OmniFocus: New Calendar Event for Item
Using the selected task or project, the iOS/iPadOS Share Sheet, Shortcuts, and Omni Automation, a new event is created in the Calendar app containing the parameters and data from the selected OmniFocus item.
Video 1: New Event for Item |
Using the selected task or project, the iOS/iPadOS Share Sheet, Shortcuts, and Omni Automation, a new event is created in the Calendar app containing the parameters and data from the selected OmniFocus item. |
|
1 Share Sheet • The shortcut is set to be displayed in the Share Sheet.
2 Input Filter • The input is set to return only the URLs passed as input to the Share Sheet. In OmniFocus, the resulting URLs are either calendar links, TaskPaper representations, or a unique ID link to the selected item.
3 “Omni Automation Script” action • Contains an Omni Automation script that iterates the passed-in URLs to isolate the one containing the unique identifier for the selected item, and then uses that primaryKey value to retrieve other property values that are added to a JaaScript object (dictionary) and returned as a string to the next action. (see script below).
4 “Get Dictionary” action • Converts the passed-in JSON element into a dictionary whose elements are accessible to the other actions.
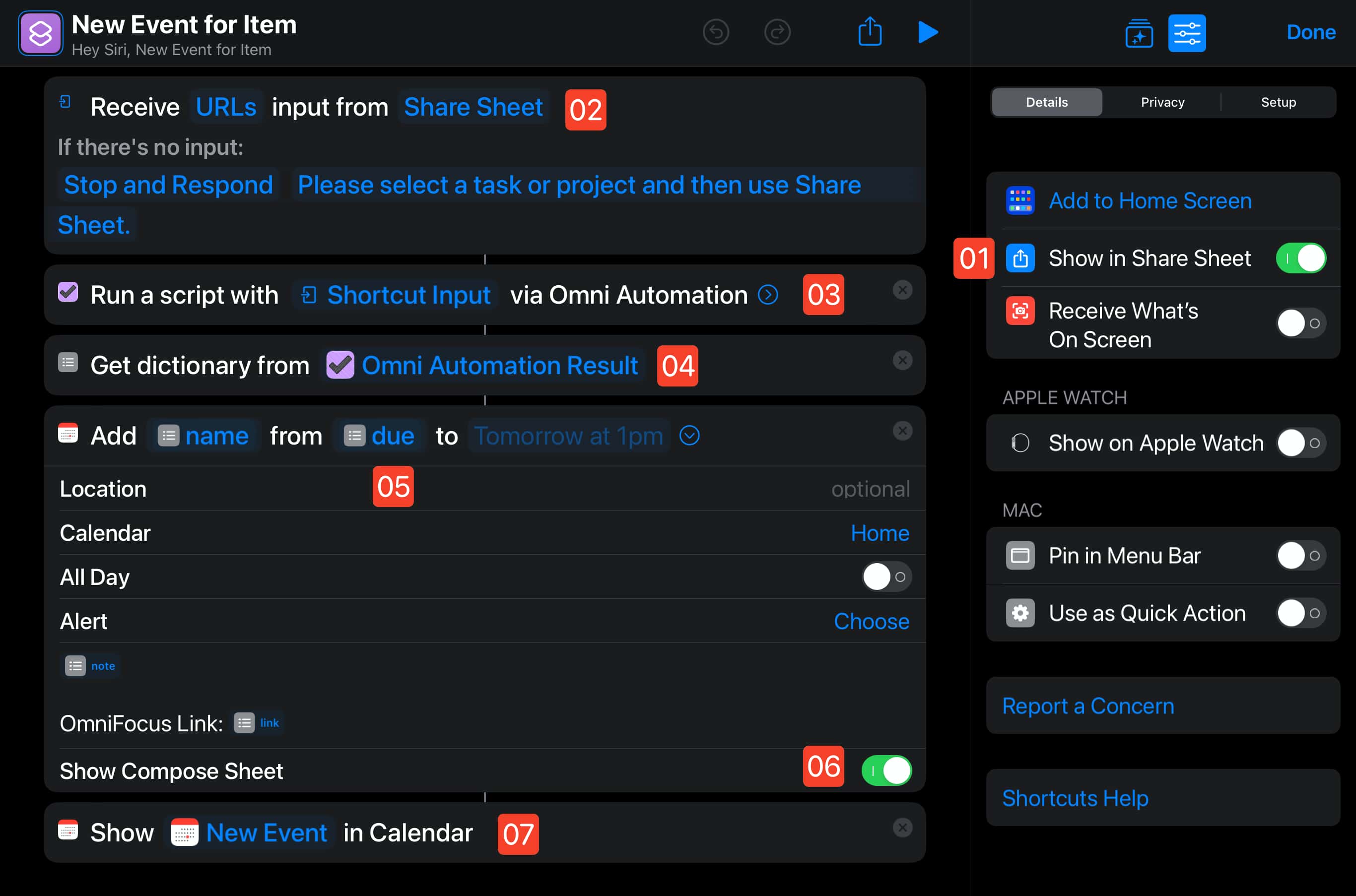
5 “Add Event” action • The dictionary elements (keys/values) are used with this action to create a new event in the Calendar app.
6 Event Compose Sheet • Displays the event options to the user to enable easy alterations and updates to the data used from the dictionary.
7 Show Event • The created event is displayed in the Calendar app. NOTE: a navigation link to the source OmniFocus element has been added to the note of the created event.
Omni Automation Script
This script serves two purposes:
- Filter the passed-in URLs to derive the unique identifier for the selected object, and create an object reference to the object. If the selected item is not a project or task (perhaps it’s a folder!), an error is displayed to the user and the workflow is stopped.
- Create and pass a JavaScript dictionary of relevant properties and values, and pass it as a string to the next action in the workflow.
Retrieve and Pass Properties of Selected Item
(async () => {
try {
// INPUT IS URL OR ARRAY OF URL
var passedData = argument.input
if (typeof passedData === "string"){passedData = [passedData]}
var url = ""
passedData.forEach(item => {
if (
item.startsWith('omnifocus:') &&
!item.includes('?') &&
!item.includes(',')
){url = item}
})
segments = url.split("/")
var id = segments[4]
var type = segments[3]
if(type === "task"){
// OBJECT REFERENCE TO ITEM
var item = Task.byIdentifier(id)
var type = "task"
if(!item){
var item = Project.byIdentifier(id)
var type = "project"
}
// IF A DICTIONARY IS TO BE PASSED
itemProperties = new Object()
itemProperties["primaryKey"] = id
itemProperties["type"] = type
itemProperties["link"] = "omnifocus:///task/" + id
itemProperties["name"] = item.name
itemProperties["note"] = item.note || ""
itemProperties["due"] = item.dueDate
var duration = item.estimatedMinutes
if(duration){
itemProperties["duration"] = duration
} else {
itemProperties["duration"] = 0
}
// PASS ITEM DICTIONARY
return JSON.stringify(itemProperties)
} else {
throw {
name: "Selection Issue",
message: "Please select a single task or project."
}
}
}
catch(err){
if(!err.message.includes("cancelled")){
await new Alert(err.name, err.message).show()
}
throw `${err.name}\n${err.message}`
}
})();