Timer
Timers enable your automation scripts to perform tasks over a period of time, or after a specified delay. You create a Timer object using one of these functions:
once(intervalSeconds: Number, actionFunction: Function) → (Timer) Makes a new Timer that will fire once, after the specified interval (in seconds from the current time). When the Timer fires, the passed-in function is called, passing the Timer instance as its argument.
repeating(intervalSeconds: Number, actionFunction: Function) → (Timer) Makes a new Timer that will fire repeatedly after the specified interval (in seconds, with the first invocation happening that interval after the current time). When the Timer fires, the passed-in function is called, passing the created Timer object as its argument.
Instance Functions
The only instance method available for use with a Timer object is a method for stopping a repeating timer:
cancel() → (Timer) Stop the timer.
The cancel() method is called within the repeating timer’s function, after a specified condition is met.
Instance Properties
The single property of a Timer object is its assigned time value:
interval (r/o) (Number) The number of seconds the timer is set to wait.
Let’s examine the two types of Timer objects, delayed and repeating.
Delayed Timer
A delayed Timer is useful for situations where you want a script to execute after a specified time period. To create a delayed timer, call the once handler on the Timer class and provide two items: 1) the number of seconds the timer should wait before executing 2) a function containing a passed referenced to the created timer object, and the function code to execute.
In the following example, the script will log the start time (in milliseconds) and the time when the passed function is executed:
Delay Timer
// delay a specified amount
start = new Date().getTime()
console.log(start)
Timer.once(5, function(timer){
now = new Date().getTime()
console.log(now)
})
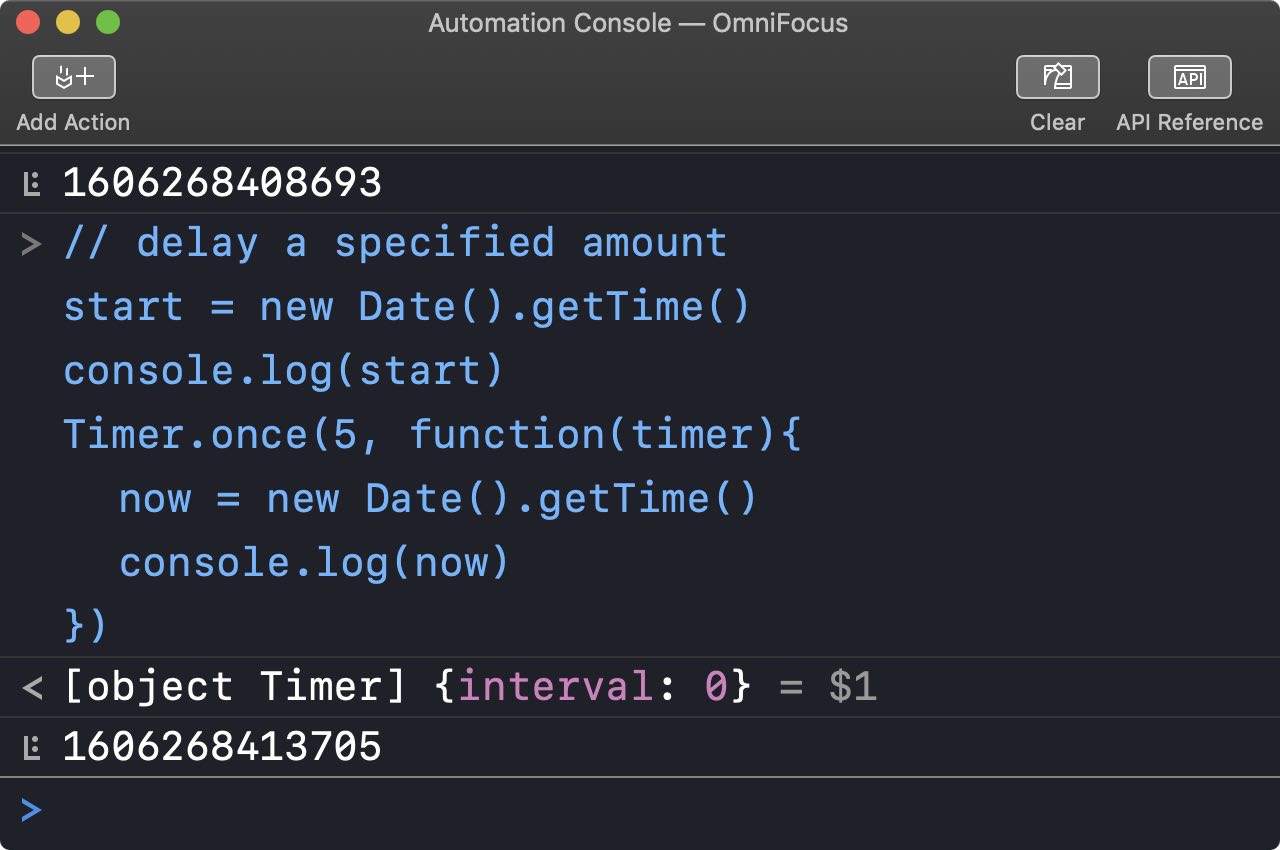
Repeating Timer: Stop after Duration
Repeating timers are designed to stop after a condition is met. In this first example of a repeating timer, the condition is that a specified amount of time has passed.
