Repeating Tasks
Omni Automation in OmniFocus provides the ability to set the repetition rules for a task. The repetitionRule property of the Task class is used to access and set any repeating parameters.
repetitionRule (Task.RepetitionRule or null) • If repetition is enabled for the task, the value of this task property is an instance of the Task.RepetitionRule class.
The Task.RepetitionRule Class
A Task.RepetitionRule describes a pattern of dates using a ICS formatted recurrence string (iCalendar.org) and a Task.RepetitionMethod to describe how those dates are applied to a Task.
The properties of the Task.RepetitionRule class:
method (Task.RepetitionMethod r/o) • The method used to create the repetition rule.
ruleString (String r/o) • The ICS rule string used to create the repetition rule.
The instance functions of the Task.RepetitionRule class:
firstDateAfterDate(date: Date) → (Date) • Returns the first date described by the repetition rule that is after the given date.
Date/Time of the Next Task Repetition
var task = document.windows[0].selection.tasks[0]
if(task && task.repetitionRule){
console.log(task.name, task.repetitionRule.firstDateAfterDate(new Date()))
}
The Task.RepetitionMethod Class
DeferUntilDate (Task.RepetitionMethod r/o) • XXXXX
DueDate (Task.RepetitionMethod r/o) • XXXXX
Fixed (Task.RepetitionMethod r/o) • Task.RepetitionMethod r/o) • XXXXX
None (Task.RepetitionMethod r/o) • The task does not repeat.
all (Array of Task.RepetitionMethod r/o) • An array of all items of this enumeration. Often used when creating action forms.
