Plug-In: Project with Tasks
An example of using a plug-in as a project template. This plug-in will prompt for titles and status of the project and any tasks (up to five), and use that to construct a new parent project placed at the top of the Projects list.
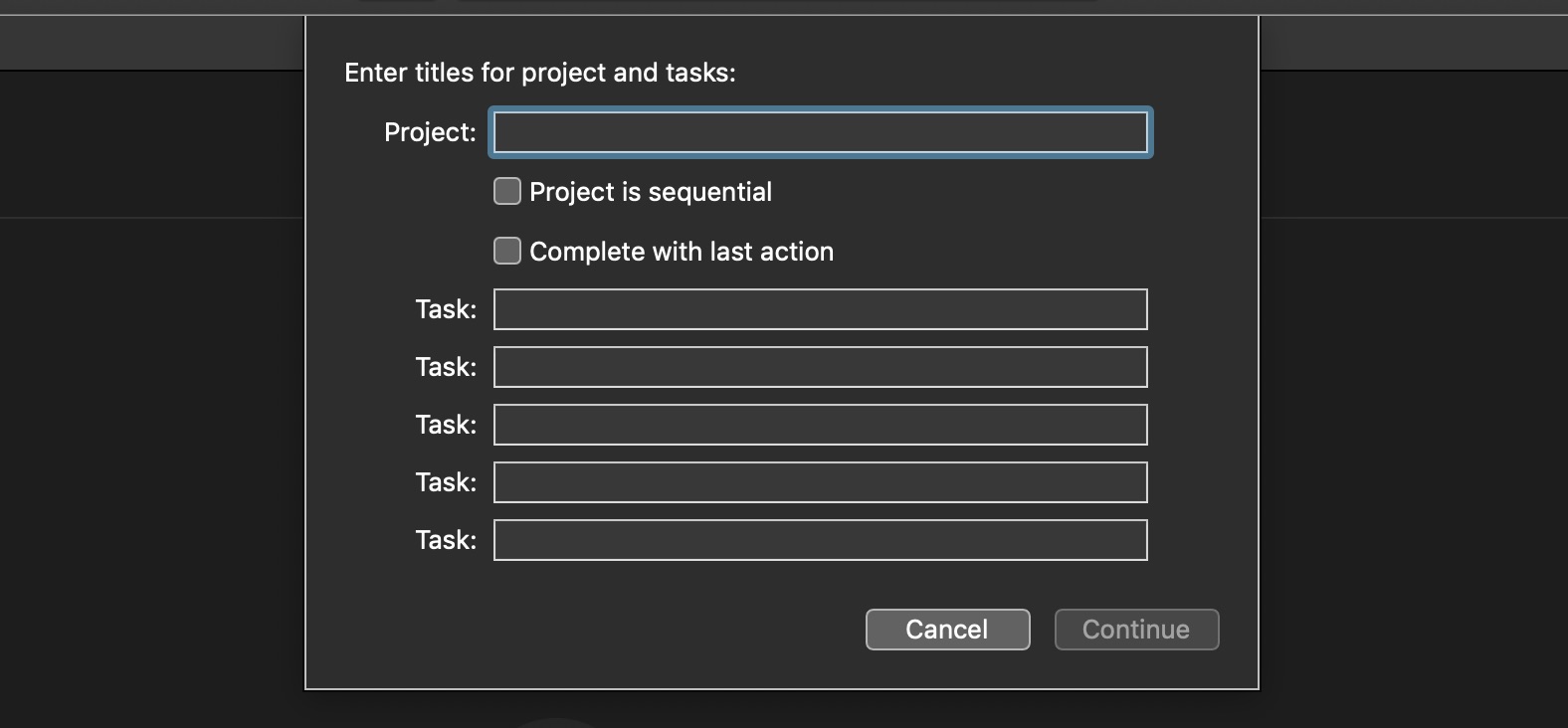
Return to: OmniFocus Plug-In Collection
New Project with Tasks
/*{
"type": "action",
"targets": ["omnifocus"],
"author": "Otto Automator",
"identifier": "com.omni-automation.of.project-with-tasks",
"version": "1.1",
"description": "This plug-in creates a new project containing the specified tasks.",
"label": "New Project with Tasks",
"shortLabel": "Project with Tasks",
"paletteLabel": "Project with Tasks",
"image": "list.bullet.rectangle"
}*/
(() => {
const action = new PlugIn.Action(async function(selection, sender){
try {
textInputField01 = new Form.Field.String(
"main-project",
"Project",
null
)
textInputField02 = new Form.Field.String(
"sub-task-1",
"Task",
null
)
textInputField03 = new Form.Field.String(
"sub-task-2",
"Task",
null
)
textInputField04 = new Form.Field.String(
"sub-task-3",
"Task",
null
)
textInputField05 = new Form.Field.String(
"sub-task-4",
"Task",
null
)
textInputField06 = new Form.Field.String(
"sub-task-5",
"Task",
null
)
shouldBeSequentialCheckbox = new Form.Field.Checkbox(
"shouldBeSequential",
"Project is sequential",
false
)
shouldBeCompletedByChildrenCheckbox = new Form.Field.Checkbox(
"shouldBeCompletedByChildren",
"Complete with last action",
false
)
inputForm = new Form()
inputForm.addField(textInputField01)
inputForm.addField(shouldBeSequentialCheckbox)
inputForm.addField(shouldBeCompletedByChildrenCheckbox)
inputForm.addField(textInputField02)
inputForm.addField(textInputField03)
inputForm.addField(textInputField04)
inputForm.addField(textInputField05)
inputForm.addField(textInputField06)
inputForm.validate = function(formObject){
var inputText01 = formObject.values['main-project']
var inputText01Status = (!inputText01)?false:true
var validation = (inputText01Status) ? true:false
return validation
}
formPrompt = "Enter titles for project and tasks:"
buttonTitle = "Continue"
formObject = await inputForm.show(formPrompt,buttonTitle)
mainProjectTitle = formObject.values['main-project']
subTask1Title = formObject.values['sub-task-1']
subTask2Title = formObject.values['sub-task-2']
subTask3Title = formObject.values['sub-task-3']
subTask4Title = formObject.values['sub-task-4']
subTask5Title = formObject.values['sub-task-5']
shouldBeSequential = formObject.values['shouldBeSequential']
shouldBeCompletedByChildren = formObject.values['shouldBeCompletedByChildren']
project = new Project(mainProjectTitle,library.beginning)
projectID = project.id.primaryKey
if(shouldBeSequential === true){project.sequential = true}
if(shouldBeCompletedByChildren === true){project.completedByChildren = true}
if(subTask1Title){
new Task(subTask1Title, project)
console.log(subTask1Title)
}
if(subTask2Title){
new Task(subTask2Title, project)
console.log(subTask2Title)
}
if(subTask3Title){
new Task(subTask3Title, project)
console.log(subTask3Title)
}
if(subTask4Title){
new Task(subTask4Title, project)
console.log(subTask4Title)
}
if(subTask5Title){
new Task(subTask5Title, project)
console.log(subTask5Title)
}
URL.fromString(`omnifocus:///task/${projectID}`).open()
}
catch(err){
console.error(err)
}
});
action.validate = function(selection, sender){
return true
};
return action;
})();