This example uses the standard JavaScript getTime() method on the Date class to get the current time (in milliseconds) to determine if a specified number of seconds (in this case 20000 milliseconds or 20 seconds) has passed. If it has, then the function is halted by calling the cancel() method on the passed timer object. Note that a time value of one-second (line 4) is passed to the function as the interval between function repetitions.
Repeating Timer: Stop after Duration
// repeat function after interval, stopping after duration
start = new Date().getTime()
console.log(start)
Timer.repeating(1, function(timer){
now = new Date().getTime()
console.log(now)
console.log(now - start)
if (now - start > 20000){
console.log('done')
timer.cancel()
}
})
Repeating Timer: Stop after Specified Repetition
The condition for stopping the following example of a repeating timer is that a specified number of repetitions have occurred. Note that the time value for the delay between repetitions is five seconds (line 6).
Timer: Stop After Repetition
// repeat function after interval x times
start = new Date().getTime()
console.log(start)
counter = 0
repeats = 5
Timer.repeating(5, function(timer){
now = new Date().getTime()
console.log(now)
console.log(now - start)
if (counter === repeats){
console.log('done')
timer.cancel()
} else {
counter = counter + 1
}
})
Repeating Timer: Stop on Condition
The following example of a repeating timer is an automated race between shapes in an OmniGraffle document. And the condition for stopping the repeating timer is that one of the shapes will reach the finish line on the right side of the document. The timer function is set to execute every one-half second (0.5 line 51) and the function relies upon the standard JavaScript methods of floor() and random() of the Math class to determine how many pixels each graphic moves to the right. Fun stuff!
Object Race
alert = new Alert("Object Race", "This example script will delete all graphics on the current canvas and resize it.\n\nShould the script continue?")
alert.addOption("Continue")
alert.addOption("Stop")
alert.show(function(result){
if (result != 0){
throw new Error('script cancelled')
} else {
var cnvs = document.windows[0].selection.canvas
// delete existing graphics
g = cnvs.graphics
g = g.reverse()
for(i = 0; i < g.length; i++){
g[i].remove()
}
// resize and center the canvas
cnvs.size = new Size(1024, 768)
cnvs.canvasSizingMode = CanvasSizingMode.Fixed
document.windows[0].centerVisiblePoint = cnvs.background.geometry.center
// add shapes
var diamond = cnvs.newShape()
var circle = cnvs.newShape()
var square = cnvs.newShape()
var finishLine = cnvs.newLine()
var startingLine = cnvs.newLine()
diamond.textUnitRect = new Rect(0.14, 0.12, 0.75, 0.75)
diamond.shape = "Diamond"
diamond.geometry = new Rect(32.00, 334.00, 100.00, 100.00)
diamond.shadowColor = null
diamond.fillColor = Color.RGB(1.0, 0.75, 0.75)
circle.textUnitRect = new Rect(0.10, 0.15, 0.80, 0.70)
circle.shape = "Circle"
circle.geometry = new Rect(32.00, 483.00, 100.00, 100.00)
circle.shadowColor = null
circle.fillColor = Color.RGB(0.75, 1.0, 0.75)
square.shape = "Rectangle"
square.geometry = new Rect(32.00, 185.00, 100.00, 100.00)
square.shadowColor = null
square.fillColor = Color.RGB(0.75, 0.75, 1.0)
finishLine.strokeThickness = 8
finishLine.lineType = LineType.Curved
finishLine.shadowColor = null
finishLine.points = [new Point(886.00, 83.59), new Point(886.00, 684.41)]
finishLineHorizontal = 886.00
startingLine.strokeThickness = 8
startingLine.lineType = LineType.Curved
startingLine.shadowColor = null
startingLine.points = [new Point(168.00, 83.59), new Point(168.00, 684.41)]
var factor = 50
Timer.repeating(0.5,function(timer){
//square
squareGeometry = square.geometry
squareWidth = squareGeometry.width
squareOrigin = squareGeometry.origin
squareMovement = Math.floor(Math.random() * factor)
newSquareHOffset = squareOrigin.x + squareMovement
squareOrigin = new Point(newSquareHOffset,squareOrigin.y)
squareGeometry.origin = squareOrigin
square.geometry = squareGeometry
if (newSquareHOffset + squareWidth >= finishLineHorizontal){
finishLine.strokeColor = Color.blue
square.fillColor = Color.blue
timer.cancel()
}
// diamond
diamondGeometry = diamond.geometry
diamondWidth = diamondGeometry.width
diamondOrigin = diamondGeometry.origin
diamondMovement = Math.floor(Math.random() * factor)
newDiamondHOffset = diamondOrigin.x + diamondMovement
diamondOrigin = new Point(newDiamondHOffset,diamondOrigin.y)
diamondGeometry.origin = diamondOrigin
diamond.geometry = diamondGeometry
if (newDiamondHOffset + diamondWidth >= finishLineHorizontal){
finishLine.strokeColor = Color.red
diamond.fillColor = Color.red
timer.cancel()
}
// circle
circleGeometry = circle.geometry
circleWidth = circleGeometry.width
circleOrigin = circleGeometry.origin
circleMovement = Math.floor(Math.random() * factor)
newCircleHOffset = circleOrigin.x + circleMovement
circleOrigin = new Point(newCircleHOffset,circleOrigin.y)
circleGeometry.origin = circleOrigin
circle.geometry = circleGeometry
if (newCircleHOffset + circleWidth >= finishLineHorizontal){
finishLine.strokeColor = Color.green
circle.fillColor = Color.green
timer.cancel()
}
})
}
})