New Repeating Task
var task = new Task("My Weekly Task")
var ruleString = "FREQ=WEEKLY"
var repetitionMethod = Task.RepetitionMethod.DueDate
task.repetitionRule = new Task.RepetitionRule(ruleString, repetitionMethod)
var taskID = task.id.primaryKey
URL.fromString("omnifocus:///task/" + taskID).open()
And to disable repetition, set the property value to null:
Stop Repetition
var task = taskNamed("My Weekly Task")
task.repetitionRule = null
The Rule Info Plug-In
Aside from using the free RRULE Tool at iCalendar.org, perhaps the simplest way to derive an ICS rule string is to set up an example repeating task in OmniFocus using the standard application interface, and then use Omni Automation to retrieve the corresponding ISC rule string from the selected task. You can then use the extracted ICS rule string in your scripts to define the specified repetition parameters.
The plug-in provided below will both display the ICS string and log it to the console for you.
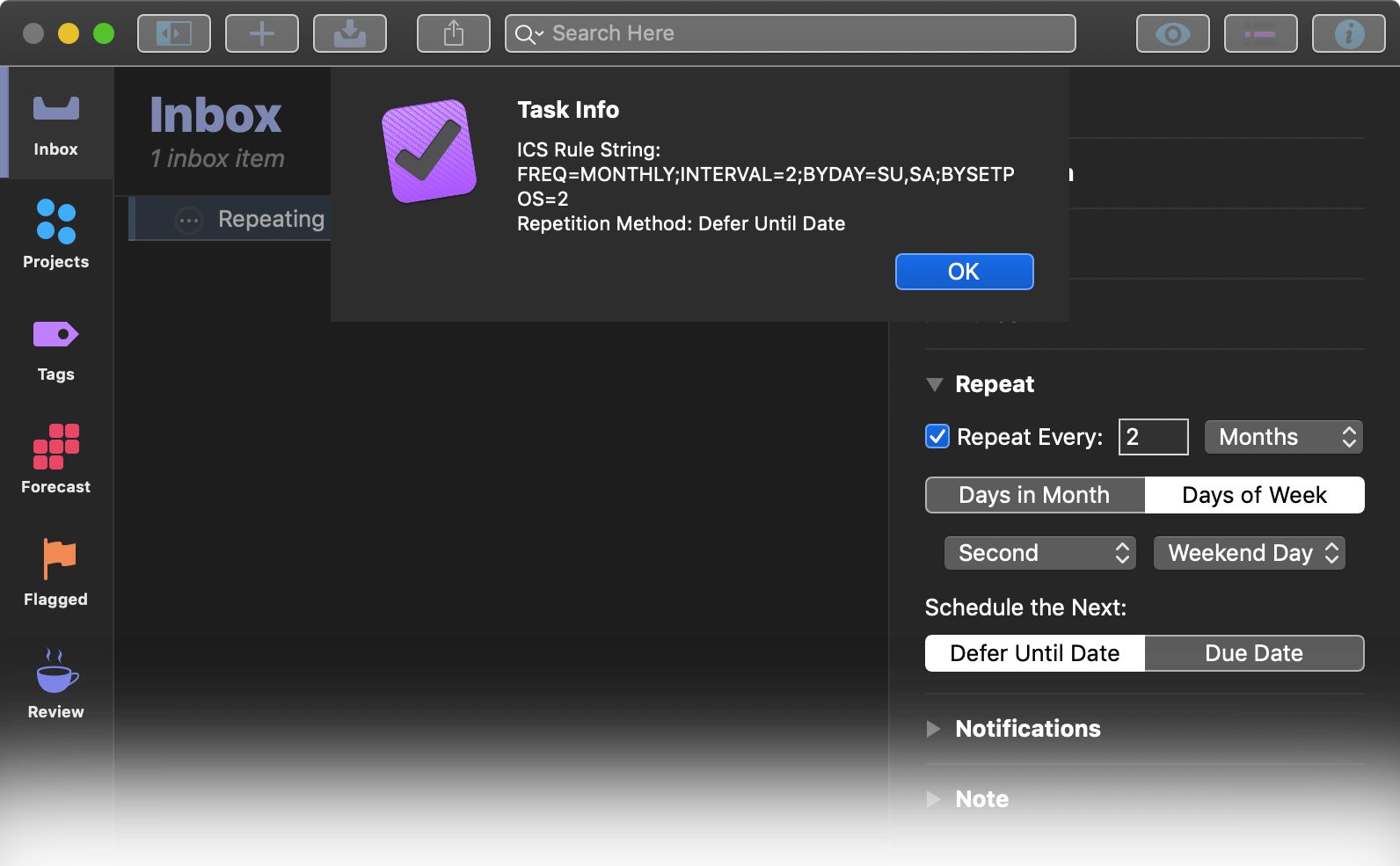
Rule Info for Selected Task
/*{
"type": "action",
"targets": ["omnifocus"],
"author": "Otto Automator",
"identifier": "com.omni-automation.of.task-rule-info",
"version": "1.1",
"description": "This action will display an alert showing the ICS rule string and repetition method of the selected task or project.",
"label": "Rule Info for Selected Item",
"shortLabel": "Rule Info",
"paletteLabel": "Rule Info",
"image": "info.square.fill"
}*/
(() => {
const action = new PlugIn.Action(async function(selection, sender){
if (selection.projects.length === 1){
var item = selection.projects[0]
