The Application Object
In Omni Automation, the OmniFocus application object has properties (name, version) and elements (documents, windows, database). In a script, the application object is represented as: app
If you entered just “app” in the OmniFocus console window and executed the line, the result would be a reference to the application object:
The Application Object
app
//--> [object Application]
Properties: name
The value of the name property of the application object is a string representing the name of the OmniFocus application.
Application Name Property
app.name
//--> OmniFocus
The value of the application name property is read-only.
Properties: platformName
To get the name of the OS the app is running on:
The Platform Property
app.platformName
//--> iOS (iPadOS), macOS
Properties: Key Down Properties (macOS)
On macOS, the following application properties can be used by scripts to determine if the standard modifier keys are pressed when the script is run:
commandKeyDown (Boolean r/o) • Is the Command key pressed?
controlKeyDown (Boolean r/o) • Is the Control key pressed?
optionKeyDown (Boolean r/o) • Is the Option key pressed?
shiftKeyDown (Boolean r/o) • Is the Shift key pressed?
Modifier Key Sensor
if(app.platformName === "macOS"){
var keys = new Array()
if (app.commandKeyDown){keys.push("Command Key " + '\u2318')}
if (app.optionKeyDown){keys.push("Option Key " + '\u2325')}
if (app.shiftKeyDown){keys.push("Shift Key " + '\u21E7')}
if (app.controlKeyDown){keys.push("Control Key " + '\u2303')}
if (keys.length === 0){keys = ["No modifier keys were pressed."]}
alertStr = keys.join("\n")
var alert = new Alert('\uF8FF' + ' Modifier Keys',alertStr)
alert.show()
} else {console.error('macOS only')}
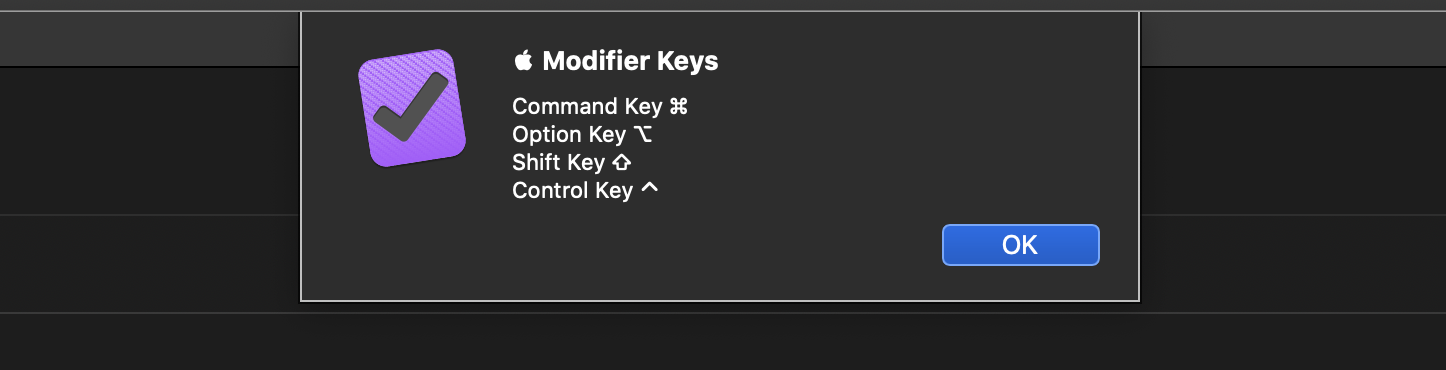
See the section on PlugIns and Actions for examples of the use of modifier keys.
Properties: version
The value of the version property of the application object is a string representing the version number of the OmniFocus application.
Version 3.11.2 or greater of OmniFocus now includes two version properties that replace the previous version property.
The value of the application version properties are read-only.
version (String r/o) • Deprecated: Recommend using either userVersion or buildVersion. For backwards compatibility with existing scripts, this returns the same result as buildVersion.versionString. We recommend using either the user-visible userVersion or the internal buildVersion instead, which are more clear about which version they’re returning and provide their results as Version objects which can be semantically compared with other Version objects.
userVersion (Version r/o) • The user-visible version number for the app. See also buildVersion.
buildVersion (Version r/o) • The internal build version number for the app. See also userVersion.
App Version Property (deprecated)
app.version
//--> "149.6.7"
App User Version Property
app.userVersion
//--> [object Version: 3.11.2]
app.userVersion.versionString
//--> "3.11.2"
App Build Version Property
app.buildVersion
//--> [object Version: 149.6.7]
app.buildVersion.versionString
//--> "149.6.7"
Version Objects
To create a new version object:
Create Version Object
new Version('201.33')
//--> [object Version]
To extract the version string from a version object:
Get Version String
var vers = new Version('201.33')
vers.versionString
//--> 201.33
Comparing Versions
There are four methods you can use for comparing version objects:
equals(versToCheck)
isBefore(versToCheck)
isAfter(versToCheck)
atLeast(versToCheck)
Here are functions demonstrating their use:
Version Comparison Functions
function isBeforeCurVers(versStrToCheck){
curVers = app.userVersion
curVersStr = curVers.versionString
versToCheck = new Version(versStrToCheck)
result = versToCheck.isBefore(curVers)
console.log(versStrToCheck + ' is before ' + curVersStr + " = " + result)
return result
}
function isEqualToCurVers(versStrToCheck){
curVers = app.userVersion
curVersStr = curVers.versionString
versToCheck = new Version(versStrToCheck)
result = versToCheck.equals(curVers)
console.log(versStrToCheck + ' equals ' + curVersStr + " = " + result)
return result
}
function isAtLeastCurVers(versStrToCheck){
curVers = app.userVersion
curVersStr = curVers.versionString
versToCheck = new Version(versStrToCheck)
result = versToCheck.atLeast(curVers)
console.log(versStrToCheck + ' is at least ' + curVersStr + " = " + result)
return result
}
function isAfterCurVers(versStrToCheck){
curVers = app.userVersion
curVersStr = curVers.versionString
versToCheck = new Version(versStrToCheck)
result = versToCheck.isAfter(curVers)
console.log(versStrToCheck + ' is after ' + curVersStr + " = " + result)
return result
}