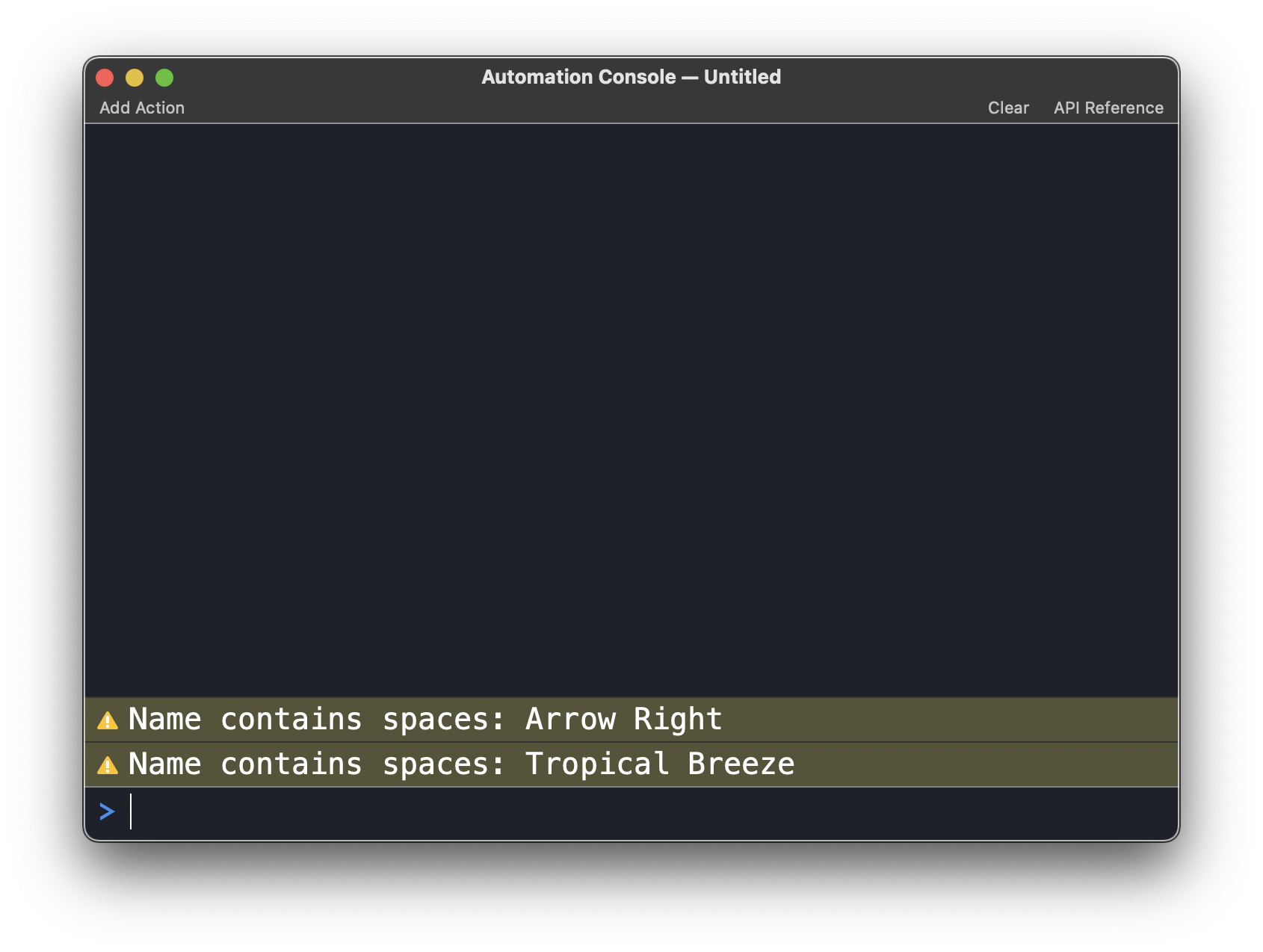
Graphic Summary
Here’s an example of using console logging to review the status of the graphics in the current canvas. Run the script with the OmniGraffle console window open to view the results.