var alertTitle = "Project: " + item.name
} else {
var item = selection.tasks[0]
var alertTitle = "Task: " + item.name
}
rule = item.repetitionRule
ruleString = item.repetitionRule.ruleString
repetitionMethod = item.repetitionRule.method
msg = ""
var methodName
switch(repetitionMethod) {
case Task.RepetitionMethod.DueDate:
methodName = "Due Date"
break;
case Task.RepetitionMethod.Fixed:
methodName = "Fixed"
break;
case Task.RepetitionMethod.DeferUntilDate:
methodName = "Defer Until Date"
break;
default:
methodName = "None"
}
msg = "ICS Rule String: " + ruleString + "\n" + "Repetition Method: " + methodName
console.log(msg)
new Alert(alertTitle, msg).show()
});
action.validate = function(selection, sender){
return (
selection.projects.length === 1 &&
selection.projects[0].repetitionRule != null ||
selection.tasks.length === 1 &&
selection.tasks[0].repetitionRule != null
)
};
return action;
})();
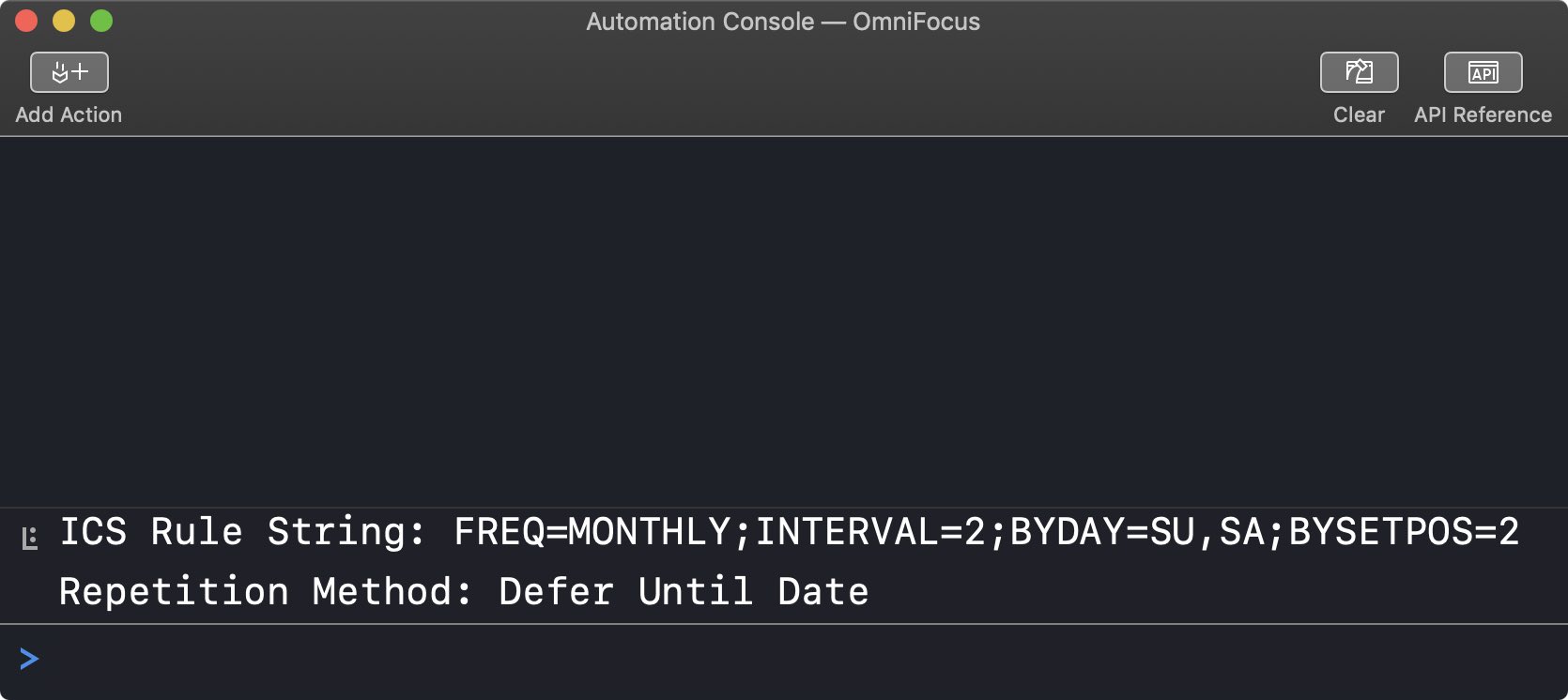
Repeating Project with Sequential Tasks
This example demonstrates repetition through the tasks required to create and present a kindergarten class every week. Each task has a morning notification and evening due date. Once the project’s sequential tasks are completed, the project is marked completed and a new blank project is generated as its replacement.
Kinder Class
formatter = Formatter.Date.withStyle(Formatter.Date.Style.Short)
folder = folderNamed("Personal") || new Folder("Personal")
projectName = "Kinder Class"
project = folder.projectNamed(projectName)
if(project){deleteObject(project)}
project = Object.assign(
