Plug-In: Quick Appointment
This plug-in will prompt for the information required to create an appointment task.
Updates:
v1.1 · Day options now include “today” and “tomorrow.” Time options expanded to 8:00AM - 5:45PM
v1.2 · Plug-In now uses asynchronous code
Return to: OmniFocus Plug-In Collection
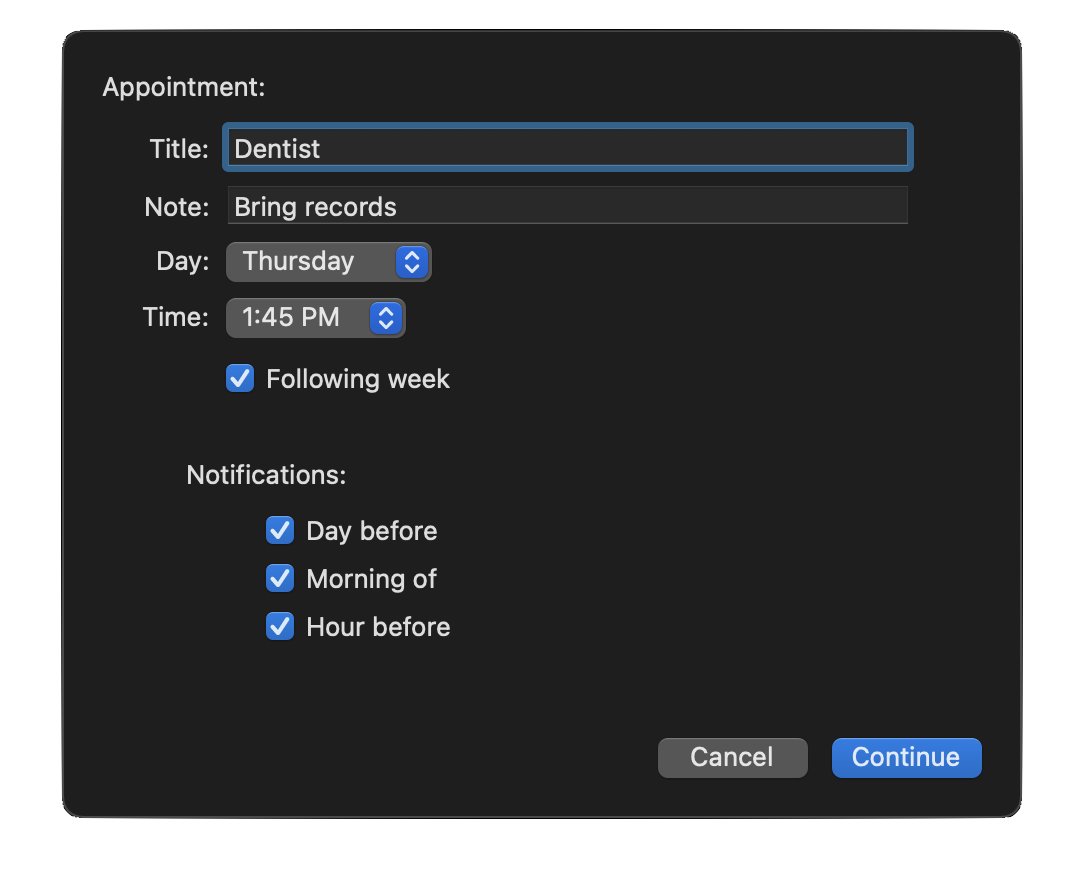
Quick Appointment
/*{
"type": "action",
"targets": ["omnifocus"],
"author": "Otto Automator",
"identifier": "com.omni-automation.of.quick-appointment",
"version": "1.2",
"description": "Presents an interface for creating a new appointment task.",
"label": "Quick Appointment",
"shortLabel": "Appointment",
"paletteLabel": "Appointment",
"image": "calendar.badge.plus"
}*/
(() => {
const action = new PlugIn.Action(async function(selection, sender){
appointmentNameField = new Form.Field.String(
"appointmentName",
"Title",
null,
null
)
appointmentNoteField = new Form.Field.String(
"appointmentNote",
"Note",
null,
null
)
menu1Items = ["Today", "Tomorrow", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday", "Sunday"]
var menu1Indexes = new Array()
menu1Items.forEach((item, index) => menu1Indexes.push(index))
menu2Items = ["8:00 AM", "8:15 AM", "8:30 AM", "8:45 AM", "9:00 AM", "9:15 AM", "9:30 AM", "9:45 AM", "10:00 AM", "10:15 AM", "10:30 AM", "10:45 AM", "11:00 AM", "11:15 AM", "11:30 AM", "11:45 AM", "12:00 PM", "12:15 PM", "12:30 PM", "12:45 PM", "1:00 PM", "1:15 PM", "1:30 PM", "1:45 PM", "2:00 PM", "2:15 PM", "2:30 PM", "2:45 PM", "3:00 PM", "3:15 PM", "3:30 PM", "3:45 PM", "4:00 PM", "4:15 PM", "4:30 PM", "4:45 PM", "5:00 PM", "5:15 PM", "5:30 PM", "5:45 PM"]
menu2Indexes = new Array()
menu2Items.forEach((item, index) => menu2Indexes.push(index))
menu1Element = new Form.Field.Option(
"menu1Element",
"Day",
menu1Indexes,
menu1Items,
0
)
menu2Element = new Form.Field.Option(
"menu2Element",
"Time",
menu2Indexes,
menu2Items,
0
)
checkSwitchField = new Form.Field.Checkbox(
"followingWeek",
"Following week",
null
)
menuLabel = "Notifications"
notificationOptionsStrings = ["Day before", "Morning of", "Hour before"]
multiOptionMenu = new Form.Field.MultipleOptions(
"notificationsOptions",
menuLabel,
[0,1,2],
notificationOptionsStrings,
[0,1,2]
)
inputForm = new Form()
inputForm.addField(appointmentNameField)
inputForm.addField(appointmentNoteField)
