Pasteboard
A pasteboard (also referred to as “the clipboard”) temporarily holds representations of items of different types for transfer between different applications or different locations in the application.
Class Properties
general (Pasteboard r/o) • The Pasteboard used for user-initiated copy/paste support.
Class Functions
makeUnique() → (Pasteboard) • Creates a new unique pasteboard.
Instance Properties
URL (URL or null) • Gets or sets the pasteboard content as a single URL.
URLs (Array of URL or null) • Gets or sets the pasteboard content as a list of URLs.
color (Color or null) • Gets or sets the pasteboard content as a single color.
colors (Array of Color or null) • Gets or sets the pasteboard content as a list of colors.
hasColors (boolean r/o) • Returns true if the pasteboard contains one or more colors.
hasImages (boolean r/o) • Returns true if the pasteboard contains one or more images.
hasStrings (boolean r/o) • Returns true if the pasteboard contains one or more strings.
hasURLs (boolean r/o) • Returns true if the pasteboard contains one or more URLs.
image (Image or null) • Gets or sets the pasteboard content as a single image.
images (Array of Image or null) • Gets or sets the pasteboard content as a list of images.
items (Array of Pasteboard.Item) • The array of individual items on the pasteboard, each potentially with their own set of types.
string (String or null) • Gets or sets the pasteboard content as a single plain-text string.
strings (String or null) • Gets or sets the pasteboard content as a list of plain-text strings.
types (Array of TypeIdentifier r/o) • The list of pasteboard types currently available on the pasteboard.
Log User Clipboard Text
if (Pasteboard.general.hasStrings){
console.log(Pasteboard.general.string)
} else {console.log('No Clipboard Text')}
Set User Pasteboard String
Pasteboard.general.string = "How Now Brown Cow"
console.log(Pasteboard.general.string)
Set/Get Temporary Pasteboard Text
tempClip = Pasteboard.makeUnique()
tempClip.string = "The rain in Spain falls mainly on the plain."
console.log(tempClip.string)
NOTE: altering the content of a unique pasteboard does not effect the contents of the user pasteboard (main clipboard).
Save Plain-Text from Pasteboard to File
(async () => {
try {
if(Pasteboard.general.hasStrings){
data = Data.fromString(Pasteboard.general.string)
wrapper = FileWrapper.withContents('Pasteboard.txt', data)
filesaver = new FileSaver()
urlObj = await filesaver.show(wrapper)
console.log(urlObj.string)
urlObj.open()
}
}
catch(err){
if(!err.causedByUserCancelling){
new Alert(err.name, err.message).show()
}
}
})();
Instance Functions
availableType(types: Array of TypeIdentifier) → (TypeIdentifier or null) • The first type from the provided list which is available on the pasteboard, or null if none are available.
addItems(items: Array of Pasteboard.Item) → ( ) • Appends the new items to the pasteboard.
clear() → ( ) • Remove all items from the pasteboard.
dataForType(type: TypeIdentifier) → (Data or null) • The Data representation for the given type in this pasteboard, or null if none is available.
setDataForType(data: Data, type: TypeIdentifier) → ( ) • Set the Data representation for the given type in this pasteboard, replacing any previously set data.
stringForType(type: TypeIdentifier) → (String or null) • The String representation for the given type in this pasteboard, or null if none is available.
setStringForType(string: String, type: TypeIdentifier) → ( ) • Set the String representation for the given type in this pasteboard, replacing any previously set data.
