Plug-In: Overlay Image Notes
This plug-in will overlay the notes of a selected image, providing options for positioning and text color.
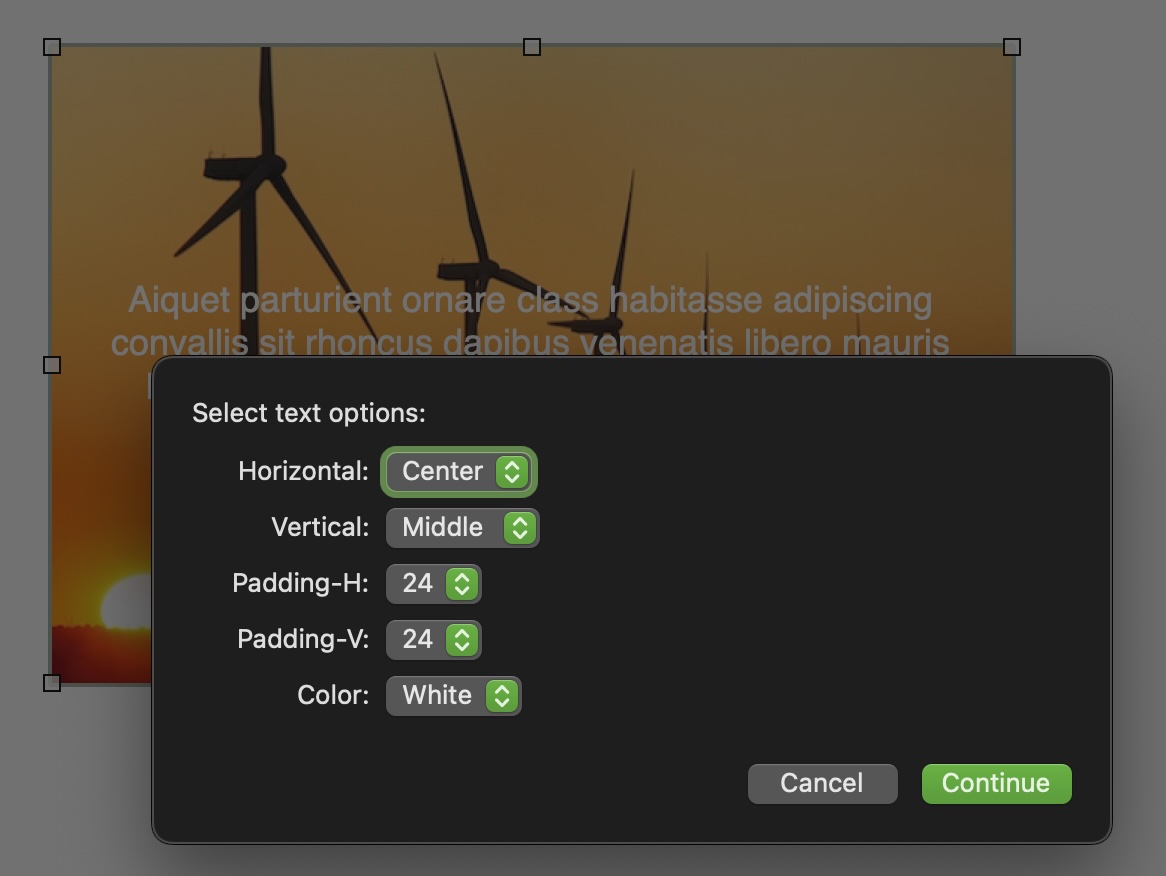
Ovelay Image Notes Plug-In
/*{
"type": "action",
"targets": ["omnigraffle"],
"author": "Otto Automator",
"identifier": "com.omni-automation.og.overlay-image-notes",
"version": "1.0",
"description": "WIll overlay the notes of the selected image and position the text according to selected options.",
"label": "Overlay Notes on Image",
"shortLabel": "Overlay Notes",
"paletteLabel": "Overlay Notes",
"image": "dock.arrow.down.rectangle"
}*/
(() => {
var preferences = new Preferences() // NO ID = PLUG-IN ID
const action = new PlugIn.Action(async function(selection, sender){
try {
defaultTextVerticalIndex = 0
defaultTextHorizontalIndex = 0
defaultTextColorIndex = 0
defaultTextVerticalPadding = 0
defaultTextHorizontalPadding = 0
textHorizontalIndex = preferences.readNumber("textHorizontalIndex")
if(!textHorizontalIndex){
textHorizontalIndex = defaultTextHorizontalIndex
}
textVerticalIndex = preferences.readNumber("textVerticalIndex")
if(!textVerticalIndex){
textVerticalIndex = defaultTextVerticalIndex
}
textColorIndex = preferences.readNumber("textColorIndex")
if(!textColorIndex){
textColorIndex = defaultTextColorIndex
}
textHorizontalPadding = preferences.readNumber("textHorizontalPadding")
if(!textHorizontalPadding){
textHorizontalPadding = defaultTextHorizontalPadding
}
textVerticalPadding = preferences.readNumber("textVerticalPadding")
if(!textVerticalPadding){
textVerticalPadding = defaultTextVerticalPadding
}
sel = document.windows[0].selection
imajShape = sel.solids[0]
horizontalAlignmentMenu = new Form.Field.Option(
"horizontalTextAlignment",
"Horizontal",
HorizontalTextAlignment.all,
null,
HorizontalTextAlignment.all[textHorizontalIndex]
)
verticalAlignmentMenu = new Form.Field.Option(
"verticalTextAlignment",
"Vertical",
VerticalTextPlacement.all,
null,
VerticalTextPlacement.all[textVerticalIndex]
)
paddings = [0, 1, 2, 3, 4, 5, 6, 8, 10, 12, 14, 18, 20, 24]
textHorizontalPaddingMenu = new Form.Field.Option(
"textHorizontalPadding",