inputForm.addField(menu1Element)
inputForm.addField(menu2Element)
inputForm.addField(checkSwitchField)
inputForm.addField(multiOptionMenu)
inputForm.validate = function(formObject){
var appointmentName = formObject.values['appointmentName']
if (!appointmentName || appointmentName.length === 0){return false}
return true
}
formPrompt = "Appointment:"
buttonTitle = "Continue"
formObject = await inputForm.show(formPrompt, buttonTitle)
menu1Index = formObject.values['menu1Element']
chosen1Item = menu1Items[menu1Index]
menu2Index = formObject.values['menu2Element']
chosen2Item = menu2Items[menu2Index]
chosen2Item = chosen2Item.replace(' ', '')
useFollowingWeek = formObject.values['followingWeek']
fmatr = Formatter.Date.withStyle(Formatter.Date.Style.Full, Formatter.Date.Style.Short)
dateObj = fmatr.dateFromString(`${chosen1Item} @ ${chosen2Item}`)
if(useFollowingWeek){
dc = Calendar.current.dateComponentsFromDate(dateObj)
dc.day = dc.day + 7
dateObj = Calendar.current.dateFromDateComponents(dc)
}
dateStr = fmatr.stringFromDate(dateObj)
appointmentName = formObject.values['appointmentName']
appointmentNote = formObject.values['appointmentNote']
if (!appointmentNote){appointmentNote = ""}
task = new Task(appointmentName)
task.note = appointmentNote
task.dueDate = dateObj
tag = flattenedTags.byName("Appointment") || new Tag("Appointment")
task.addTag(tag)
notificationIndexes = formObject.values["notificationsOptions"]
notificationIndexes.forEach(index => {
// day before, morning of, hour before
if(index === 0){task.addNotification(-86400)}
if(index === 1){
var dc = Calendar.current.dateComponentsFromDate(dateObj)
dc.hour = 9 // morning hour
dc.minute = 0 // morning minute
var notificationDateObj = Calendar.current.dateFromDateComponents(dc)
task.addNotification(notificationDateObj)
}
if(index === 2){task.addNotification(-3600)}
})
// CONFIRMATION ALERT
alertTitle = "Form Result"
alertMessage = "Your appointment is for:\n\n" + dateStr
alert = new Alert(alertTitle, alertMessage)
alert.addOption("Show")
alert.addOption("OK")
buttonIndex = await alert.show()
if (buttonIndex === 0){
var taskID = task.id.primaryKey
var urlStr = "omnifocus://task/" + taskID
URL.fromString(urlStr).open()
}
});
action.validate = function(selection, sender){
return true
};
return action;
})();