Save Pasteboard Image to File
(async () => {
try {
if(Pasteboard.general.hasImages){
data = Pasteboard.general.dataForType(TypeIdentifier.png)
wrapper = FileWrapper.withContents('IMG-CLIP.png', data)
filesaver = new FileSaver()
urlObj = await filesaver.show(wrapper)
console.log(urlObj.string)
urlObj.open()
}
}
catch(err){
if(!err.causedByUserCancelling){
new Alert(err.name, err.message).show()
}
}
})();
Get Specific Type from Pasteboard
(async () => {
try {
targetType = new TypeIdentifier("public.utf8-plain-text")
if(Pasteboard.general.availableType([targetType])){
items = Pasteboard.general.items
var i
for (i = 0; i < items.length; i++) {
var data = items[i].dataForType(targetType)
if(data){
console.log(data.toString())
break
}
}
}
wrapper = FileWrapper.withContents('Pasteboard.txt', data)
filesaver = new FileSaver()
urlObj = await filesaver.show(wrapper)
console.log(urlObj.string)
urlObj.open()
}
catch(err){
if(!err.causedByUserCancelling){
new Alert(err.name, err.message).show()
}
}
})();
Save Clipboard RTF to Disk
(async () => {
try {
targetType = TypeIdentifier.rtf
if(Pasteboard.general.availableType([targetType])){
data = Pasteboard.general.dataForType(TypeIdentifier.rtf)
wrapper = FileWrapper.withContents('TEST-RTF-EXPORT.rtf', data)
filesaver = new FileSaver()
urlObj = await filesaver.show(wrapper)
console.log(urlObj.string)
urlObj.open()
}
}
catch(err){
if(!err.causedByUserCancelling){
new Alert(err.name, err.message).show()
}
}
})();
If there’s a map link on the clipboard, open it:
Open Copied Map URL
if(Pasteboard.general.hasURLs){
urlStr = Pasteboard.general.stringForType(TypeIdentifier.URL)
if(urlStr.startsWith("https://maps.")){
URL.fromString(urlStr).open()
}
}
Pasteboard.Item Class
A pasteboard item is the generic instance of the Pasteboard.Item class, a single instance of one of the data types supported by the Pasteboard class.
new Pasteboard.Item() → (Pasteboard.Item) • Make a new empty pasteboard item with no contents.
Instance Properties
The properties of an instance of the Pasteboard.Item class:
types (Array of TypeIdentifier r/o) • The list of types available for this pasteboard item.
Instance Functions
The functions used to manipulate an instance of the Pasteboard.Item class:
dataForType(type:TypeIdentifier) → (Data or null) • The Data representation for the given type in this pasteboard item, or null if none is available.
setDataForType(data:Data, type:TypeIdentifier) → ( ) • Set the Data representation for the given type in this pasteboard item, replacing any previously set data.
stringForType(type:TypeIdentifier) → (String or null) • The String representation for the given type in this pasteboard item, or null if none is available.
setStringForType(string:String, type:TypeIdentifier) → ( ) • Set the String representation for the given type in this pasteboard item, replacing any previously set data.
Place Image from Data on Pasteboard
img64 = "iVBORw0KGgoAAAANSUhEUgAAABMAAAASCAYAAAC5DOVpAAAAAXNSR0IArs4c6QAAAAlwSFlzAAALEwAACxMBAJqcGAAAAgtpVFh0WE1MOmNvbS5hZG9iZS54bXAAAAAAADx4OnhtcG1ldGEgeG1sbnM6eD0iYWRvYmU6bnM6bWV0YS8iIHg6eG1wdGs9IlhNUCBDb3JlIDUuNC4wIj4KICAgPHJkZjpSREYgeG1sbnM6cmRmPSJodHRwOi8vd3d3LnczLm9yZy8xOTk5LzAyLzIyLXJkZi1zeW50YXgtbnMjIj4KICAgICAgPHJkZjpEZXNjcmlwdGlvbiByZGY6YWJvdXQ9IiIKICAgICAgICAgICAgeG1sbnM6dGlmZj0iaHR0cDovL25zLmFkb2JlLmNvbS90aWZmLzEuMC8iPgogICAgICAgICA8dGlmZjpSZXNvbHV0aW9uVW5pdD4yPC90aWZmOlJlc29sdXRpb25Vbml0PgogICAgICAgICA8dGlmZjpDb21wcmVzc2lvbj41PC90aWZmOkNvbXByZXNzaW9uPgogICAgICAgICA8dGlmZjpPcmllbnRhdGlvbj4xPC90aWZmOk9yaWVudGF0aW9uPgogICAgICAgICA8dGlmZjpQaG90b21ldHJpY0ludGVycHJldGF0aW9uPjI8L3RpZmY6UGhvdG9tZXRyaWNJbnRlcnByZXRhdGlvbj4KICAgICAgPC9yZGY6RGVzY3JpcHRpb24+CiAgIDwvcmRmOlJERj4KPC94OnhtcG1ldGE+CobSriQAAAGeSURBVDgRjZS/K4VhFMfv9TOykM0iPzJgkDIYDCyUxSIpi0kZDAaDSUb8CQYmKWGQRXdQykgMSukOlIVIym8+3+s5b8frva5Tn3vOc873fd7ned7z3HTqb2uh3ABlcAVH8Ab/tmKUE3AOn/AKZyG+wS9BNRS0WhQHoEmMDPGsGyt/DV2Q16qonIJNYn6KXGtC/pFcByTaClmbwPv6oLZt+9oFtYpQj1w70Qd4oeLjSJFKLSTUpZk2TUkIxvGH8GSF4NfdeI24040tHCXQR4lMZ6XP3hRlCgeVSFZBq6vz8oeQvMMP+UKeWP13AnYs3V6nXrKC/CLYEXid4mGwl9szfV6k7raC+V4vCLFuglrCNObbvHYrJtB2S73AxZsx7b1pi4JIAm+7DLR12QDMQFoDbPvbRb87RKbNJbUKNaAte4RYL5oH6z89VAO6crrs0r7Djy0yzlkPv3rDCzTDHtjk5rPkdCf3Q20On9fGqGThEmyCuH+mpp5cBjsmwmTTCpMuvE16S30y6VE71HhN/2n9MAiNUA5abQY2QF/7l30BWpSQgTjp6dQAAAAASUVORK5CYII="
imgData = Data.fromBase64(img64)
item = new Pasteboard.Item()
item.setDataForType(imgData,TypeIdentifier.jpeg)
Pasteboard.general.clear()
Pasteboard.general.addItems([item])
Replace String on Pastebord
Here's an Omni Automation plug-in that replaces the designated string in the text currently on the global Pasteboard:
Replace String on Pasteboard
/*{
"type": "action",
"targets": ["omnifocus","omnigraffle","omnioutliner","omniplan"],
"author": "Otto Automator",
"identifier": "com.omni-automation.all.replace-string-on-pasteboard",
"version": "1.1",
"description": "This action will replace the provided string with the provided replacement string in the text currently on the user pasteboard. NOTE: the resulting text will be in plain-text format.",
"label": "Replace String on Pasteboard",
"shortLabel": "Find-Change Clipboard",
"paletteLabel": "Find-Change Clipboard",
"image": "text.viewfinder"
}*/
(() => {
const action = new PlugIn.Action(async function(selection, sender){
textInputField01 = new Form.Field.String(
"textInput01",
"Search for",
null
)
textInputField02 = new Form.Field.String(
"textInput02",
"Replace with",
null
)
checkSwitchField = new Form.Field.Checkbox(
"checkboxSwitch",
"Case-insensitive and word-only",
true
)
inputForm = new Form()
inputForm.addField(textInputField01)
inputForm.addField(textInputField02)
inputForm.addField(checkSwitchField)
inputForm.validate = function(formObject){
inputText01 = formObject.values['textInput01']
inputText01Status = (!inputText01)?false:true
inputText02Status = true
validation = (inputText01Status && inputText02Status) ? true:false
return validation
}
formPrompt = "Search and replace text on the clipboard:"
buttonTitle = "Continue"
formObject = await inputForm.show(formPrompt,buttonTitle)
searchString = formObject.values['textInput01']
replacementString = formObject.values['textInput02']
if (!replacementString){replacementString = ""}
checkboxStatus = formObject.values['checkboxSwitch']
contentString = Pasteboard.general.string
if (checkboxStatus){
re = new RegExp(`\\b${searchString}\\b`, 'gi')
newString = contentString.replace(re,replacementString)
} else {
re = new RegExp(`${searchString}`, 'g')
newString = contentString.replace(re,replacementString)
}
Pasteboard.general.string = newString
});
action.validate = function(selection, sender){
return (Pasteboard.general.hasStrings)
};
return action;
})();
Pasteboard Image as Attachment
The following script will add the current pasteboard image to the selected OmniFocus project or task as an attachment:
Clipboard Image as Attachment
try {
sel = document.windows[0].selection
selCount = sel.tasks.length + sel.projects.length
if(selCount === 1){
if (sel.tasks.length === 1){
var selectedItem = sel.tasks[0]
} else {
var selectedItem = sel.projects[0]
}
} else {
throw {
name: "Selection Issue",
message: "Please select a single project or task."
