Plug-In: Table Cloner Plug-In
Here’s an Omni Automation plug-in for OmniGraffle that creates a table cloned from the selected solid.
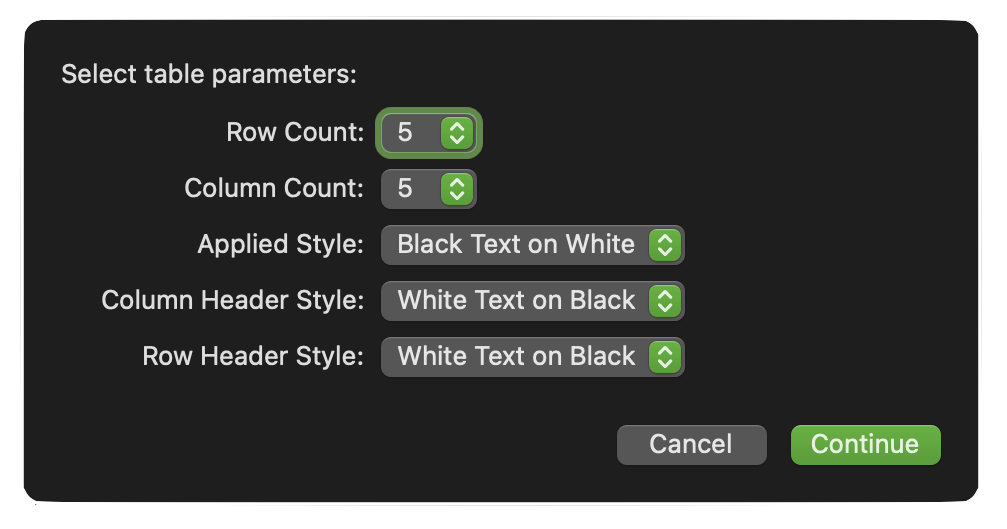
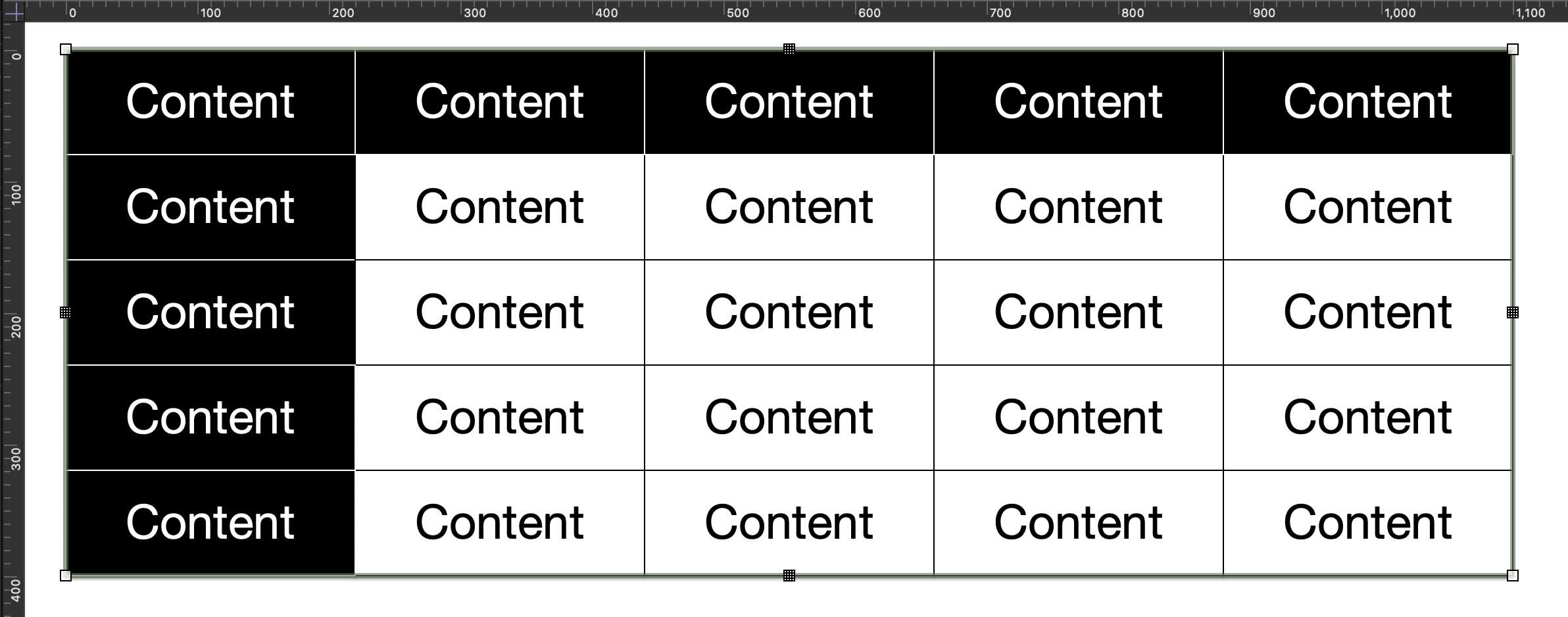
Return to: OmniGraffle Plug-In Collection
Table Cloner Plug-In
/*{
"type": "action",
"targets": ["omnigraffle"],
"author": "Otto Automator",
"identifier": "com.omni-automation.og.table-cloner",
"version": "1.0",
"description": "This plug-in creates a table of cells cloned from the selected solid.",
"label": "Create Cloned Table",
"shortLabel": "Create Cloned Table",
"paletteLabel": "Create Cloned Table",
"image": "tablecells"
}*/
(() => {
const action = new PlugIn.Action(async function(selection, sender){
try {
shape = selection.solids[0]
countLabels = ["2","3","4","5","6","7","8",
"9", "10", "11", "12", "15", "20"] countOptions = [2,3,4,5,6,7,8,9,10,11,12,15,20]
rowCountMenu = new Form.Field.Option(
"rowCount",
"Row Count",
countOptions,
countLabels,
5
)
columnCountMenu = new Form.Field.Option(
"columnCount",
"Column Count",
countOptions,
countLabels,
5
)
styleLabels = ["None", "Black Text on White",
"White Text on Black"] styleOptions = styleLabels.map((items, index) => index)
appliedTableStyleMenu = new Form.Field.Option(
"appliedTableStyle",
"Applied Table Style",
styleOptions,
styleLabels,
0
)
columnHeaderStyleMenu = new Form.Field.Option(
"columnHeaderStyle",
"Column Header Style",
styleOptions,
styleLabels,
0
)
rowHeaderStyleMenu = new Form.Field.Option(
"rowHeaderStyle",
"Row Header Style",
styleOptions,
styleLabels,
0
)
inputForm = new Form()
inputForm.addField(rowCountMenu)
inputForm.addField(columnCountMenu)
inputForm.addField(appliedTableStyleMenu)
inputForm.addField(columnHeaderStyleMenu)
inputForm.addField(rowHeaderStyleMenu)
formPrompt = "Select table parameters:"
buttonTitle = "Continue"
