Find/Change Colors
This OmniGraffle plug-in will find and replace all occurrences of a specified HEX color used in any of the selected ways (fill color, stroke color, text color) in all of graphics of the current canvas. If some graphics are already selected, they will be processed, otherwise if no graphics are selected, all graphics on the canvas will be processed.
Return to: OmniGraffle Plug-In Collection
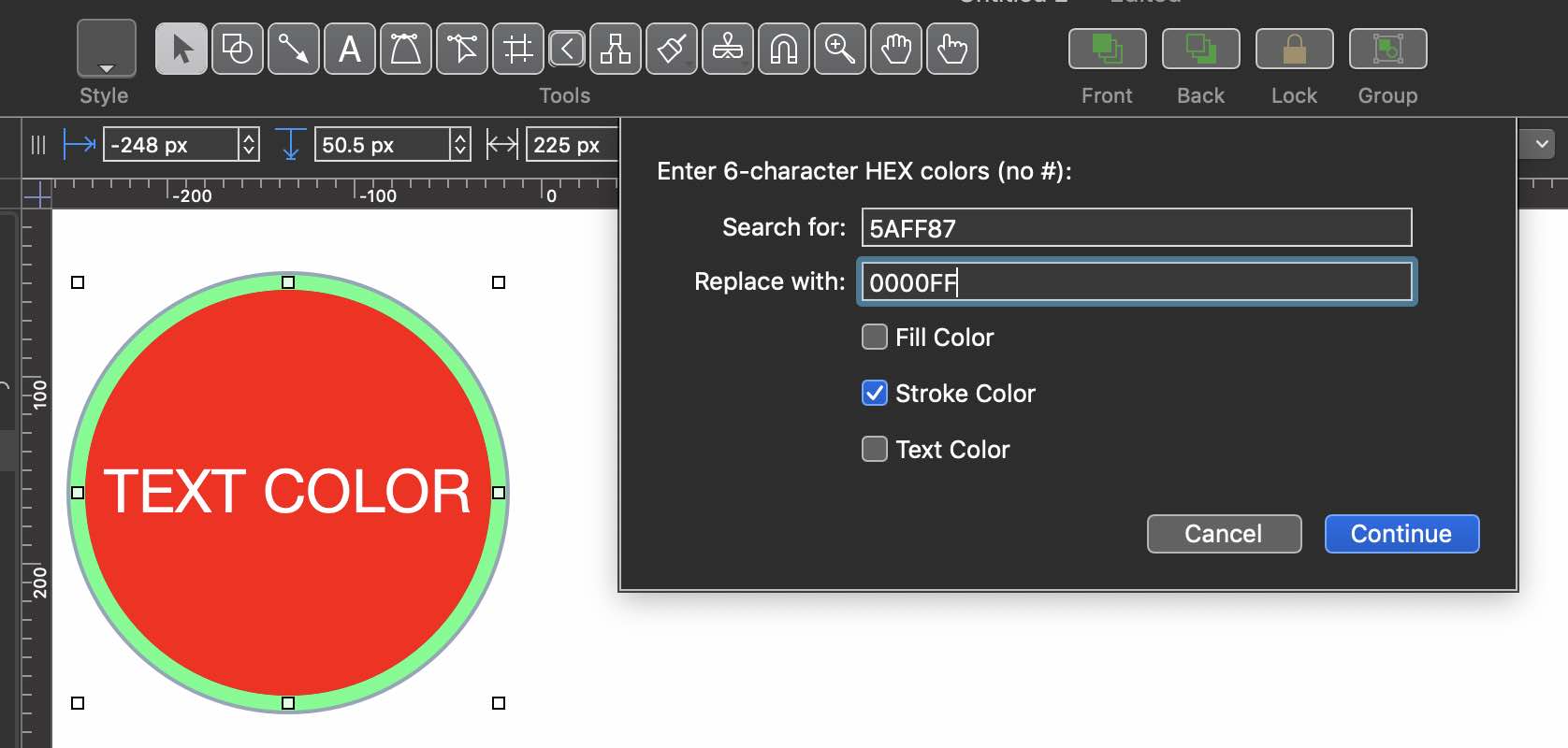
Find/Replace HEX Color
/*{
"type": "action",
"targets": ["omnigraffle"],
"author": "Otto Automator",
"identifier": "com.omni-automation.og.find-change-color",
"version": "1.8",
"description": "Will replace the indicated HEX color value for fill, stroke, and text in either the selected graphics, or if no graphics are selected, all graphics on the current canvas.",
"label": "Find/Change Color",
"shortLabel": "Find/Change Color",
"paletteLabel": "Find/Change Color",
"image":"paintpalette.fill"
}*/
(() => {
var changeCount = 0
function incrementChangeCount(){
changeCount = changeCount + 1
}
function componentToHex(c){
var hex = c.toString(16);
return hex.length == 1 ? "0" + hex : hex;
}
function HEXforColor(clrObj){
rv = clrObj.red
gv = clrObj.green
bv = clrObj.blue
r = Math.round(rv * 255)
g = Math.round(gv * 255)
b = Math.round(bv * 255)
colorHEX = componentToHex(r) + componentToHex(g) + componentToHex(b);
return colorHEX.toUpperCase()
}
function hexToRGBColorObject(hexStr) {
var r = 0, g = 0, b = 0;
r = "0x" + hexStr[0] + hexStr[1];
g = "0x" + hexStr[2] + hexStr[3];
b = "0x" + hexStr[4] + hexStr[5];
red = (+r / 255).toFixed(10)
green = (+g / 255).toFixed(10)
blue = (+b / 255).toFixed(10)
return Color.RGB(Number(red), Number(green), Number(blue), 1)
}
function colorMatch(hexColor, clrObj){
objHEXvalue = HEXforColor(clrObj)
hexValue = objHEXvalue
return (hexColor === hexValue) ? true:false
}
function RGBMatch(colorA, colorB){
return (
colorA.red === colorB.red &&
colorA.green === colorB.green &&
colorA.blue === colorB.blue
) ? true:false
}
function processGroup(aGroup, shouldChangeFill, shouldChangeStroke, shouldChangeText, targetHEX, replacementColor){
processItems(aGroup.graphics, shouldChangeFill, shouldChangeStroke, shouldChangeText, targetHEX, replacementColor)
}
function processItems(items, shouldChangeFill, shouldChangeStroke, shouldChangeText, targetHEX, replacementColor){
items.forEach(item => {
if (item instanceof Shape){
var itemName = (item.name) ? item.name : ""
console.log("Shape", item.id, itemName)
if(shouldChangeText && item.textColor){
if (colorMatch(targetHEX, item.textColor)){
item.textColor = replacementColor
incrementChangeCount()
console.log("Shape", item.id, "text changed")
}
}
if(shouldChangeFill && item.fillColor){
if (colorMatch(targetHEX, item.fillColor)){
item.fillColor = replacementColor
incrementChangeCount()
console.log("Shape", item.id, "fill changed")
}
}
if(shouldChangeStroke && item.strokeColor){
if (colorMatch(targetHEX, item.strokeColor)){
item.strokeColor = replacementColor
incrementChangeCount()
console.log("Shape", item.id, "stroke changed")
}
}
}
else if (item instanceof Line){
var itemName = (item.name) ? item.name : ""
console.log("Line", item.id, itemName)
if(shouldChangeStroke && item.strokeColor){
if (colorMatch(targetHEX, item.strokeColor)){
item.strokeColor = replacementColor