}
}
if(Pasteboard.general.hasImages){
imageType = new TypeIdentifier("public.png")
ext = ".png"
data = Pasteboard.general.dataForType(imageType)
if(!data){
imageType = new TypeIdentifier("public.tiff")
ext = ".tif"
data = Pasteboard.general.dataForType(imageType)
}
if(!data){
imageType = new TypeIdentifier("public.jpeg")
ext = ".jpg"
data = Pasteboard.general.dataForType(imageType)
}
if(!data){
imageType = new TypeIdentifier("public.image")
ext = ""
data = Pasteboard.general.dataForType(imageType)
}
fmtr = Formatter.Date.withFormat('eee-MMM-dd-yyyy-h-mm-ss-a')
imageName = "Clip-Image_" + fmtr.stringFromDate(new Date()) + ext
wrapper = FileWrapper.withContents(imageName, data)
selectedItem.addAttachment(wrapper)
} else {
throw {
name: "Missing Resource",
message: "There is no image on the clipboard."
}
}
}
catch(err){
new Alert(err.name, err.message).show()
}
Fetch Chart to Pasteboard
The following plug-in demonstrates how charts can be generated using an online service, and the resulting chart images be automatically placed on the Omni application’s pasteboard (clipboard) ready to be pasted.
The URL APIs from the QuickChart website (quickchart.io) are used to generate the chart images.
You can edit this plug-in to use data from the current Omni document as the data sets for the charts, instead of the provided example data sets.
Fetch Chart Image
/*{
"type": "action",
"targets": ["omnifocus","omnigraffle","omnioutliner","omniplan"],
"author": "Otto Automator",
"identifier": "com.omni-automation.all.fetch-chart",
"version": "1.1",
"description": "This action generates a chart using the QuickChart (quickchart.io) APIs.",
"label": "Fetch Chart Image",
"shortLabel": "Fetch Chart",
"paletteLabel": "Fetch Chart",
"image": "chart.bar.xaxis"
}*/
(() => {
const action = new PlugIn.Action(async function(selection, sender){
try {
menuItems = ["Doughnut","Bar","Pie","Radial Guage","Line Graph"]
menuIndexes = menuItems.map((item, index) => index)
menuElement = new Form.Field.Option(
"menuElement",
"Chart Type",
menuIndexes,
menuItems,
0
)
inputForm = new Form()
inputForm.addField(menuElement)
