SharePanel Class
An interface that can display the system share interaction for the given items.
new SharePanel(items: Array of URL, String, Image, or FileWrapper) → (SharePanel) • Create a new share panel with the given items.
Instance properties:
items (Array of URL, String, Image, or FileWrapper) • The items that will be supplied to the system share interaction upon calling show.
Instance functions:
addItem(shareItem: URL, String, Image, or FileWrapper) → ( ) • Appends the item to the end of items.
addItems(shareItems: Array of URL, String, Image, or FileWrapper) → ( ) • Appends the contents of the given array to the end of items.
removeItem(shareItem: URL, String, Image, or FileWrapper) → ( ) • Removes the first occurrence of the item from items if it is present in items.
removeItems(shareItems: Array of URL, String, Image, or FileWrapper) → ( ) • Removes the first occurrence of each member of the given array from items if that member is present in items.
clearItems() → ( ) • Sets items to an empty array. Note: Calling show when items is empty results in an error, so be sure to add new items after calling this and before calling show.
show() → (Promise) • Presents the share panel for its items. NOTE: No specific share method will be selected when the panel is displayed. The desired sharing method must be chosen by the user.
Example: AirDrop OmniFocus Database Backup
Here is an example script that use the SharePanel class to AirDrop a backup copy of the OmniFocus database:
Share Database Backup
fileTypeID = 'com.omnigroup.omnifocus.filetype.ofocus-backup'
defaultName = "OmniFocus DB Backup"
wrapperPromise = document.makeFileWrapper(defaultName, fileTypeID)
wrapperPromise.then(function(wrapper){
new SharePanel([wrapper]).show()
})
Share Database Backup (Asynchronous)
(async () => {
try {
fileTypeID = 'com.omnigroup.omnifocus.filetype.ofocus-backup'
defaultName = "OmniFocus DB Backup"
wrapper = await document.makeFileWrapper(defaultName, fileTypeID)
new SharePanel([wrapper]).show()
}
catch(err){
new Alert(err.name, err.message).show()
}
})();
When the script is run, the share sheet appears from which you can select the option to AirDrop the exported file:
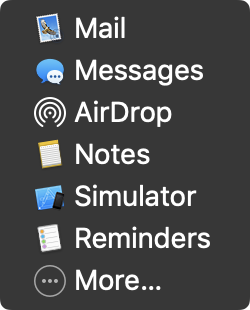
Once you have chosen the sharing method from the Share Sheet, the corresponding share dialog appears:
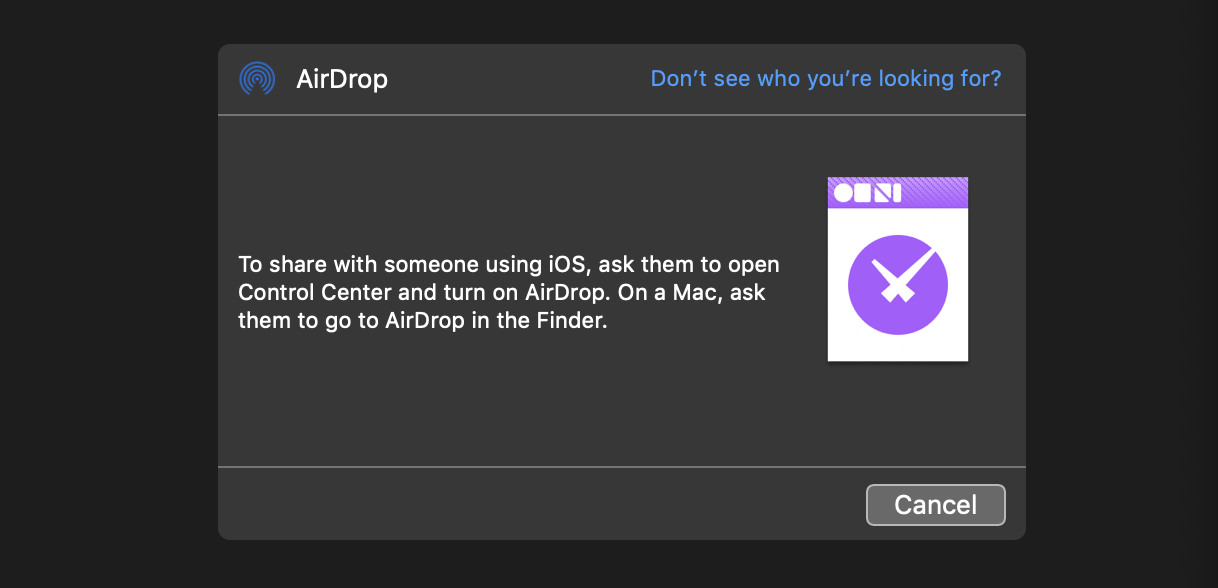
Plug-In: Share Clipboard Text
Here’s a useful plug-in that summons the system Share Panel to share the current text on the clipboard. Great for creating a new Apple note, or Drafts document!
Share Clipboard Text
/*{
"type": "action",
"targets": ["omnifocus", "omniplan", "omnioutliner", "omnigraffle"],
"author": "Otto Automator",
"identifier": "com.omni-automation.all.share-clipboard-text",
"version": "1.1",
"description": "This plug-in will display the system Share Panel for sharing the current text contents of the pasteboard.",
"label": "Share Clipboard Text",
"shortLabel": "Share Clipboard Text",
"paletteLabel": "Share Clipboard Text",
"image": "square.and.arrow.up.circle.fill"
}*/
(() => {
const action = new PlugIn.Action(function(selection, sender){
clipboardText = Pasteboard.general.string
new SharePanel([clipboardText]).show()
});
action.validate = function(selection, sender){
return (Pasteboard.general.string.length > 0)
};
return action;
})();
Share OmniFocus Attachments
Here’s a script that will share the attachments of the selected task or project:
Share Attachments
try {
sel = document.windows[0].selection
selCount = sel.tasks.length + sel.projects.length
if(selCount === 1){
if (sel.tasks.length === 1){
if (sel.tasks[0].attachments.length === 0){
throw new Error("The selected task has no attachments to share.")
}
new SharePanel(sel.tasks[0].attachments).show()
} else {
if (sel.projects[0].attachments.length === 0){
throw new Error("The selected project has no attachments to share.")
}
new SharePanel(sel.projects[0].attachments).show()
}
} else {
throw new Error("Please select a single project or task with attachments.")
}
}
catch(err){
new Alert("SELECTION ISSUE", err.message).show()
}