Note the use of JavaScript Ternary operators in lines 8 and 10:
NOTE: This page contains interactive script examples that are executed only with your approval. See the section on Script Security for details about allowing the execution of remote scripts.Graphics Summary
console.clear()
var graphics = document.windows[0].selection.canvas.graphics
graphics.forEach(function(graphic, index){
var obj = new Object()
obj.layerName = graphic.layer.name
obj.name = graphic.name
obj.id = graphic.id
obj.type = graphic instanceof Line ? "Line" : graphic.shape
if (obj.type != "Line"){
obj.image = graphic.image instanceof ImageReference ? true : false
} else {obj.image = false}
obj.locked = graphic.locked
console.log("Graphic " + String(index),JSON.stringify(obj))
})
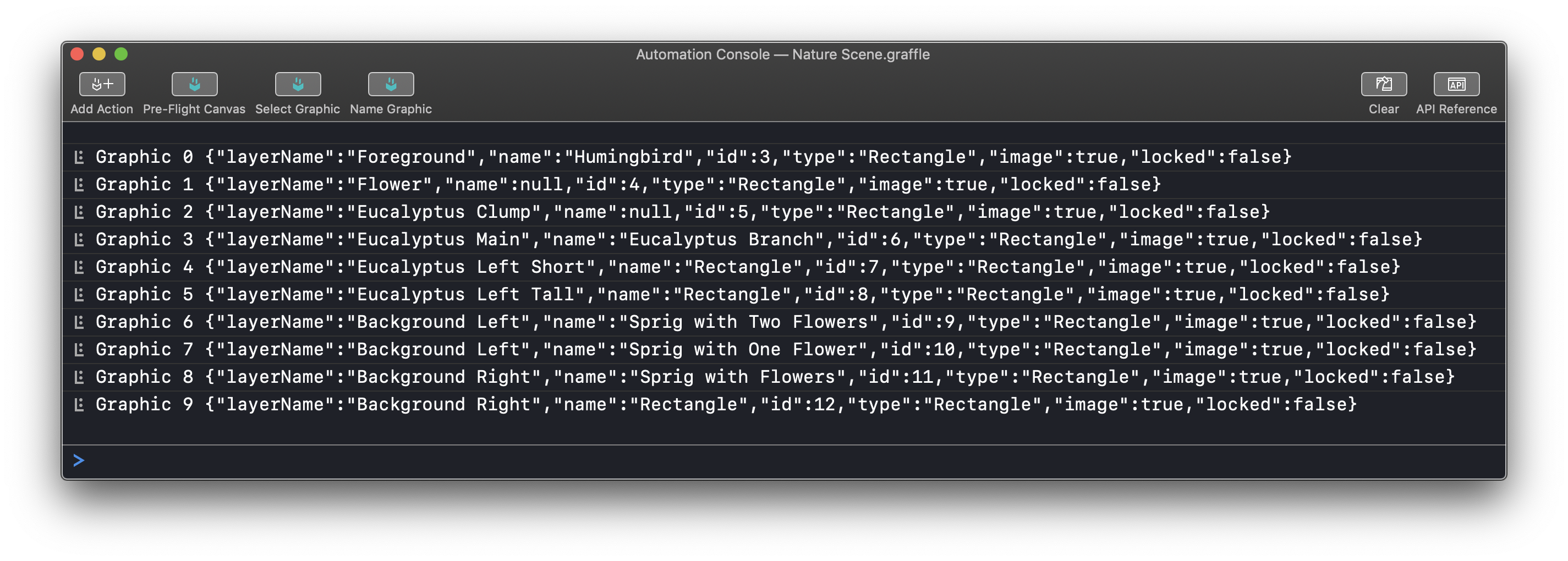
“Pre-Flight” Documents
Here’s an example of using the console to Pre-flight a document prior to saving, printing, or processing. If installed as an action, the results will log to the application’s console window when the action is selected from the Automation menu.
This example script checks for graphics that are unnamed or are set to not print. You can edit the script to check for the document or graphic properties you require.
Flight-Check Canvas
console.clear()
var cnvs = document.windows[0].selection.canvas
var cnvsName = cnvs.name
cnvs.graphics.forEach(function(graphic, index){
var obj = new Object()
obj.canvasName = cnvsName
obj.layerName = graphic.layer.name
obj.name = graphic.name
obj.id = graphic.id
obj.type = graphic instanceof Line ? "Line" : graphic.shape
if (obj.type != "Line"){
obj.image = graphic.image instanceof ImageReference ? true : false
} else {
obj.image = false
}
obj.locked = graphic.locked
obj.prints = graphic.layer.prints
var objStr = JSON.stringify(obj)
if (obj.prints === false){
console.warn("Non-Printing Graphic " + String(index),objStr)
} else if (obj.name === null){
console.warn("Unnamed Graphic " + String(index),objStr)
} else {
console.info("Graphic " + String(index),objStr)
}
})
|
MORE: See the next page about the Console Toolbar for more detail about the pre-flight example.