incrementChangeCount()
console.log("Line", item.id, "stroke changed")
}
}
}
else if (item instanceof Group){
var itemName = (item.name) ? item.name : ""
console.log("Group", itemName)
processGroup(item, shouldChangeFill, shouldChangeStroke, shouldChangeText, targetHEX, replacementColor)
}
else {
var itemName = (item.name) ? item.name : ""
console.log("Unknown", item.id, itemName)
}
})
}
const action = new PlugIn.Action(async function(selection, sender){
targetColorField = new Form.Field.String(
"targetColor",
"Search for",
"FFFFFF"
)
replaceColorField = new Form.Field.String(
"replaceColor",
"Replace with",
"000000"
)
fillCheckbox = new Form.Field.Checkbox(
"shouldProcessFill",
"Fill Color",
false
)
strokeCheckbox = new Form.Field.Checkbox(
"shouldProcessStroke",
"Stroke Color",
false
)
textCheckbox = new Form.Field.Checkbox(
"shouldProcessText",
"Text Color",
false
)
inputForm = new Form()
inputForm.addField(targetColorField)
inputForm.addField(replaceColorField)
inputForm.addField(fillCheckbox)
inputForm.addField(strokeCheckbox)
inputForm.addField(textCheckbox)
inputForm.validate = function(formObject){
processFill = formObject.values['shouldProcessFill']
processStroke = formObject.values['shouldProcessStroke']
processText = formObject.values['shouldProcessText']
values = [processFill, processStroke, processText]
var targetStatus, replaceStatus
targetColor = formObject.values['targetColor']
if(!targetColor){return false}
if (/^[a-f0-9]+$/i.test(targetColor)){
targetStatus = (targetColor.length === 6) ? true:false
} else {
targetStatus = false
}
replaceColor = formObject.values['replaceColor']
if (!replaceColor){return false}
if (/^[a-f0-9]+$/i.test(replaceColor)){
replaceStatus = (replaceColor.length === 6) ? true:false
} else {
replaceStatus = false
}
return (values.includes(true) && targetStatus === true && replaceStatus === true) ? true:false
}
formPrompt = "Enter 6-character HEX colors (no #):"
buttonTitle = "Continue"
formObject = await inputForm.show(formPrompt,buttonTitle)
console.clear()
shouldChangeFill = formObject.values['shouldProcessFill']
shouldChangeStroke = formObject.values['shouldProcessStroke']
shouldChangeText = formObject.values['shouldProcessText']
targetHEX = formObject.values['targetColor']
console.log("targetHEX",targetHEX)
targetColor = hexToRGBColorObject(targetHEX)
replaceHEX = formObject.values['replaceColor']
console.log("replaceHEX",replaceHEX)
replacementColor = hexToRGBColorObject(replaceHEX)
console.log("fill:" + shouldChangeFill,"stroke:" + shouldChangeStroke,"text:" + shouldChangeText)
// use selected graphics or if none, all graphics
targetGraphics = selection.graphics
if (targetGraphics.length === 0){
cnvs = selection.canvas
targetGraphics = cnvs.graphics
}
changeCount = 0
processItems(targetGraphics, shouldChangeFill, shouldChangeStroke, shouldChangeText, targetHEX, replacementColor)
console.log("// Script to locate an item by ID:")
console.log("cnvs = document.windows[0].selection.canvas")
console.log("graphic = cnvs.graphicWithId(*idNumber*)")
console.log("document.windows[0].selection.view.select([graphic],false)")
message = String(changeCount) + " items were altered."
new Alert("COMPLETED",message).show()
});
action.validate = function(selection, sender){
return true
};
return action;
})();