Plug-In: Multi-Day Project ![Plug-in is compatible with Apple Vision Pro Apple Vision Pro]()
This plug-in creates a project, lasting a specified number of days, containing a sequential series of daily tasks.
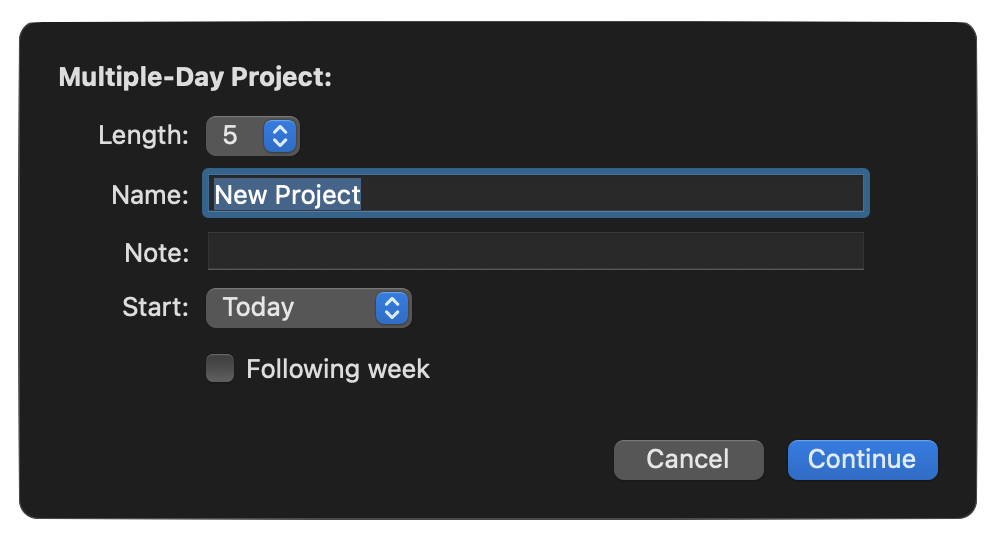
Return to: OmniFocus Plug-In Collection
Mutli-Day Project
/*{
"type": "action",
"targets": ["omnifocus"],
"author": "Otto Automator",
"identifier": "com.omni-automation.of.multiple-day-project",
"version": "1.1",
"description": "Creates a sequential project with tasks for each of the indicated days.",
"label": "Multi-Day Project",
"shortLabel": "Multi-Day Project",
"paletteLabel": "Multi-Day Project",
"image": "archivebox.fill"
}*/
(() => {
const action = new PlugIn.Action(async function(selection, sender){
lengths = [2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14]
lengthStrings = ["2", "3", "4", "5", "6", "7", "8", "9", "10", "11", "12", "13", "14"]
projectLengthField = new Form.Field.Option(
"projectLength",
"Length",
lengths,
lengthStrings,
3
)
projectNameField = new Form.Field.String(
"projectName",
"Name",
"New Project",
null
)
appointmentNoteField = new Form.Field.String(
"projectNote",
"Note",
null,
null
)
menu1Items = ["Today", "Tomorrow", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday", "Sunday"]
menu1Indexes = menu1Items.map((item, index) => index)
menu1Element = new Form.Field.Option(
"menu1Element",
"Start",
menu1Indexes,
menu1Items,
0
)
checkSwitchField = new Form.Field.Checkbox(
"followingWeek",
"Following week",
null
)
inputForm = new Form()
inputForm.addField(projectLengthField)
inputForm.addField(projectNameField)
inputForm.addField(appointmentNoteField)
inputForm.addField(menu1Element)
inputForm.addField(checkSwitchField)
inputForm.validate = function(formObject){
projectName = formObject.values['projectName']
if (!projectName || projectName.length === 0){return false}
return true
}
defaultDueTime = settings.objectForKey('DefaultDueTime')
formPrompt = "Multiple-Day Project:"
buttonTitle = "Continue"
formObject = await inputForm.show(formPrompt, buttonTitle)
menu1Index = formObject.values['menu1Element']
chosen1Item = menu1Items[menu1Index]
projectName = formObject.values['projectName']
useFollowingWeek = formObject.values['followingWeek']
projectLength = formObject.values['projectLength']
projectNote = formObject.values['projectNote']
fmatr = Formatter.Date.withStyle(Formatter.Date.Style.Full, Formatter.Date.Style.Short)
dateObj = fmatr.dateFromString(`${chosen1Item} @ ${defaultDueTime}`)
if(useFollowingWeek){
dc = Calendar.current.dateComponentsFromDate(dateObj)
dc.day = dc.day + 7
dateObj = Calendar.current.dateFromDateComponents(dc)
}
currentLocale = Calendar.current.locale.identifier
currentLocale = currentLocale.replace("_", "-");
project = new Project(projectName)
project.sequential = true
project.completedByChildren = true
if(projectNote){project.note = projectNote}
for (var i = 0; i < projectLength; i++) {
var weekday = dateObj.toLocaleString(currentLocale, {weekday: 'short'});
var taskName = `Day ${i + 1} · ${weekday} ${dateObj.toLocaleDateString()}`
new Task(taskName, project).dueDate = dateObj
var dateObj = new Date(dateObj.setDate(dateObj.getDate() + 1))
}
projectID = project.id.primaryKey
URL.fromString("omnifocus:///task/" + projectID).open()
});
action.validate = function(selection, sender){
return true
};
return action;
})();