"Padding-H",
paddings,
null,
textHorizontalPadding
)
textVerticalPaddingMenu = new Form.Field.Option(
"textVerticalPadding",
"Padding-V",
paddings,
null,
textVerticalPadding
)
textColorValues = [Color.white, Color.black]
textColorMenu = new Form.Field.Option(
"textColorIndex",
"Color",
[0,1],
["White", "Black"],
textColorIndex
)
inputForm = new Form()
inputForm.addField(horizontalAlignmentMenu)
inputForm.addField(verticalAlignmentMenu)
inputForm.addField(textHorizontalPaddingMenu)
inputForm.addField(textVerticalPaddingMenu)
inputForm.addField(textColorMenu)
formPrompt = "Select text options:"
buttonTitle = "Continue"
formObject = await inputForm.show(formPrompt,buttonTitle)
imajShape.text = imajShape.notes
verticalTextAlignment = formObject.values["verticalTextAlignment"]
imajShape.textVerticalPlacement = verticalTextAlignment
textVerticalIndex = VerticalTextPlacement.all.indexOf(verticalTextAlignment)
preferences.write("textVerticalIndex", textVerticalIndex)
horizontalTextAlignment = formObject.values["horizontalTextAlignment"]
imajShape.textHorizontalAlignment = horizontalTextAlignment
textHorizontalIndex = HorizontalTextAlignment.all.indexOf(horizontalTextAlignment)
preferences.write("textHorizontalIndex", textHorizontalIndex)
textHorizontalPadding = formObject.values["textHorizontalPadding"]
imajShape.textHorizontalPadding = textHorizontalPadding
preferences.write("textHorizontalPadding", textHorizontalPadding)
textVerticalPadding = formObject.values["textVerticalPadding"]
imajShape.textVerticalPadding = textVerticalPadding
preferences.write("textVerticalPadding", textVerticalPadding)
textColorIndex = formObject.values["textColorIndex"]
textColorValue = textColorValues[textColorIndex]
imajShape.textColor = textColorValue
preferences.write("textColorIndex", textColorIndex)
}
catch(err){
if(!err.causedByUserCancelling){
new Alert(err.name, err.message).show()
}
}
});
action.validate = function(selection, sender){
sel = document.windows[0].selection
return (
sel.solids.length === 1 &&
sel.solids[0].image instanceof ImageReference &&
sel.solids[0].notes.length > 0
)
};
return action;
})();