inputForm.validate = function(formObject){
return true
}
formPrompt = "Fetch an image from QuickChart (quickchart.io):"
buttonTitle = "Continue"
formObject = await inputForm.show(formPrompt,buttonTitle)
menuIndex = formObject.values['menuElement']
chosenChartType = menuItems[menuIndex]
console.log('Chosen chart: ',chosenChartType)
// QuickChart https://quickchart.io/
url = "https://quickchart.io/chart?c="
chartDataSets = [
"{type:'doughnut',data:{labels:['January','February','March','April','May'],datasets:[{data:[50,60,70,180,190]}]},options:{plugins:{doughnutlabel:{labels:[{text:'550',font:{size:20}},{text:'total'}]}}}}",
"{type:'bar',data:{labels:['Q1','Q2','Q3','Q4'], datasets:[{label:'Users',data:[50,60,70,180]},{label:'Revenue',data:[100,200,300,400]}]}}",
"{type:'pie',data:{labels:['January','February', 'March','April', 'May'], datasets:[{data:[50,60,70,180,190]}]}}",
"{type:'radialGauge',data:{datasets:[{data:[70],backgroundColor:'green'}]}}",
"{type:'line',data:{labels:['January','February', 'March','April', 'May'], datasets:[{label:'Dogs', data: [50,60,70,180,190], fill:false,borderColor:'blue'},{label:'Cats', data:[100,200,300,400,500], fill:false,borderColor:'green'}]}}"
]
chartDataSet = chartDataSets[menuIndex]
url = url + encodeURIComponent(chartDataSet)
URL.fromString(url).fetch(data => {
item = new Pasteboard.Item()
item.setDataForType(data,TypeIdentifier.png)
Pasteboard.general.items = [item]
alert = new Alert(chosenChartType, "Chart on pasteboard.")
alert.addOption("Done")
alert.addOption("Website")
alert.show(function(result){
if (result == 0){
console.log("Button 1")
} else {
var url = "https://quickchart.io"
URL.fromString(url).open()
}
})
})
}
catch(err){
if(!err.causedByUserCancelling){
new Alert(err.name, err.message).show()
}
}
});
action.validate = function(selection, sender){
return true
};
return action;
})();
Doughnut:
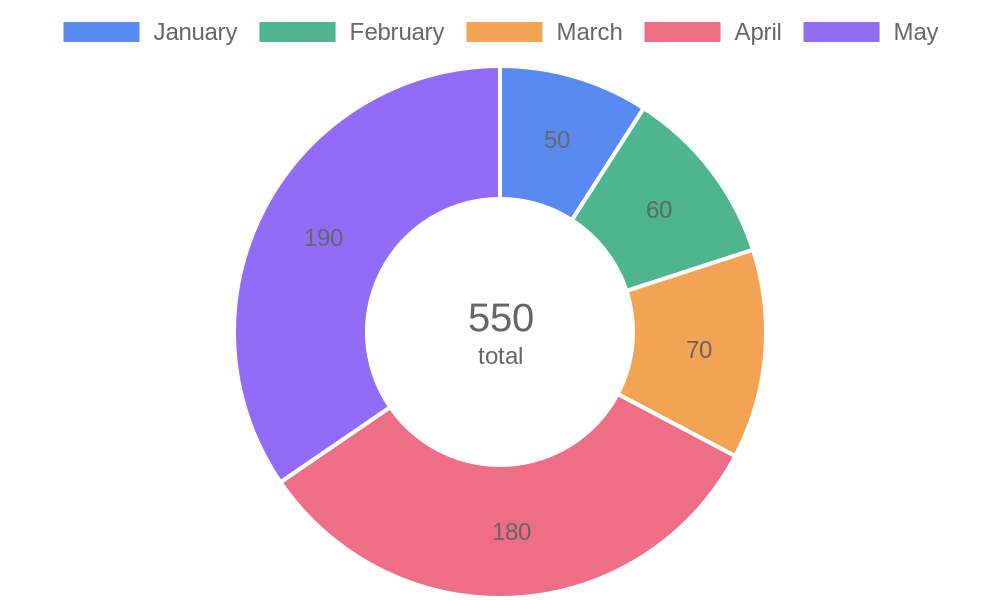
Line:
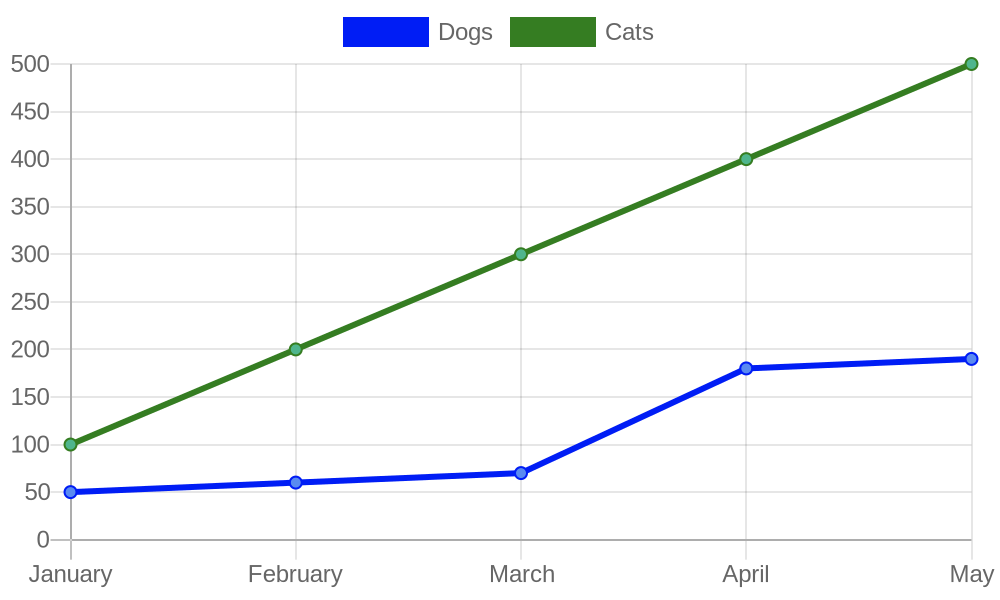
Bar:
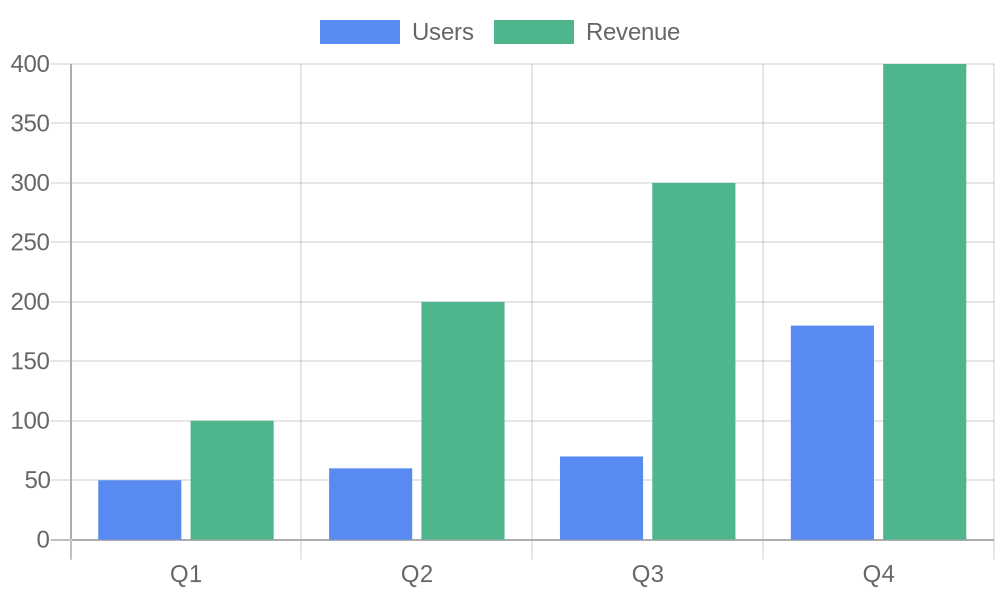
Pie:
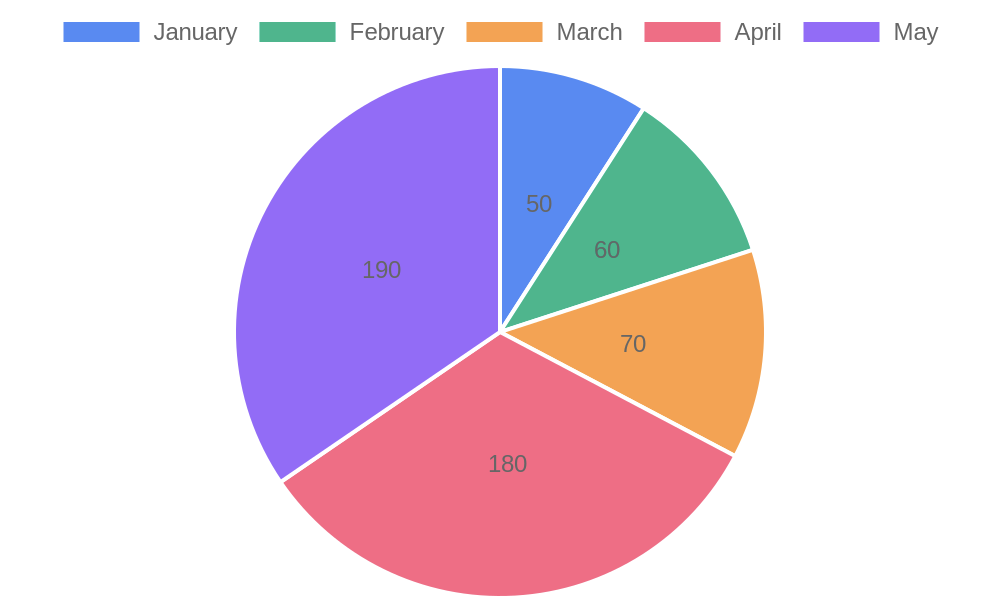
Radial Guage:
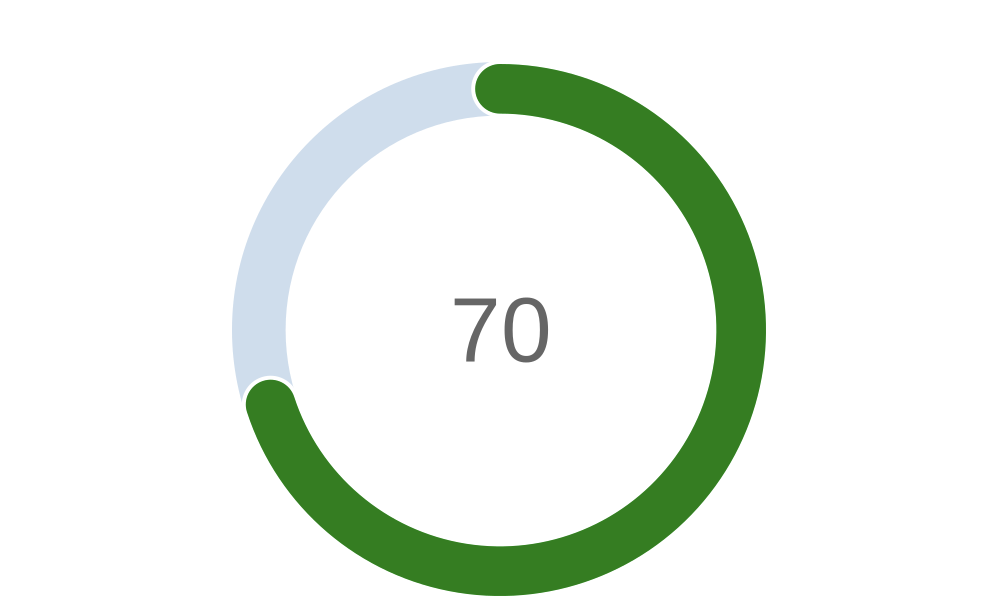