formObject = await inputForm.show(formPrompt, buttonTitle)
rowCount = formObject.values["rowCount"]
columnCount = formObject.values["columnCount"]
appliedTableStyle = formObject.values["appliedTableStyle"]
columnHeaderStyle = formObject.values["columnHeaderStyle"]
rowHeaderStyle = formObject.values["rowHeaderStyle"]
table = new Table(shape)
table.columns = columnCount
table.rows = rowCount
switch(appliedTableStyle){
case 1:
// Black Text on White
for (cell of table.graphics){
cell.fillColor = Color.white
cell.textColor = Color.black
cell.strokeColor = Color.black
}
break;
case 2:
// White Text on Black
for(cell of table.graphics){
cell.fillColor = Color.black
cell.textColor = Color.white
cell.strokeColor = Color.white
}
break;
default:
// None
}
switch(columnHeaderStyle){
case 1:
// Black Text on White
r = 0
for(c = 0; c < table.columns; c++){
cell = table.graphicAt(r,c)
cell.fillColor = Color.white
cell.textColor = Color.black
cell.strokeColor = Color.black
}
break;
case 2:
// White Text on Black
r = 0
for(c = 0; c < table.columns; c++){
cell = table.graphicAt(r,c)
cell.fillColor = Color.black
cell.textColor = Color.white
cell.strokeColor = Color.white
}
break;
default:
// None
}
switch(rowHeaderStyle){
case 1:
// Black Text on White
c = 0
for(r = 0; r < table.rows; r++){
cell = table.graphicAt(r,c)
cell.fillColor = Color.white
cell.textColor = Color.black
cell.strokeColor = Color.black
}
break;
case 2:
// White Text on Black
c = 0
for(r = 0; r < table.rows; r++){
cell = table.graphicAt(r,c)
cell.fillColor = Color.black
cell.textColor = Color.white
cell.strokeColor = Color.white
}
break;
default:
// None
}
selection.view.select([table], false)
}
catch(err){
if(!err.causedByUserCancelling){
new Alert(err.name, err.message).show()
}
}
});
action.validate = function(selection, sender){
return (selection.solids.length === 1)
};
return action;
})();