new Project(projectName, folder),
{
sequential : true,
note : "My weekly kindergarten class"
}
)
tag = flattenedTags.byName("Instruction") || new Tag("Instruction")
project.addTag(tag)
ruleString = "FREQ=WEEKLY"
repetitionMethod = Task.RepetitionMethod.DueDate
project.repetitionRule = new Task.RepetitionRule(ruleString, repetitionMethod)
project.completedByChildren = true
task = Object.assign(
new Task("(Wed) Select Topic for Kinder Class", project),
{
note : "Determine a topic for this week’s class",
dueDate: formatter.dateFromString('wednesday @ 7:00PM')
}
)
dateObj = formatter.dateFromString('wednesday @ 9:00AM')
task.addNotification(dateObj)
task.clearTags()
task = Object.assign(
new Task("(Thu) Create Materials for Kinder Class", project),
{
note : "Create materials for this week’s class",
dueDate: formatter.dateFromString('thursday @ 7:00PM')
}
)
dateObj = formatter.dateFromString('thursday @ 9:00AM')
task.addNotification(dateObj)
task.clearTags()
task = Object.assign(
new Task("(Sat) Kinder Class Rehearsal", project),
{
note : "Rehearse Kinder Class presentation",
dueDate: formatter.dateFromString('saturday @ 7:00PM')
}
)
dateObj = formatter.dateFromString('saturday @ 9:00AM')
task.addNotification(dateObj)
task.clearTags()
task = Object.assign(
new Task("(Sun) Kinder Class", project),
{
note : "Kinder Class",
dueDate : formatter.dateFromString('sunday @ 11:30AM')
}
)
task.addNotification(-3600)
dateObj = formatter.dateFromString('sunday @ 9:00AM')
task.addNotification(dateObj)
task.clearTags()
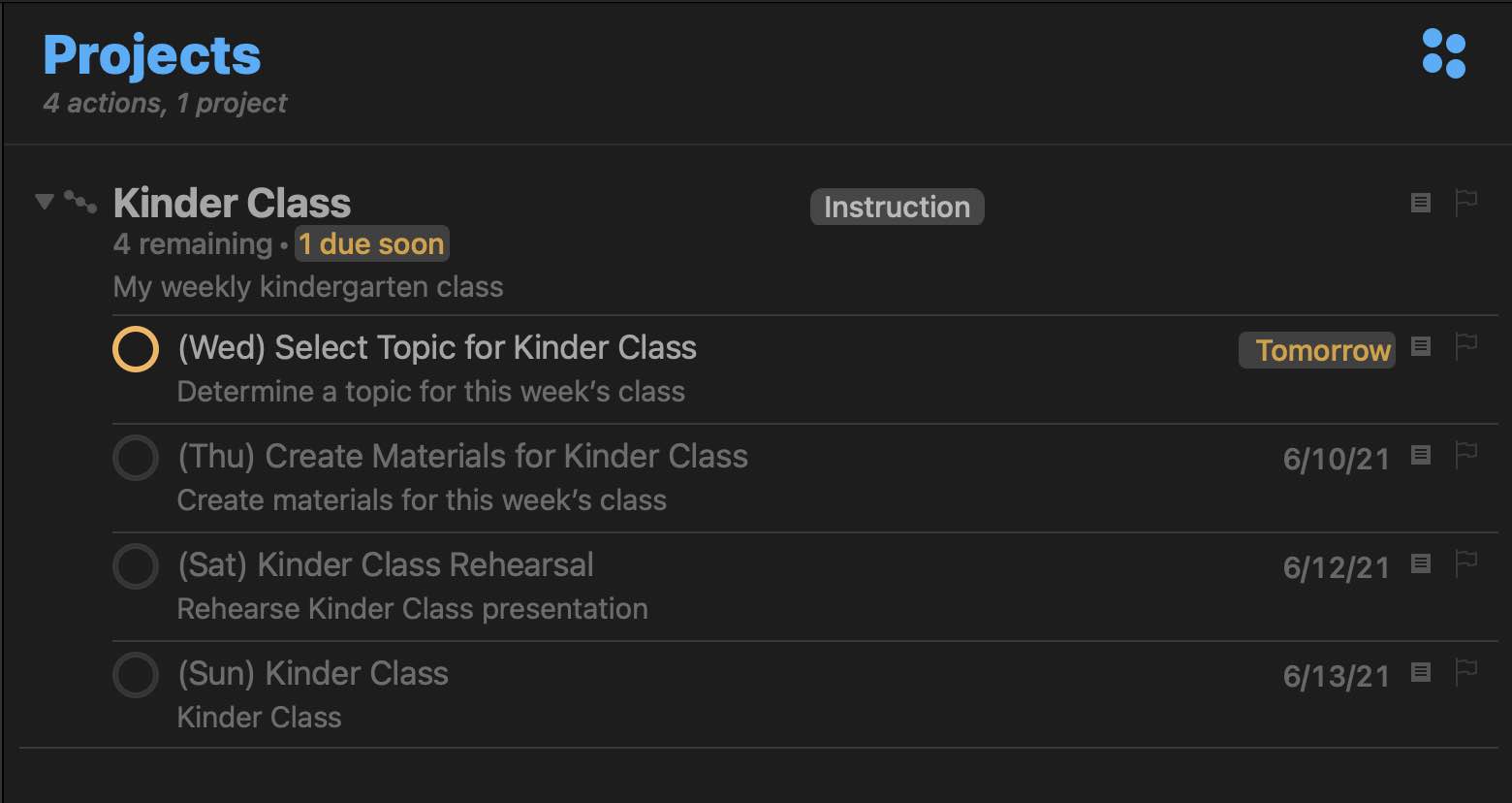